In my [previous post](https://leofinance.io/hive-167922/@chasmic-cosm/automated-trading-with-the-macd-in-python) I introduced a rudimentary trading strategy based on the [MACD](https://leofinance.io/hive-167922/@chasmic-cosm/calculating-the-macd-in-python). This strategy produced lots of spurious trading signals and would probably not be particularly effective in the wild. In this post I'll explore some attempts to improve the quality of signal produced by the MACD strategy. # Baseline results In order to evaluate the performance of each approach, we need to have a reference. The naïve MACD strategy produces lots of bad trade signals over the test data. 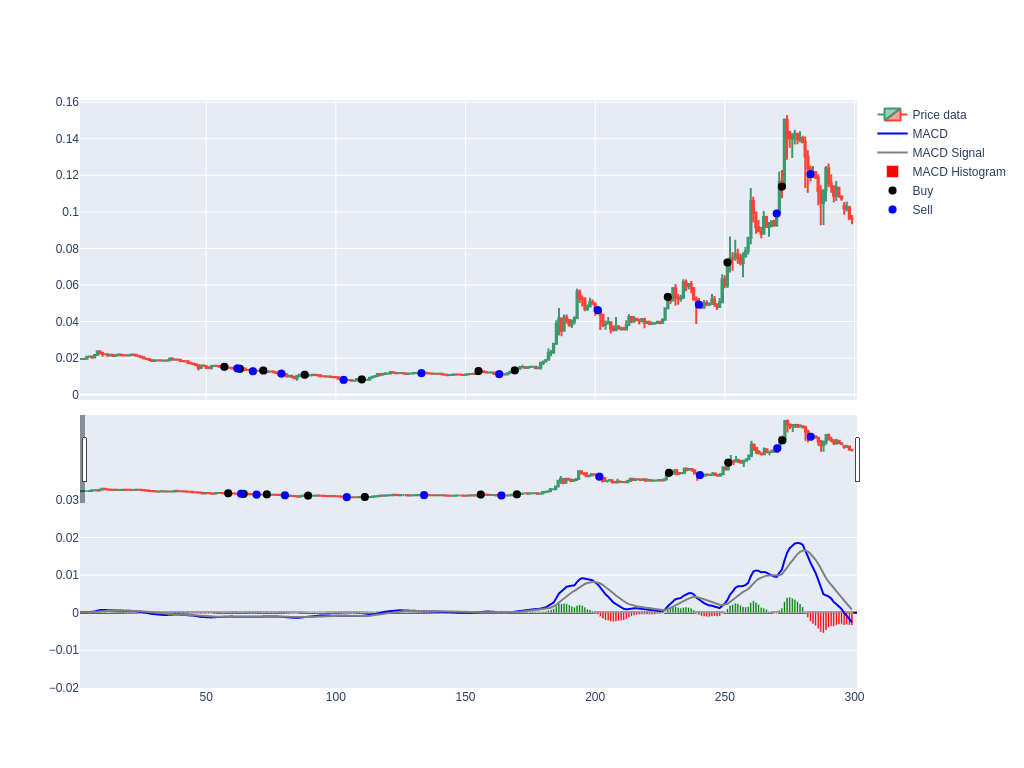 # Approach 1: Positivity of the MACD We can try to filter out bad trade signals by insisting that the MACD be positive in order to trigger a trade. ```python import numpy as np def macd_strategy2(ohlcv): """ Calculate the macd of a stock, decide whether to buy, sell, or hold based on signal behaviour Inputs: ohlcv: numpy array - A 5 x N matrix with rows corresponding to o, h, l, c, v respectively Outputs: status: string - "BUY", "SELL", or "HOLD" """ typical = typical_price(ohlcv) macd, macd_sig, macd_hist = macd(typical) macd_hist = macd_hist[::-1] # reversed MACD histogram macd = macd[::-1] if macd_hist[0] > 0 and macd_hist[1] <= 0 and macd[0] >= 0: return "BUY" elif macd_hist[0] < 0 and macd_hist[1] >= 0: return "SELL" else: return "HOLD" ``` This seems to filter out quite a few of the bad buy signals, however this strategy isn't applicable for sell signals. 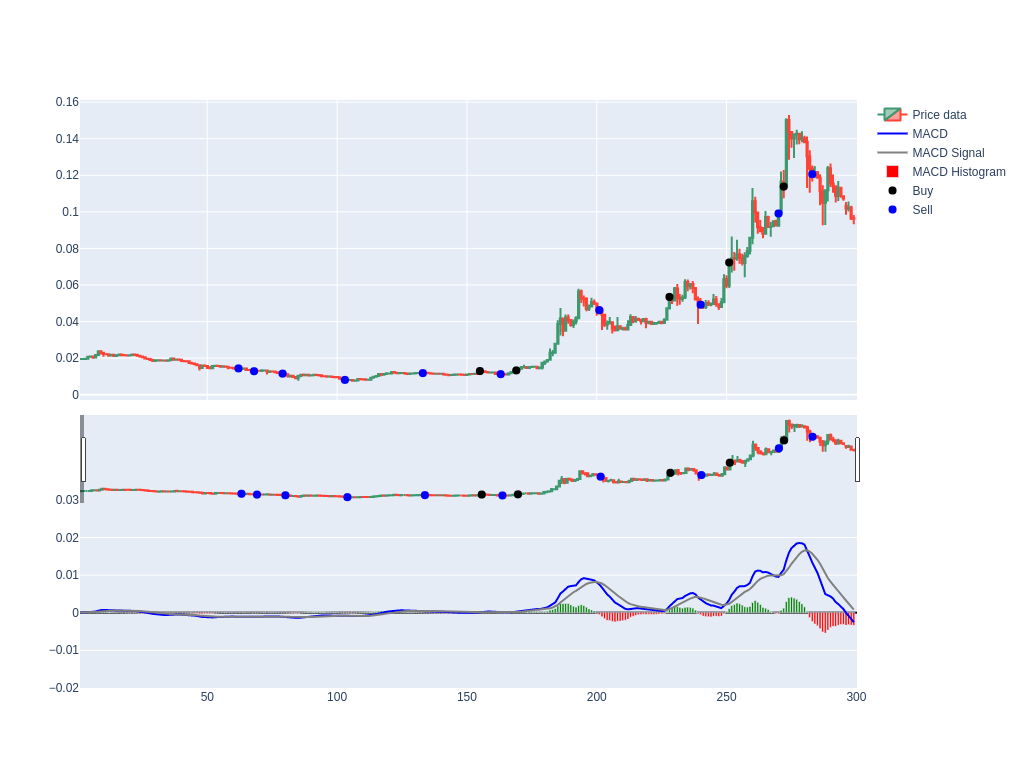 # Approach 2: Positivity of the derivative Another potential way to filter out signals from the MACD is to look at the sign of the first derivative. In order to calculate the first derivative, we can use a rudimentary method such as a finite difference scheme: ```python import numpy as np def first_deriv(signal, dt): """ Calculate the first derivative using finite difference method Inputs: signal: numpy array - A sequence of price points in time dt: int - Bucket size in seconds Outputs: dx: numpy array - First derivative at each point in points """ dx = np.zeros(len(signal)) dx[0] = (signal[1] - signal[0]) / dt dx[-1] = (signal[-1] - signal[-2]) for i in range(1, len(dx) - 1): dx[i] = (signal[i + 1] - signal[i - 1]) / (2 * dt) return dx ``` Then integrating this into the MACD strategy: ```python def macd_strategy3(ohlcv): """ Calculate the macd of a stock, decide whether to buy, sell, or hold based on signal behaviour Inputs: ohlcv: numpy array - A 5 x N matrix with rows corresponding to o, h, l, c, v respectively Outputs: status: string - "BUY", "SELL", or "HOLD" """ typical = ta.typical_price(ohlcv) macd, macd_sig, macd_hist = ta.macd(typical) dx = ta.first_deriv(typical, 1)[::-1] macd_hist = macd_hist[::-1] # reversed MACD histogram if macd_hist[0] > 0 and macd_hist[1] <= 0 and dx[0] > 0: return "BUY" elif macd_hist[0] < 0 and macd_hist[1] >= 0 and dx[0] < 0: return "SELL" else: return "HOLD" ``` 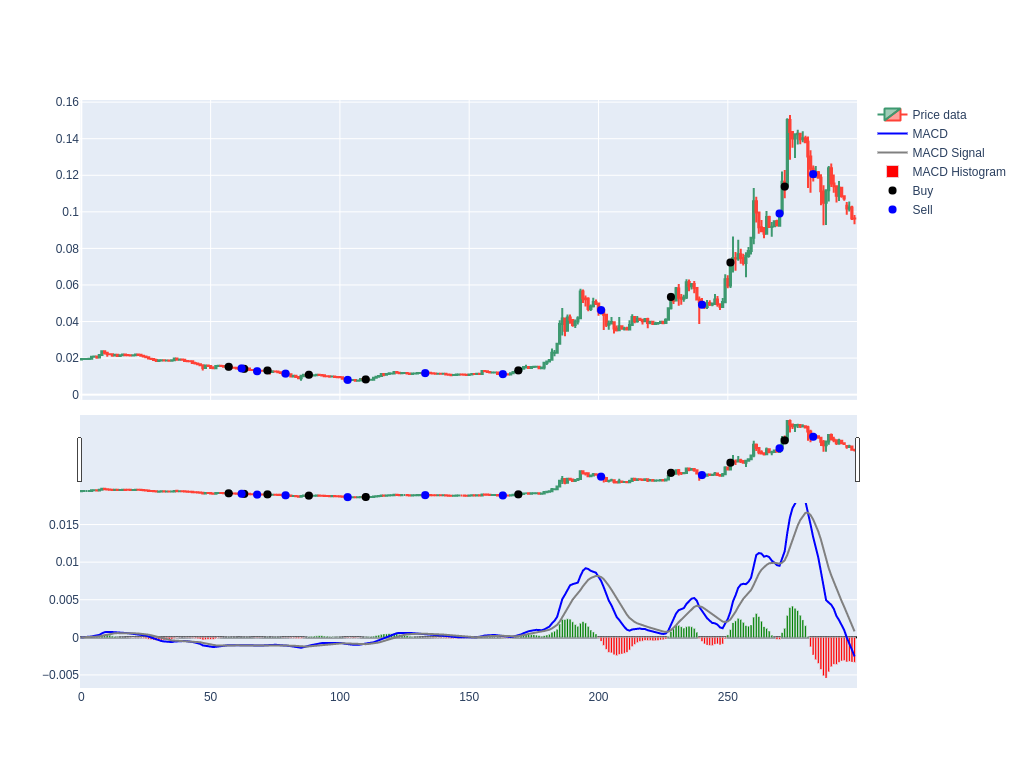 I had high hopes for this approach, but it didn't seem to have much effect at all. # Results Neither of these approaches do a particularly good job of cleaning up the signals generated by the original [MACD strategy](https://leofinance.io/hive-167922/@chasmic-cosm/automated-trading-with-the-macd-in-python). Usually the MACD is used in conjunction with other indicators in order to provide more reliable trading signals. Posted Using [LeoFinance](https://leofinance.io/@chasmic-cosm/attempting-to-improve-on-the-macd-strategy-in-python)
author | chasmic-cosm |
---|---|
permlink | attempting-to-improve-on-the-macd-strategy-in-python |
category | hive-167922 |
json_metadata | {"tags":["investing","trading","finance","maths","programming","python","leofinance"],"image":["https://images.hive.blog/DQmUKPq2bNviTCYYSVK1KTc2CxV3qkzhpLwRX1nDFzhNfsS/macd%20reference1.png","https://images.hive.blog/DQmZ7rtr2eJLPqK3Q4GijyLtp14nMRvyvpc73jAk66s5ESo/approach%201.png","https://images.hive.blog/DQmRGtjx5wBztBNJq1fnx6NyHNo2sYmFgubzERM1bBCSCqc/approach%202.png"],"links":["https://leofinance.io/hive-167922/@chasmic-cosm/automated-trading-with-the-macd-in-python","https://leofinance.io/hive-167922/@chasmic-cosm/calculating-the-macd-in-python"],"app":"leofinance/0.1","format":"markdown","canonical_url":"https://leofinance.io/@chasmic-cosm/attempting-to-improve-on-the-macd-strategy-in-python"} |
created | 2020-08-10 19:11:12 |
last_update | 2020-08-10 19:11:12 |
depth | 0 |
children | 1 |
last_payout | 2020-08-17 19:11:12 |
cashout_time | 1969-12-31 23:59:59 |
total_payout_value | 0.748 HBD |
curator_payout_value | 0.718 HBD |
pending_payout_value | 0.000 HBD |
promoted | 0.000 HBD |
body_length | 4,213 |
author_reputation | 4,159,775,155,640 |
root_title | "Attempting to improve on the MACD strategy in python" |
beneficiaries | [] |
max_accepted_payout | 1,000,000.000 HBD |
percent_hbd | 10,000 |
post_id | 99,000,637 |
net_rshares | 4,283,006,182,173 |
author_curate_reward | "" |
voter | weight | wgt% | rshares | pct | time |
---|---|---|---|---|---|
ereismatias | 0 | 1,145,745,854 | 100% | ||
fiona777 | 0 | 673,501,131 | 100% | ||
alinix | 0 | 4,323,225,890 | 100% | ||
mlmtraffic | 0 | 1,810,932,479 | 100% | ||
bobbythegreat | 0 | 1,589,315,781 | 100% | ||
yourseldom | 0 | 690,396,068 | 100% | ||
aewind | 0 | 77,939,835 | 100% | ||
decebal2dac | 0 | 8,051,040,963 | 100% | ||
bigtakosensei | 0 | 45,416,462,688 | 100% | ||
cardboard | 0 | 14,253,751,539 | 100% | ||
networker5 | 0 | 4,617,922,275 | 100% | ||
catulhu | 0 | 205,343,937 | 100% | ||
kingyus | 0 | 3,379,363,234 | 100% | ||
papadimos | 0 | 1,276,956,673 | 10% | ||
aaliyahholt | 0 | 17,111,699,683 | 100% | ||
danstatus | 0 | 14,156,809,254 | 100% | ||
angry0historian | 0 | 732,382,074 | 100% | ||
cristo | 0 | 0 | 100% | ||
decaptain | 0 | 0 | 100% | ||
siswadi | 0 | 1,424,542,447 | 100% | ||
sirjaxxy | 0 | 979,062,775 | 100% | ||
marlucco | 0 | 4,341,359,937 | 100% | ||
suhaimi | 0 | 2,873,450,550 | 100% | ||
paasz | 0 | 29,462,485,473 | 100% | ||
mcoinz79 | 0 | 1,475,632,486 | 1% | ||
waihlyan123 | 0 | 0 | 100% | ||
fandy | 0 | 43,435,441 | 100% | ||
sketcher | 0 | 1,538,656,851 | 100% | ||
min2thant | 0 | 328,569,526 | 100% | ||
khalil319 | 0 | 1,171,410,915 | 50% | ||
yagoub | 0 | 451,465,053 | 100% | ||
gamersnews | 0 | 3,215,704,824 | 100% | ||
jgr33nwood | 0 | 810,883,785 | 100% | ||
earthion | 0 | 98,987,115 | 100% | ||
adnanbtc | 0 | 0 | 100% | ||
xtophercruzeu | 0 | 455,280,078 | 100% | ||
phungminhtuan | 0 | 14,013,393 | 100% | ||
bitcoin-123 | 0 | 138,154,952 | 100% | ||
mdominicorobin | 0 | 234,899,170 | 100% | ||
garytyme | 0 | 153,330,925 | 100% | ||
claudiaz | 0 | 17,277,930,743 | 100% | ||
khaleelkazi | 0 | 337,960,003,664 | 100% | ||
maaz23 | 0 | 539,361,929 | 50% | ||
cryptoconfiance | 0 | 2,444,343,097 | 100% | ||
dejan.vuckovic | 0 | 33,625,281,656 | 100% | ||
midobashamido | 0 | 25,976,634,177 | 100% | ||
lowesyposey | 0 | 135,703,983 | 100% | ||
amulla505 | 0 | 150,625,662 | 100% | ||
joordanzzz | 0 | 15,260,688,836 | 100% | ||
sitasharma | 0 | 1,960,911,074 | 100% | ||
anniversary | 0 | 2,535,519,699 | 100% | ||
mrscwin | 0 | 662,225,663 | 100% | ||
eropromo | 0 | 3,562,703,056 | 100% | ||
resteemerbot | 0 | 553,432,268 | 100% | ||
kenjidois | 0 | 138,822,843 | 100% | ||
kpd-244 | 0 | 2,216,666,436 | 100% | ||
shajj | 0 | 679,188,485 | 100% | ||
vishaladatala | 0 | 2,194,486,391 | 100% | ||
kdjakirtgl | 0 | 545,633,100 | 100% | ||
dylen | 0 | 214,561,728 | 100% | ||
os1 | 0 | 695,248,949 | 100% | ||
eleidap | 0 | 642,683,806 | 100% | ||
vempromundo | 0 | 3,943,548,462 | 100% | ||
fortunee | 0 | 1,763,683,009 | 100% | ||
smokeasare165 | 0 | 1,175,561,382 | 100% | ||
evernew | 0 | 1,091,623,156 | 100% | ||
invariable.muse | 0 | 595,064,404 | 100% | ||
nyeichanaung | 0 | 732,011,453 | 100% | ||
darkloard | 0 | 684,367,096 | 100% | ||
acheever | 0 | 56,706,563 | 100% | ||
najar10791 | 0 | 943,342,457 | 100% | ||
yuri22 | 0 | 671,363,165 | 100% | ||
fantom1 | 0 | 719,021,587 | 100% | ||
psicoparedes | 0 | 737,364,905 | 100% | ||
naylinn | 0 | 1,080,689,503 | 100% | ||
platomaniac | 0 | 11,495,634,111 | 100% | ||
danishnawaz | 0 | 1,428,700,525 | 100% | ||
nicksmitley | 0 | 3,368,047,946 | 100% | ||
moazbakry1 | 0 | 822,141,483 | 100% | ||
investprosper | 0 | 6,632,347,657 | 14% | ||
andysign | 0 | 523,120,235 | 100% | ||
jason7282 | 0 | 3,294,918,298 | 100% | ||
hellenita | 0 | 206,917,069 | 100% | ||
intellihandling | 0 | 16,324,942,086 | 100% | ||
mcandceo | 0 | 2,938,143,427 | 100% | ||
charleswealth | 0 | 1,107,792,988 | 100% | ||
artoftherhyme | 0 | 55,591,256,237 | 100% | ||
markusmichael | 0 | 4,792,925,617 | 100% | ||
danishmobeen | 0 | 86,421,890 | 100% | ||
khard | 0 | 157,056,505 | 100% | ||
prometheus21 | 0 | 594,266,057 | 100% | ||
anonek | 0 | 222,205,034 | 100% | ||
joshh71390 | 0 | 11,789,321,759 | 100% | ||
stojay007 | 0 | 1,000,200,472 | 100% | ||
starlord28 | 0 | 93,881,733 | 100% | ||
fs-pyrotechnik | 0 | 248,214,090 | 100% | ||
hlaingwin | 0 | 136,473,786 | 100% | ||
myohein | 0 | 0 | 100% | ||
pyaytheinkhine | 0 | 0 | 100% | ||
tombs | 0 | 146,040,232 | 100% | ||
zinmintun7 | 0 | 6,161,517,628 | 100% | ||
marco42 | 0 | 1,023,244,370 | 50% | ||
godenlight | 0 | 60,822,919 | 100% | ||
mgmgnaing128 | 0 | 26,696,437 | 100% | ||
murizalpangeran | 0 | 1,435,550,769 | 100% | ||
harkadam2506 | 0 | 649,294,752 | 100% | ||
abdullahyusuf | 0 | 16,554,273,152 | 100% | ||
stefi11 | 0 | 675,693,876 | 50% | ||
crispycoinboys | 0 | 7,109,429,035 | 100% | ||
aron117 | 0 | 94,762,387 | 100% | ||
kyawlay1 | 0 | 1,112,093 | 100% | ||
geuratan | 0 | 0 | 100% | ||
gadrian | 0 | 12,493,952,209 | 8.4% | ||
xomegax | 0 | 335,467,059 | 100% | ||
cheerylay | 0 | 0 | 100% | ||
klone | 0 | 47,996,759 | 100% | ||
cryptocoinkb | 0 | 200,006,491,354 | 85% | ||
kokogyi1 | 0 | 98,646,722 | 100% | ||
mrkwh | 0 | 47,254,571 | 100% | ||
jarosalawszafran | 0 | 930,563,477 | 30% | ||
teaminfo | 0 | 133,438,539 | 100% | ||
oso111 | 0 | 750,932,569 | 100% | ||
mmidoaa | 0 | 562,590,684 | 100% | ||
adaboin | 0 | 306,336,106 | 100% | ||
loverlay | 0 | 0 | 100% | ||
nyanlynnhtay | 0 | 214,669,449 | 100% | ||
payroll | 0 | 101,720,214,874 | 2% | ||
myanmarvds | 0 | 27,923,414 | 100% | ||
eadenworld | 0 | 27,740,412 | 100% | ||
m3morito | 0 | 88,941 | 100% | ||
tabraiz.jutt | 0 | 255,840,584 | 100% | ||
igienistamentale | 0 | 796,186 | 100% | ||
leoxin | 0 | 0 | 100% | ||
enm1 | 0 | 22,829,472,100 | 100% | ||
trumpman2 | 0 | 3,461,739,539 | 31% | ||
positivexposure | 0 | 7,072,800,949 | 100% | ||
mammapiera57 | 0 | 144,880,244 | 100% | ||
pgrab86 | 0 | 926,579,786 | 100% | ||
roadbet | 0 | 187,337,384 | 100% | ||
lantracy | 0 | 23,430,437,699 | 100% | ||
russiandownload | 0 | 1,402,918,701 | 100% | ||
freshee | 0 | 318,766,171 | 100% | ||
xazid | 0 | 260,089,328 | 100% | ||
waggy6 | 0 | 5,456,029,421 | 100% | ||
justasperm | 0 | 3,283,333,696 | 100% | ||
bandit-keren | 0 | 0 | 100% | ||
nateaguila | 0 | 293,319,256,186 | 8% | ||
hlaingwin2 | 0 | 0 | 100% | ||
adam.tran | 0 | 11,413,133,242 | 100% | ||
sumaakter | 0 | 0 | 100% | ||
pyaethein1989 | 0 | 0 | 100% | ||
dtholy | 0 | 0 | 100% | ||
xonekaway | 0 | 0 | 100% | ||
stellapentra | 0 | 0 | 100% | ||
mrblueberry | 0 | 64,457,495 | 100% | ||
sobrietystuntin | 0 | 210,968,177 | 100% | ||
zayarzayar018 | 0 | 0 | 100% | ||
rupon89 | 0 | 603,352,366 | 100% | ||
doom42 | 0 | 78,983,486 | 100% | ||
nasel | 0 | 4,609,427,879 | 100% | ||
layphyu | 0 | 0 | 100% | ||
bc-i | 0 | 1,604,476,827 | 100% | ||
haikalfahmi | 0 | 536,620,420 | 100% | ||
goldbloger4 | 0 | 3,793,754 | 100% | ||
tangera | 0 | 953,927,912 | 100% | ||
fakheralamsher | 0 | 146,802,442 | 100% | ||
asm3 | 0 | 0 | 100% | ||
andresaulait | 0 | 407,759,207 | 100% | ||
imagendevoz | 0 | 3,092,232,742 | 100% | ||
romanolsamuels | 0 | 6,523,757,728 | 100% | ||
kally | 0 | 283,176,937 | 100% | ||
aungthu | 0 | 0 | 100% | ||
cnandofer | 0 | 2,244,019,472 | 100% | ||
cooper28 | 0 | 0 | 100% | ||
lancipdjiwa | 0 | 428,396,214 | 100% | ||
smartworld | 0 | 37,663,772 | 100% | ||
nin4i | 0 | 56,959,571 | 100% | ||
jpolitical96 | 0 | 120,759,042 | 100% | ||
airdropking | 0 | 1,510,830,910 | 100% | ||
supsnehal | 0 | 946,706,909 | 100% | ||
tigerstripe | 0 | 40,689,120,351 | 100% | ||
desposyni | 0 | 46,329,217 | 100% | ||
zinnech | 0 | 131,472,852 | 100% | ||
visualsketch | 0 | 1,260,310 | 100% | ||
puregrace | 0 | 4,609,702,177 | 100% | ||
stephbalanquit | 0 | 0 | 100% | ||
htikehtike00734 | 0 | 14,739,697 | 100% | ||
hayzer93 | 0 | 0 | 100% | ||
wunna | 0 | 0 | 100% | ||
wunna1 | 0 | 0 | 100% | ||
kakibukit | 0 | 6,616,778,440 | 100% | ||
biehany | 0 | 124,303,294 | 100% | ||
jayboi | 0 | 391,759,428 | 100% | ||
mvisions | 0 | 2,936,599,988 | 100% | ||
alamsher | 0 | 0 | 100% | ||
alibaaaaaa | 0 | 0 | 100% | ||
srl-zone | 0 | 1,538,050,612 | 100% | ||
ridor5301 | 0 | 777,622,809 | 100% | ||
az88822 | 0 | 174,410,417 | 100% | ||
ntamntam | 0 | 144,689,458 | 100% | ||
moelout | 0 | 142,821,720 | 100% | ||
zabatar | 0 | 144,271,847 | 100% | ||
konlon | 0 | 144,383,295 | 100% | ||
atalgyi | 0 | 33,007,928 | 100% | ||
sebafelician | 0 | 38,745,818 | 100% | ||
moenya | 0 | 0 | 100% | ||
audyavic | 0 | 0 | 100% | ||
cmalescov | 0 | 1,589,187,063 | 100% | ||
stream.studios | 0 | 0 | 100% | ||
minmin008 | 0 | 0 | 100% | ||
gridbox | 0 | 660,787,822 | 100% | ||
vhhau | 0 | 991,918,310 | 100% | ||
vixmemon | 0 | 2,088,918,670 | 100% | ||
minmyatthu | 0 | 0 | 100% | ||
kyawmin | 0 | 0 | 100% | ||
jhaniz | 0 | 0 | 100% | ||
ayaennaciri | 0 | 493,338,018 | 100% | ||
laycho | 0 | 58,199,441 | 100% | ||
bodymaual | 0 | 275,591,389 | 100% | ||
rossilay | 0 | 0 | 100% | ||
collect1 | 0 | 142,405,409 | 100% | ||
shwemoetae | 0 | 0 | 100% | ||
payi | 0 | 144,548,199 | 100% | ||
land1 | 0 | 142,588,490 | 100% | ||
dvice | 0 | 998,065,212 | 100% | ||
cryptouno | 0 | 245,656,943 | 100% | ||
tamilcharitycoin | 0 | 533,992,838 | 100% | ||
miladopensador | 0 | 0 | 100% | ||
venkatesh15921 | 0 | 923,689,745 | 100% | ||
becan | 0 | 133,034,595 | 100% | ||
domey | 0 | 0 | 100% | ||
crantar | 0 | 142,234,634 | 100% | ||
dream9 | 0 | 144,770,358 | 100% | ||
ryanma0723 | 0 | 270,378,900 | 100% | ||
steemit-games | 0 | 132,985,486 | 100% | ||
waihein | 0 | 75,668,741 | 100% | ||
amaron | 0 | 0 | 100% | ||
atalay6 | 0 | 0 | 100% | ||
gface | 0 | 0 | 100% | ||
bipin7 | 0 | 46,399,270 | 100% | ||
prabhav | 0 | 0 | 100% | ||
farelsteem | 0 | 0 | 100% | ||
sayeds1956 | 0 | 373,690,090 | 100% | ||
emmaeliza | 0 | 0 | 100% | ||
laissez-faire | 0 | 20,724,004 | 100% | ||
ateeqbj | 0 | 130,725,915 | 100% | ||
emir54 | 0 | 709,783,058 | 100% | ||
ritikdokania23 | 0 | 120,025,094 | 100% | ||
leonidastouch | 0 | 523,122,660 | 100% | ||
tup.crypto | 0 | 652,684,774 | 100% | ||
deepakpathania | 0 | 38,204,611 | 100% | ||
ishan89 | 0 | 533,280,826 | 100% | ||
arslannasir9090 | 0 | 464,150,723 | 100% | ||
gaurav9971 | 0 | 23,753,201 | 100% | ||
nankhan | 0 | 0 | 100% | ||
thaw | 0 | 0 | 100% | ||
hamko | 0 | 0 | 100% | ||
dktrending341 | 0 | 152,988,133 | 100% | ||
anroja | 0 | 6,789,497,486 | 100% | ||
jamespradhan | 0 | 51,648,516 | 100% | ||
rabiagilani | 0 | 0 | 100% | ||
drd.olek | 0 | 734,577,222 | 100% | ||
memeholic | 0 | 0 | 100% | ||
franciferrer | 0 | 2,241,304,461 | 100% | ||
sohelsarowar | 0 | 144,615,499 | 100% | ||
necho41 | 0 | 1,174,684,689 | 100% | ||
kazbo | 0 | 210,194,214 | 100% | ||
shoutloud | 0 | 31,108,954 | 100% | ||
manika | 0 | 128,016,500 | 100% | ||
cakemonster | 0 | 57,282,463,241 | 50% | ||
arena11 | 0 | 0 | 100% | ||
fedesh | 0 | 906,252,854 | 100% | ||
bluebella | 0 | 0 | 100% | ||
robertpayne114 | 0 | 182,418,343 | 100% | ||
rocketblog | 0 | 72,890,978 | 100% | ||
arthemis | 0 | 442,730,176 | 100% | ||
thewanderingwind | 0 | 2,913,915,846 | 100% | ||
lostprophet | 0 | 4,036,110,489 | 100% | ||
miftac | 0 | 54,945,303 | 100% | ||
caliboycalwayne | 0 | 724,604,039 | 100% | ||
bulia | 0 | 0 | 100% | ||
bunchtale | 0 | 325,436,219 | 100% | ||
gargi | 0 | 26,067,014,204 | 100% | ||
klaranlage | 0 | 56,775,138 | 100% | ||
limax21 | 0 | 667,446,196 | 100% | ||
limka | 0 | 0 | 5% | ||
busenversteher | 0 | 73,691,125 | 100% | ||
myquotes | 0 | 0 | 100% | ||
minacorpo | 0 | 74,060,883 | 100% | ||
killeroase | 0 | 3,005,042 | 100% | ||
buddha-ist-tot | 0 | 0 | 100% | ||
cehu | 0 | 0 | 100% | ||
schach | 0 | 2,826,693,368 | 100% | ||
mersedes | 0 | 358,578,511 | 100% | ||
zaenach73 | 0 | 758,662,727 | 100% | ||
archiv | 0 | 0 | 100% | ||
stellafranco | 0 | 13,746,302 | 100% | ||
lifequote | 0 | 0 | 100% | ||
halli-hallo | 0 | 517,851,144 | 100% | ||
geiwoyibeipijiu | 0 | 190,583,010 | 100% | ||
engrsayful | 0 | 2,244,404,676 | 5.6% | ||
arthur.grafo4 | 0 | 6,900,159,635 | 100% | ||
a-x | 0 | 0 | 100% | ||
yoshi24517 | 0 | 2,809,257,800 | 100% | ||
nullnull | 0 | 59,265,890 | 100% | ||
klohsdieserwelt | 0 | 293,211,002 | 100% | ||
klismois | 0 | 1,632,114,179 | 100% | ||
transom | 0 | 145,650,966,487 | 100% | ||
hundemama | 0 | 1,879,132,991 | 100% | ||
dxc | 0 | 267,643,809 | 100% | ||
cryptof | 0 | 67,645,279 | 100% | ||
trontokens | 0 | 543,354,990 | 100% | ||
rempav | 0 | 323,043,296 | 100% | ||
savetheday | 0 | 13,499,273 | 100% | ||
haxxdump | 0 | 19,777,399 | 10% | ||
vinay1999 | 0 | 42,138,890 | 100% | ||
blueboar3 | 0 | 1,118,034,413 | 100% | ||
darkphoenixapoc | 0 | 12,987,451,117 | 100% | ||
hoss1 | 0 | 552,822,024 | 100% | ||
hoss2 | 0 | 695,776,202 | 100% | ||
fahmy1 | 0 | 726,634,639 | 100% | ||
sheko1 | 0 | 674,097,948 | 100% | ||
sheko2 | 0 | 576,117,087 | 100% | ||
abdelzaher2 | 0 | 793,874,459 | 100% | ||
abdelzaherr | 0 | 751,599,132 | 100% | ||
bittyd | 0 | 673,099,792 | 100% | ||
sonyeye | 0 | 239,507,507 | 100% | ||
leo.voter | 0 | 2,292,548,565,654 | 28% | ||
leo.curator | 0 | 2,994,698,518 | 97% | ||
vxc.leo | 0 | 222,487,982 | 100% | ||
zaku-leo | 0 | 989,065,222 | 28% | ||
denise.menya | 0 | 133,634,700 | 100% | ||
midlet-yourleo | 0 | 1,311,858,213 | 97% | ||
eloi | 0 | 890,426,495 | 100% | ||
refinement | 0 | 539,152,197 | 100% | ||
tomhall.leo | 0 | 311,012,718 | 100% | ||
kawalingpinoy | 0 | 0 | 100% | ||
deflacion | 0 | 980,527,188 | 9.9% | ||
smf37 | 0 | 1,195,620,666 | 100% | ||
spinvest-leo | 0 | 347,722,090 | 5% | ||
nitin21 | 0 | 283,310,540 | 100% | ||
astraeas | 0 | 1,026,313,267 | 100% | ||
akpofure | 0 | 440,301,206 | 100% | ||
chloem | 0 | 1,030,887,566 | 100% | ||
leotrail | 0 | 1,096,367,646 | 99.5% | ||
sqljoker | 0 | 3,627,506,365 | 100% | ||
snoochieboochies | 0 | 12,044,885,720 | 31% | ||
autowin | 0 | 763,250,223 | 70% | ||
toni.curation | 0 | 141,703,190 | 4% | ||
tariqul.bibm | 0 | 3,427,295,142 | 14% | ||
asepandriyana | 0 | 2,537,287,542 | 100% | ||
rehan.blog | 0 | 551,867,946 | 28% | ||
empoderat.leo | 0 | 59,890,746 | 31% | ||
platino94 | 0 | 15,833,707,255 | 31% | ||
steemage | 0 | 443,800,454 | 100% | ||
rehan-leo | 0 | 1,252,479,730 | 100% | ||
bukitberbunga | 0 | 485,255,134 | 100% | ||
pinggangbukit | 0 | 479,458,828 | 100% | ||
sgerhart | 0 | 15,160,484,954 | 100% | ||
punggungbukit | 0 | 471,047,117 | 100% | ||
leofinance | 0 | 14,746,346,870 | 28% | ||
lembahbukit | 0 | 540,220,025 | 100% | ||
capingbukit | 0 | 530,019,018 | 100% | ||
sawahbukit | 0 | 836,697,854 | 100% | ||
ladangbukit | 0 | 529,053,182 | 100% | ||
bukitpinus | 0 | 482,096,779 | 100% | ||
oberhessen | 0 | 1,617,937,813 | 100% | ||
amateur2020 | 0 | 1,647,745,821 | 100% | ||
bukitcemara | 0 | 457,680,075 | 100% | ||
anakbukit | 0 | 394,118,822 | 100% | ||
adechina | 0 | 1,339,046,533 | 100% | ||
bukitbatu | 0 | 3,526,393,495 | 100% | ||
adamsidney | 0 | 49,569,843 | 10% | ||
bukitawan | 0 | 3,579,993,852 | 100% | ||
bukittandus | 0 | 3,537,460,056 | 100% | ||
reward.app | 0 | 14,684,937,333 | 20% |
<center>UpvoteBank</center> | <center>Your upvote bank</center> ------------ | ------------- 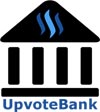 | This post have been upvoted by the @UpvoteBank service. Want to know more and receive "free" upvotes click [here](https://steemit.com/steemit/@upvotebank/gzsr5aw6)
author | upvotebank |
---|---|
permlink | 20200810t191136430z |
category | hive-167922 |
json_metadata | {"tags":["comment"],"app":"steemjs/comment"} |
created | 2020-08-10 19:11:36 |
last_update | 2020-08-10 19:11:36 |
depth | 1 |
children | 0 |
last_payout | 2020-08-17 19:11:36 |
cashout_time | 1969-12-31 23:59:59 |
total_payout_value | 0.000 HBD |
curator_payout_value | 0.000 HBD |
pending_payout_value | 0.000 HBD |
promoted | 0.000 HBD |
body_length | 354 |
author_reputation | 44,533,286,469,385 |
root_title | "Attempting to improve on the MACD strategy in python" |
beneficiaries | [] |
max_accepted_payout | 1,000,000.000 HBD |
percent_hbd | 10,000 |
post_id | 99,000,644 |
net_rshares | -5,347,465,312 |
author_curate_reward | "" |
voter | weight | wgt% | rshares | pct | time |
---|---|---|---|---|---|
spaminator | 0 | -5,347,465,312 | -0.25% |