 [source](https://pixabay.com/de/photos/audit-buchhaltung-finanzbuchhaltung-4190945/) I was trying to count all my STEEM/SBD balances for my tax report as I found out that the STEEM account history api is not reliable: ``` #!/usr/bin/python from beem import Steem from beem.account import Account from beem.amount import Amount from beem.nodelist import NodeList if __name__ == "__main__": nodelist = NodeList() nodelist.update_nodes() stm = Steem(node=nodelist.get_steem_nodes()) print(stm) account_name = "holger80" account = Account(account_name, steem_instance=stm) ops_dict = {} for ops in account.history(): ops_dict[ops["index"]] = ops STEEM = 0 SBD = 0 index = 0 for _id in sorted(list(ops_dict.keys())): ops = ops_dict[_id] if ops["type"] == "fill_convert_request": amount_out = Amount(ops["amount_out"], blockchain_instance=stm) amount_in = Amount(ops["amount_in"], blockchain_instance=stm) STEEM += float(amount_out) SBD -= float(amount_in) elif ops["type"] == "fill_transfer_from_savings": amount = Amount(ops["amount"], steem_instance=stm) if ops["to"] == account_name: if amount.symbol == "STEEM": STEEM += float(amount) else: SBD += float(amount) elif ops["type"] == "transfer_to_savings": amount = Amount(ops["amount"], steem_instance=stm) if amount.symbol == "STEEM": STEEM -= float(amount) else: SBD -= float(amount) elif ops["type"] == "transfer_to_vesting": amount = Amount(ops["amount"], steem_instance=stm) if ops["from"] == account_name and ops["to"] == account_name: STEEM -= float(amount) elif ops["from"] == account_name: STEEM -= float(amount) index += 1 elif ops["type"] == "fill_vesting_withdraw": amount = Amount(ops["deposited"], steem_instance=stm) if ops["to_account"] == account_name: STEEM += float(amount) elif ops["type"] == "proposal_pay": amount = Amount(ops["payment"], steem_instance=stm) SBD += float(amount) index += 1 elif ops["type"] == "create_proposal": SBD -= 10 index += 1 elif ops["type"] == "transfer": amount = Amount(ops["amount"], steem_instance=stm) if ops["from"] == account_name and ops["to"] == account_name: continue if ops["to"] == account_name: if amount.symbol == "STEEM": STEEM += float(amount) else: SBD += float(amount) else: if amount.symbol == "STEEM": STEEM -= float(amount) else: SBD -= float(amount) index += 1 elif ops["type"] == "account_create_with_delegation": if ops["new_account_name"] == account_name: continue fee = Amount(ops["fee"], blockchain_instance=stm) STEEM -= float(fee) elif ops["type"] == "account_create": if ops["new_account_name"] == account_name: continue fee = Amount(ops["fee"], blockchain_instance=stm) STEEM -= float(fee) elif ops["type"] == "claim_reward_balance": reward_steem = Amount(ops["reward_steem"], steem_instance=stm) reward_vests = Amount(ops["reward_vests"], steem_instance=stm) reward_sbd = Amount(ops["reward_sbd"], steem_instance=stm) if float(reward_steem) > 0: STEEM += float(reward_steem) if float(reward_sbd) > 0: SBD += float(reward_sbd) index += 1 elif ops["type"] == "fill_order": open_pays = Amount(ops["open_pays"], steem_instance=stm) current_pays = Amount(ops["current_pays"], steem_instance=stm) open_owner = ops["open_owner"] current_owner = ops["current_owner"] if current_owner == account_name: if open_pays.symbol == "STEEM": STEEM += float(open_pays) SBD -= float(current_pays) else: SBD += float(open_pays) STEEM -= float(current_pays) else: if current_pays.symbol == "STEEM": STEEM += float(current_pays) SBD -= float(open_pays) else: SBD += float(current_pays) STEEM -= float(open_pays) index += 1 print("%.3f STEEM, %.3f SBD" % (STEEM, SBD)) ``` returns ``` 6115.776 STEEM, -3.775 SBD ``` which is completely wrong. First it cannot be negative and second [steemd.com](https://steemd.com/@holger80) shows 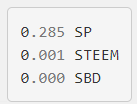 After investigating, I found out that some account history elements are shown twice. ## 1. check: Works beem as it should? E.g. beem returns: ``` print(ops_dict[150050]) print(ops_dict[150053]) ``` ``` {'current_owner': 'holger80', 'current_orderid': 1584865440, 'current_pays': {'amount': '3775', 'precision': 3, 'nai': '@@000000013'}, 'open_owner': 'cst90', 'open_orderid': 1584865425, 'open_pays': {'amount': '20000', 'precision': 3, 'nai': '@@000000021'}, 'trx_id': '0a3200e502bd6b1c06b81c2a2337e0436fc13704', 'block': 41868606, 'trx_in_block': 24, 'op_in_trx': 0, 'virtual_op': 1, 'timestamp': '2020-03-22T08:24:00', 'account': 'holger80', 'type': 'fill_order', '_id': '60ccd83432667fccb51946202811a6d65f68a6ea', 'index': 150050} {'current_owner': 'holger80', 'current_orderid': 1584865440, 'current_pays': {'amount': '3775', 'precision': 3, 'nai': '@@000000013'}, 'open_owner': 'cst90', 'open_orderid': 1584865425, 'open_pays': {'amount': '20000', 'precision': 3, 'nai': '@@000000021'}, 'trx_id': '0a3200e502bd6b1c06b81c2a2337e0436fc13704', 'block': 41868606, 'trx_in_block': 24, 'op_in_trx': 0, 'virtual_op': 1, 'timestamp': '2020-03-22T08:24:00', 'account': 'holger80', 'type': 'fill_order', '_id': '60ccd83432667fccb51946202811a6d65f68a6ea', 'index': 150053} ``` Which is also shown on steemd.com: 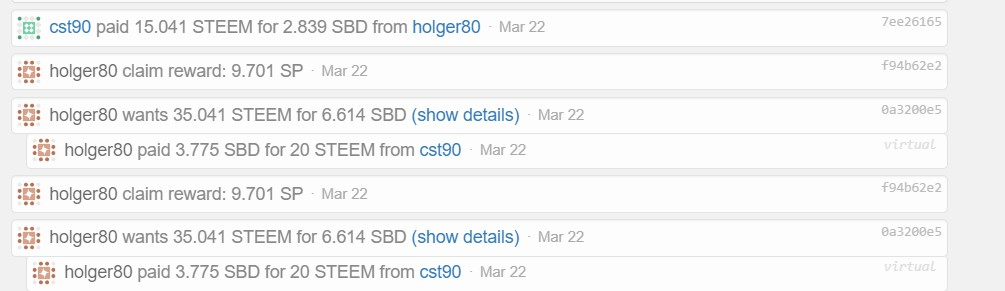 The error is not caused by beem. ## 2. check: What is stored in the trx id? https://steemd.com/tx/0a3200e502bd6b1c06b81c2a2337e0436fc13704 retuns only one operation: 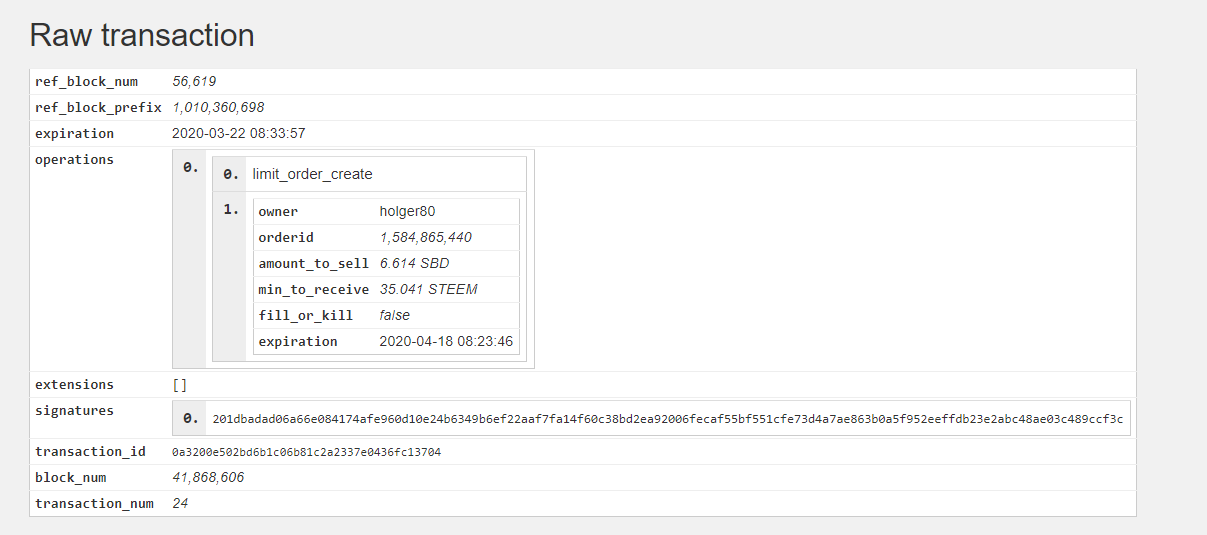 ## 3. check: What shows the block? https://steemd.com/b/41868606#0a3200e502bd6b1c06b81c2a2337e0436fc13704 shows that there is only one operation: 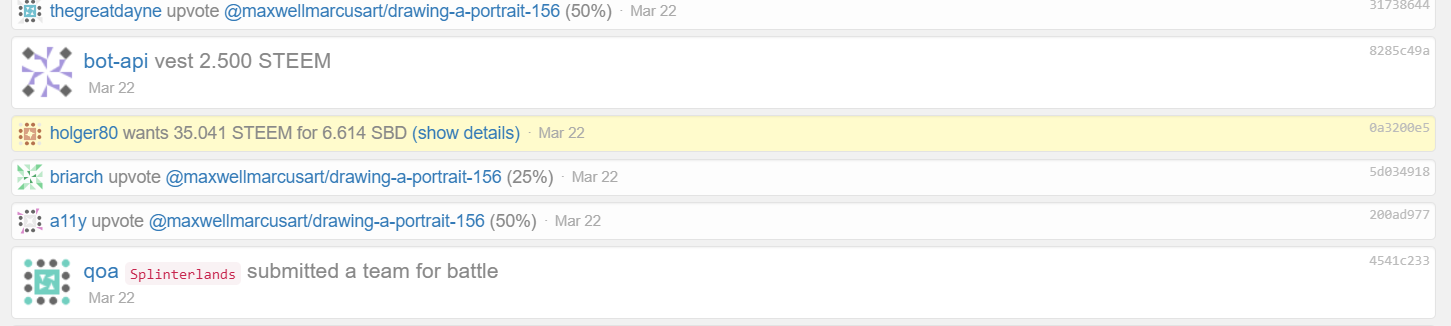 ## How to fix? I'm using the internal `_id` parameter from beem for this. For every operation, a unique identifier is calculated. Whenever when two operations are identically, their `_id` values are identically. I use this `_id` to find all duplicate entries and count then all operations with the same transaction id in the block. When there are more entries than counted operations, I mark the surplus entries as duplicate. ``` #!/usr/bin/python from beem import Steem from beem.block import Block from beem.account import Account from beem.amount import Amount from beem.nodelist import NodeList if __name__ == "__main__": nodelist = NodeList() nodelist.update_nodes() stm = Steem(node=nodelist.get_steem_nodes()) print(stm) account_name = "holger80" account = Account(account_name, steem_instance=stm) ops_dict = {} _ids = {} for ops in account.history(): ops_dict[ops["index"]] = ops if ops["_id"] in _ids: _ids[ops["_id"]] += 1 else: _ids[ops["_id"]] = 1 duplicate_indices = [] _id_list = [] for _id in sorted(list(ops_dict.keys())): ops = ops_dict[_id] if _ids[ops["_id"]] == 1: continue if ops["_id"] not in _id_list: _id_list.append(ops["_id"]) else: trx_id = ops["trx_id"] if trx_id == "0000000000000000000000000000000000000000": duplicate_indices.append(ops["index"]) else: block = Block(ops["block"], blockchain_instance=stm) count_ops = 0 for t in block.transactions: if t["transaction_id"] != trx_id: continue for o in t["operations"]: count_ops += 1 if count_ops < _ids[ops["_id"]]: duplicate_indices.append(ops["index"]) STEEM = 0 SBD = 0 index = 0 print("duplicate indices %d" % len(duplicate_indices)) for _id in sorted(list(ops_dict.keys())): ops = ops_dict[_id] if _id in duplicate_indices: continue if ops["type"] == "fill_convert_request": amount_out = Amount(ops["amount_out"], blockchain_instance=stm) amount_in = Amount(ops["amount_in"], blockchain_instance=stm) STEEM += float(amount_out) SBD -= float(amount_in) index += 1 elif ops["type"] == "fill_transfer_from_savings": amount = Amount(ops["amount"], steem_instance=stm) if ops["to"] == account_name: if amount.symbol == "STEEM": STEEM += float(amount) else: SBD += float(amount) index += 1 elif ops["type"] == "transfer_to_savings": amount = Amount(ops["amount"], steem_instance=stm) if amount.symbol == "STEEM": STEEM -= float(amount) else: SBD -= float(amount) index += 1 elif ops["type"] == "transfer_to_vesting": amount = Amount(ops["amount"], steem_instance=stm) if ops["from"] == account_name and ops["to"] == account_name: STEEM -= float(amount) elif ops["from"] == account_name: STEEM -= float(amount) index += 1 elif ops["type"] == "fill_vesting_withdraw": amount = Amount(ops["deposited"], steem_instance=stm) if ops["to_account"] == account_name: STEEM += float(amount) index += 1 elif ops["type"] == "proposal_pay": amount = Amount(ops["payment"], steem_instance=stm) SBD += float(amount) index += 1 elif ops["type"] == "create_proposal": SBD -= 10 index += 1 elif ops["type"] == "transfer": amount = Amount(ops["amount"], steem_instance=stm) if ops["from"] == account_name and ops["to"] == account_name: continue if ops["to"] == account_name: if amount.symbol == "STEEM": STEEM += float(amount) else: SBD += float(amount) else: if amount.symbol == "STEEM": STEEM -= float(amount) else: SBD -= float(amount) index += 1 elif ops["type"] == "account_create_with_delegation": if ops["new_account_name"] == account_name: continue fee = Amount(ops["fee"], blockchain_instance=stm) STEEM -= float(fee) index += 1 elif ops["type"] == "account_create": if ops["new_account_name"] == account_name: continue fee = Amount(ops["fee"], blockchain_instance=stm) STEEM -= float(fee) index += 1 elif ops["type"] == "claim_reward_balance": reward_steem = Amount(ops["reward_steem"], steem_instance=stm) reward_vests = Amount(ops["reward_vests"], steem_instance=stm) reward_sbd = Amount(ops["reward_sbd"], steem_instance=stm) if float(reward_steem) > 0: STEEM += float(reward_steem) if float(reward_sbd) > 0: SBD += float(reward_sbd) index += 1 elif ops["type"] == "fill_order": open_pays = Amount(ops["open_pays"], steem_instance=stm) current_pays = Amount(ops["current_pays"], steem_instance=stm) open_owner = ops["open_owner"] current_owner = ops["current_owner"] if current_owner == account_name: if open_pays.symbol == "STEEM": STEEM += float(open_pays) SBD -= float(current_pays) else: SBD += float(open_pays) STEEM -= float(current_pays) else: if current_pays.symbol == "STEEM": STEEM += float(current_pays) SBD -= float(open_pays) else: SBD += float(current_pays) STEEM -= float(open_pays) index += 1 print("%d entries" % index) print("%.3f STEEM, %.3f SBD" % (STEEM, SBD)) ``` This time, the script returns: ``` duplicate indices 26 0.001 STEEM, 0.000 SBD ``` which is now correct. After removing 26 duplicate entries, my balance is correct. ## Is there also a problem with duplicate entries on Hive? ``` #!/usr/bin/python from beem import Hive from beem.account import Account from beem.amount import Amount from beem.block import Block from beem.nodelist import NodeList if __name__ == "__main__": nodelist = NodeList() nodelist.update_nodes() stm = Hive(node=nodelist.get_hive_nodes()) print(stm) account_name = "holger80" account = Account(account_name, blockchain_instance=stm) ops_dict = {} _ids = {} for ops in account.history(): ops_dict[ops["index"]] = ops if ops["_id"] in _ids: _ids[ops["_id"]] += 1 else: _ids[ops["_id"]] = 1 duplicate_indices = [] _id_list = [] for _id in sorted(list(ops_dict.keys())): ops = ops_dict[_id] if _ids[ops["_id"]] == 1: continue if ops["_id"] not in _id_list: _id_list.append(ops["_id"]) else: trx_id = ops["trx_id"] if trx_id == "0000000000000000000000000000000000000000": duplicate_indices.append(ops["index"]) else: block = Block(ops["block"], blockchain_instance=stm) count_ops = 0 for t in block.transactions: if t["transaction_id"] != trx_id: continue for o in t["operations"]: count_ops += 1 if count_ops < _ids[ops["_id"]]: duplicate_indices.append(ops["index"]) type_count = {} for _id in sorted(list(ops_dict.keys())): ops = ops_dict[_id] if ops["type"] in type_count: type_count[ops["type"]] += 1 else: type_count[ops["type"]] = 1 HIVE = 0 HBD = 0 index = 0 print("duplicate indices %d" % len(duplicate_indices)) for _id in sorted(list(ops_dict.keys())): ops = ops_dict[_id] if _id in duplicate_indices: continue if ops["type"] == "fill_convert_request": amount_out = Amount(ops["amount_out"], blockchain_instance=stm) amount_in = Amount(ops["amount_in"], blockchain_instance=stm) HIVE += float(amount_out) HBD -= float(amount_in) index += 1 elif ops["type"] == "fill_transfer_from_savings": amount = Amount(ops["amount"], blockchain_instance=stm) if ops["to"] == account_name: if amount.symbol == "HIVE": HIVE += float(amount) else: HBD += float(amount) index += 1 elif ops["type"] == "transfer_to_savings": amount = Amount(ops["amount"], blockchain_instance=stm) if amount.symbol == "HIVE": HIVE -= float(amount) else: HBD -= float(amount) index += 1 elif ops["type"] == "transfer_to_vesting": amount = Amount(ops["amount"], blockchain_instance=stm) if ops["from"] == account_name and ops["to"] == account_name: HIVE -= float(amount) elif ops["from"] == account_name: HIVE -= float(amount) index += 1 elif ops["type"] == "fill_vesting_withdraw": amount = Amount(ops["deposited"], blockchain_instance=stm) if ops["to_account"] == account_name: HIVE += float(amount) index += 1 elif ops["type"] == "proposal_pay": amount = Amount(ops["payment"], blockchain_instance=stm) HBD += float(amount) index += 1 elif ops["type"] == "create_proposal": HBD -= 10 index += 1 elif ops["type"] == "transfer": amount = Amount(ops["amount"], blockchain_instance=stm) if ops["from"] == account_name and ops["to"] == account_name: continue if ops["to"] == account_name: if amount.symbol == "HIVE": HIVE += float(amount) else: HBD += float(amount) else: if amount.symbol == "HIVE": HIVE -= float(amount) else: HBD -= float(amount) index += 1 elif ops["type"] == "account_create_with_delegation": if ops["new_account_name"] == account_name: continue fee = Amount(ops["fee"], blockchain_instance=stm) HIVE -= float(fee) index += 1 elif ops["type"] == "account_create": if ops["new_account_name"] == account_name: continue fee = Amount(ops["fee"], blockchain_instance=stm) HIVE -= float(fee) index += 1 elif ops["type"] == "claim_reward_balance": reward_steem = Amount(ops["reward_steem"], blockchain_instance=stm) reward_vests = Amount(ops["reward_vests"], blockchain_instance=stm) reward_sbd = Amount(ops["reward_sbd"], blockchain_instance=stm) if float(reward_steem) > 0: HIVE += float(reward_steem) if float(reward_sbd) > 0: HBD += float(reward_sbd) index += 1 elif ops["type"] == "fill_order": open_pays = Amount(ops["open_pays"], blockchain_instance=stm) current_pays = Amount(ops["current_pays"], blockchain_instance=stm) open_owner = ops["open_owner"] current_owner = ops["current_owner"] if current_owner == account_name: if open_pays.symbol == "HIVE": HIVE += float(open_pays) HBD -= float(current_pays) else: HBD += float(open_pays) HIVE -= float(current_pays) else: if current_pays.symbol == "HIVE": HIVE += float(current_pays) HBD -= float(open_pays) else: HBD += float(current_pays) HIVE -= float(open_pays) index += 1 print("%d entries" % index) print("%.3f HIVE, %.3f HBD" % (HIVE, HBD)) ``` returns ``` duplicate indices 0 6279 entries 65.316 HIVE, 0.063 HBD ``` which is correct according to [hiveblocks](https://hiveblocks.com/@holger80): 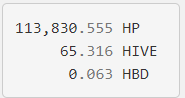 Balance is correct and no duplicate entries were found! ## Entries that look like duplicate account history entries First, I thought there is the same problem on Hive as I was tricked by the following two entries: ``` print(ops_dict[175655]) print(ops_dict[175656]) ``` ``` {'from': 'holger80', 'to': 'beembot', 'amount': {'amount': '1', 'precision': 3, 'nai': '@@000000021'}, 'memo': 'test', 'trx_id': '9b0c144b02d046a18f23639769a8c013bb3fb10a', 'block': 44657346, 'trx_in_block': 9, 'op_in_trx': 0, 'virtual_op': 0, 'timestamp': '2020-06-27T11:50:30', 'account': 'holger80', 'type': 'transfer', '_id': '09fb4d1710c4091f346bcb0fa4e11bfa4128f59c', 'index': 175655} {'from': 'holger80', 'to': 'beembot', 'amount': {'amount': '1', 'precision': 3, 'nai': '@@000000021'}, 'memo': 'test', 'trx_id': '9b0c144b02d046a18f23639769a8c013bb3fb10a', 'block': 44657346, 'trx_in_block': 9, 'op_in_trx': 0, 'virtual_op': 0, 'timestamp': '2020-06-27T11:50:30', 'account': 'holger80', 'type': 'transfer', '_id': '09fb4d1710c4091f346bcb0fa4e11bfa4128f59c', 'index': 175656} ``` which are two tranfers in one transaction: 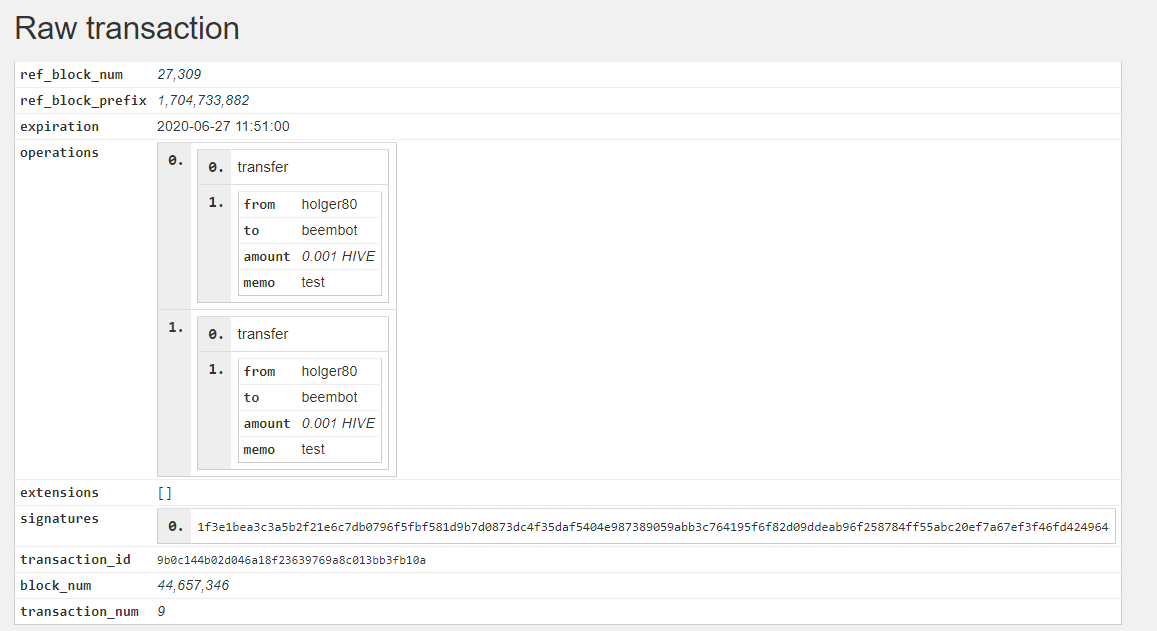 and not a duplicate entry. ``` print(ops_dict[134132]) print(ops_dict[134133]) ``` returns two times the same custom json: ``` {'required_auths': [], 'required_posting_auths': ['holger80'], 'id': 'sm_submit_team', 'json': '{"trx_id":"20b319ed22c3fd0986b3c68d4743f86548db631c","team_hash":"66b00a954a974829cc4965255fbdefb6"}', 'trx_id': 'ca950109641db603ee8ebf6f0a4345180643888b', 'block': 36147842, 'trx_in_block': 23, 'op_in_trx': 0, 'virtual_op': 0, 'timestamp': '2019-09-05T05:41:39', 'account': 'holger80', 'type': 'custom_json', '_id': 'e56596adbea0dd4abe434270ba6f6633e30796d9', 'index': 134132} {'required_auths': [], 'required_posting_auths': ['holger80'], 'id': 'sm_submit_team', 'json': '{"trx_id":"20b319ed22c3fd0986b3c68d4743f86548db631c","team_hash":"66b00a954a974829cc4965255fbdefb6"}', 'trx_id': 'ca950109641db603ee8ebf6f0a4345180643888b', 'block': 36147842, 'trx_in_block': 23, 'op_in_trx': 0, 'virtual_op': 0, 'timestamp': '2019-09-05T05:41:39', 'account': 'holger80', 'type': 'custom_json', '_id': 'e56596adbea0dd4abe434270ba6f6633e30796d9', 'index': 134133} ``` As there are really two identically operations 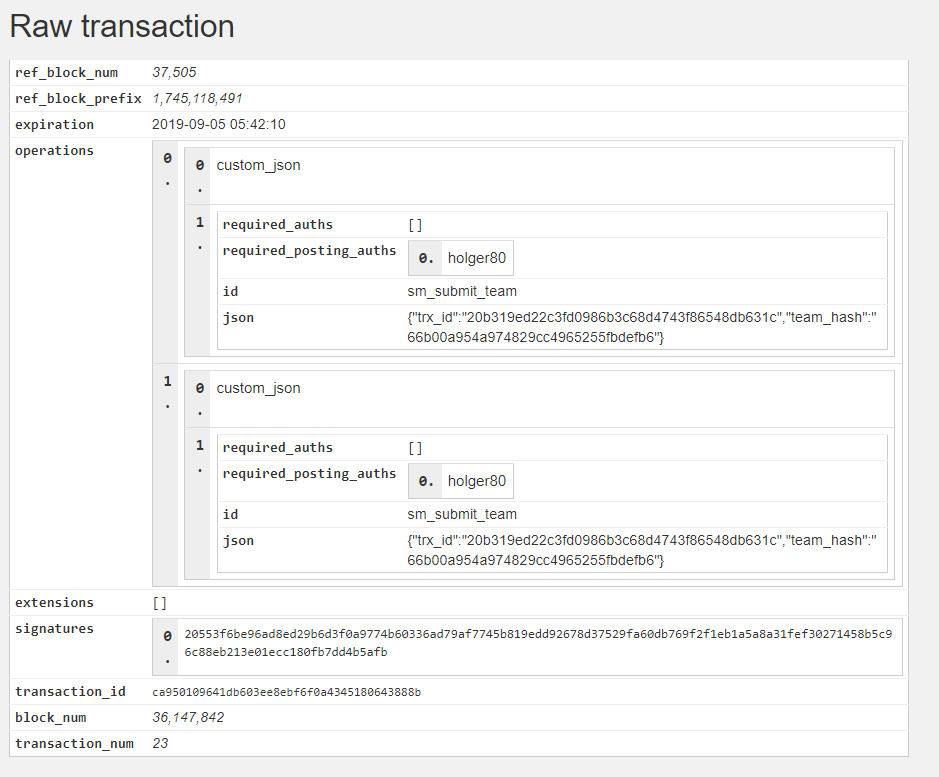 ([hiveblocks](https://hiveblocks.com/tx/ca950109641db603ee8ebf6f0a4345180643888b)), this is also correct. ## Conclusion There is currently a problem on Steem that the account history API returns sometimes duplicate entries. It is possible to identify them and to remove them. The account history API on Hive is working correctly. You can use my scripts to check if your balance can be correctly retraced by the account history api. I will use the scripts and develop them further, so that important account operations can be stored into an excel file. As the Hive source code is still quite similar to the Steem source code, this may also happen on Hive. In order to prevent this, I created an issue: https://gitlab.syncad.com/hive/hive/-/issues/62 so that that this topic can be explored further. ___ *If you like what I do, consider casting a vote for me as witness on [Hivesigner](https://hivesigner.com/sign/account-witness-vote?witness=holger80&approve=1) or on [PeakD](https://peakd.com/witnesses)*
author | holger80 |
---|---|
permlink | duplicate-account-history-entries-on-steem-how-to-detect-and-fix-them |
category | bug |
json_metadata | "{"canonical_url": "https://hive.blog/bug/@holger80/duplicate-account-history-entries-on-steem-how-to-detect-and-fix-them", "app": "beempy/0.24.6", "image": ["https://images.hive.blog/DQmdvjbbHhLMsmmK5nFHwEL97GboHWpeedTA61SpUz3X1Fp/image", "https://images.hive.blog/DQmYRuK8tf3vGoj1XmyDanYRQaqTRZaYKNiqZRojRiq4SLz/my%20steem%20balance", "https://images.hive.blog/DQmervojXHHFqctSdBLsM4mUyU3qBWyzRGgnDFHiv1zhNFS/doublicate%20entry", "https://images.hive.blog/DQmTMP2EtEUzCPLZi2F1Z3oEXsXcP1iLZ8jMfy52wNEs4Z1/image", "https://images.hive.blog/DQmcZVam9XDw2UK9iWRQh5DYXK6me5rMtVsrsgUphLwgefV/block", "https://images.hive.blog/DQmadEb3HfWRaa543vjAsQZLd3MVC5BS6n55eWx7VaVTx47/hive%20balance", "https://images.hive.blog/DQmaZgosWpykMCUsPJQiZVeyo3jRMfkUf737rxAnuLPbkx3/transfers", "https://images.hive.blog/DQmfXduxHRUQLfF4uT2UMu1WR545Xjrz8PEhaQ9mrPdAC1d/not%20a%20duplicate"], "links": ["https://pixabay.com/de/photos/audit-buchhaltung-finanzbuchhaltung-4190945/", "https://steemd.com/@holger80", "https://steemd.com/tx/0a3200e502bd6b1c06b81c2a2337e0436fc13704", "https://steemd.com/b/41868606#0a3200e502bd6b1c06b81c2a2337e0436fc13704", "https://hiveblocks.com/@holger80", "https://hiveblocks.com/tx/ca950109641db603ee8ebf6f0a4345180643888b", "https://gitlab.syncad.com/hive/hive/-/issues/62", "https://hivesigner.com/sign/account-witness-vote?witness=holger80&approve=1", "https://peakd.com/witnesses"], "tags": ["bug", "development", "beem", "python"]}" |
created | 2020-07-29 23:04:03 |
last_update | 2020-07-30 08:19:21 |
depth | 0 |
children | 27 |
last_payout | 2020-08-05 23:04:03 |
cashout_time | 1969-12-31 23:59:59 |
total_payout_value | 43.148 HBD |
curator_payout_value | 31.889 HBD |
pending_payout_value | 0.000 HBD |
promoted | 0.000 HBD |
body_length | 24,102 |
author_reputation | 358,857,509,568,825 |
root_title | "Duplicate account history entries on STEEM: How to detect and fix them" |
beneficiaries | [] |
max_accepted_payout | 1,000,000.000 HBD |
percent_hbd | 10,000 |
post_id | 98,789,828 |
net_rshares | 204,933,324,950,228 |
author_curate_reward | "" |
voter | weight | wgt% | rshares | pct | time |
---|---|---|---|---|---|
fminerten | 0 | 760,907,249,555 | 100% | ||
steempty | 0 | 10,970,428,934,361 | 100% | ||
enki | 0 | 9,475,305,324,888 | 50% | ||
tombstone | 0 | 155,764,506,383 | 7.5% | ||
fractalnode | 0 | 20,793,861,999 | 100% | ||
chitty | 0 | 255,343,297,179 | 75% | ||
onealfa | 0 | 150,370,908,390 | 7.84% | ||
kingscrown | 0 | 1,669,220,514,642 | 20% | ||
flemingfarm | 0 | 169,488,568,709 | 80% | ||
leprechaun | 0 | 6,523,788,588 | 100% | ||
mark-waser | 0 | 492,550,124,395 | 100% | ||
mammasitta | 0 | 170,667,544,073 | 35% | ||
gerber | 0 | 1,220,483,773,979 | 18.8% | ||
daan | 0 | 58,637,876,826 | 8% | ||
ezzy | 0 | 1,459,455,800,083 | 18.8% | ||
field | 0 | 18,358,832,839 | 100% | ||
livingfree | 0 | 261,562,513,933 | 2% | ||
ssjsasha | 0 | 962,135,810,931 | 100% | ||
nascimentoab | 0 | 932,060,925 | 21.99% | ||
arcange | 0 | 3,471,753,300,569 | 100% | ||
exyle | 0 | 1,443,345,996,344 | 18.8% | ||
sharker | 0 | 5,655,770,430 | 13.06% | ||
raphaelle | 0 | 2,655,709,135 | 3% | ||
logic | 0 | 241,269,758,407 | 60% | ||
kibela | 0 | 4,377,944,668 | 13.75% | ||
twinner | 0 | 18,020,604,965,528 | 100% | ||
timcliff | 0 | 1,293,665,392,088 | 100% | ||
laoyao | 0 | 46,037,224,396 | 100% | ||
somebody | 0 | 80,576,559 | 100% | ||
midnightoil | 0 | 162,721,618,801 | 100% | ||
alinalazareva | 0 | 649,682,395 | 10% | ||
xiaohui | 0 | 914,097,985,136 | 100% | ||
jphamer1 | 0 | 9,414,514,203,618 | 100% | ||
joele | 0 | 3,538,722,132,937 | 100% | ||
oflyhigh | 0 | 5,022,564,299,581 | 100% | ||
djennyfloro | 0 | 1,282,122,656 | 10% | ||
fooblic | 0 | 30,945,802,414 | 99% | ||
fingolfin | 0 | 152,953,529,050 | 100% | ||
borran | 0 | 542,847,193,320 | 75% | ||
yulan | 0 | 16,136,736,329 | 100% | ||
anech512 | 0 | 8,887,340,269 | 25% | ||
rubenalexander | 0 | 577,414,818,787 | 100% | ||
helene | 0 | 1,202,517,734,705 | 100% | ||
lemouth | 0 | 1,801,202,636,015 | 100% | ||
stevescoins | 0 | 365,878,226,373 | 100% | ||
someguy123 | 0 | 549,027,921,255 | 28.2% | ||
themonetaryfew | 0 | 762,482,108,224 | 100% | ||
digital-wisdom | 0 | 516,423,455,221 | 100% | ||
ethical-ai | 0 | 1,914,198,641 | 100% | ||
jwaser | 0 | 22,367,383,014 | 100% | ||
petrvl | 0 | 58,606,519,623 | 7.5% | ||
bwaser | 0 | 11,818,728,136 | 100% | ||
abh12345 | 0 | 774,761,816,635 | 25% | ||
whatsup | 0 | 578,578,100,358 | 100% | ||
funnyman | 0 | 3,832,124,346 | 20% | ||
steemcleaners | 0 | 3,513,924,174,300 | 60% | ||
justyy | 0 | 81,500,237,645 | 10% | ||
herpetologyguy | 0 | 154,645,604,363 | 100% | ||
morgan.waser | 0 | 24,184,359,189 | 100% | ||
ssekulji | 0 | 16,372,244,014 | 100% | ||
handyman | 0 | 2,022,490,222 | 100% | ||
strong-ai | 0 | 1,938,121,912 | 100% | ||
rishi556 | 0 | 11,367,841,093 | 100% | ||
busy.pay | 0 | 276,097,017,457 | 100% | ||
gamer00 | 0 | 67,900,256,444 | 5% | ||
techslut | 0 | 106,467,716,338 | 20% | ||
triviummethod | 0 | 553,845,981 | 100% | ||
slider2990 | 0 | 54,270,782,231 | 25% | ||
created | 0 | 336,752,105,068 | 2% | ||
felix.herrmann | 0 | 19,073,165,107 | 20% | ||
edb | 0 | 53,257,645,536 | 50% | ||
busy.org | 0 | 348,261,922,462 | 100% | ||
technoprogressiv | 0 | 970,237,557 | 100% | ||
saleg25 | 0 | 11,056,389,198 | 100% | ||
em3di | 0 | 2,368,696,998 | 10.99% | ||
askari | 0 | 55,872,204,645 | 100% | ||
blackbunny | 0 | 72,264,630,327 | 100% | ||
da-dawn | 0 | 36,327,625,163 | 23% | ||
lingfei | 0 | 79,603,994,514 | 100% | ||
tarazkp | 0 | 2,348,701,251,937 | 50% | ||
steemitboard | 0 | 2,638,889,816 | 2% | ||
privex | 0 | 34,878,720,035 | 37.6% | ||
sudutpandang | 0 | 7,483,252,131 | 100% | ||
markkujantunen | 0 | 105,229,390,955 | 100% | ||
tabea | 0 | 39,491,791,136 | 30% | ||
danielsaori | 0 | 133,498,778,860 | 100% | ||
louisthomas | 0 | 116,308,326,000 | 100% | ||
dhimmel | 0 | 5,264,140,364,440 | 100% | ||
freebornsociety | 0 | 1,848,884,013 | 10% | ||
elevator09 | 0 | 238,517,677,266 | 100% | ||
lizanomadsoul | 0 | 298,965,543,306 | 100% | ||
dune69 | 0 | 76,486,807,534 | 18.8% | ||
frankydoodle | 0 | 5,540,673,827 | 50% | ||
broncnutz | 0 | 8,146,387,830,073 | 100% | ||
bobskibob | 0 | 71,150,285,269 | 50% | ||
forykw | 0 | 236,292,222,627 | 100% | ||
jerrybanfield | 0 | 1,004,063,607,091 | 100% | ||
rocksg | 0 | 168,748,434,585 | 100% | ||
roomservice | 0 | 785,251,989,686 | 18.75% | ||
mys | 0 | 4,952,370,380 | 1.88% | ||
steempearls | 0 | 90,563,297 | 15% | ||
exec | 0 | 243,817,644,838 | 100% | ||
eval | 0 | 825,809,458 | 100% | ||
mxzn | 0 | 1,756,847,466 | 10% | ||
jeanpi1908 | 0 | 53,549,361,959 | 50% | ||
belahejna | 0 | 10,177,735,456 | 7.5% | ||
giuatt07 | 0 | 284,039,211,542 | 25% | ||
lelon | 0 | 12,645,533,137 | 100% | ||
skepticology | 0 | 1,577,926,931 | 100% | ||
enjar | 0 | 553,153,815,092 | 100% | ||
maxer27 | 0 | 136,461,791,159 | 25% | ||
tracer-paulo | 0 | 5,423,857,629 | 100% | ||
blacklux | 0 | 33,230,417,049 | 100% | ||
sam99 | 0 | 12,198,502,839 | 10% | ||
jingdol | 0 | 481,264,678,404 | 100% | ||
ew-and-patterns | 0 | 213,274,283,394 | 9% | ||
techken | 0 | 1,492,797,254 | 2.5% | ||
whd | 0 | 2,505,103,189 | 1.88% | ||
offgridlife | 0 | 572,784,959,187 | 100% | ||
benedict08 | 0 | 172,655,202,612 | 50% | ||
mcoinz79 | 0 | 64,803,251,299 | 100% | ||
bubke | 0 | 2,644,244,621,290 | 100% | ||
maskur2840 | 0 | 11,431,866,812 | 100% | ||
jacekw | 0 | 56,502,904,145 | 100% | ||
drorion | 0 | 13,972,355,007 | 100% | ||
haejin | 0 | 12,791,608,660,934 | 100% | ||
andre-verbrick | 0 | 45,358,650,552 | 100% | ||
codingdefined | 0 | 316,785,202,609 | 100% | ||
hope-on-fire | 0 | 232,796,026,470 | 26% | ||
nicniezgrublem | 0 | 726,437,251 | 17.86% | ||
synrg | 0 | 373,176,353,297 | 100% | ||
shitsignals | 0 | 7,849,216,956 | 18.8% | ||
attajuttjj | 0 | 3,380,202,551 | 100% | ||
dine77 | 0 | 7,012,788,660 | 8% | ||
tykee | 0 | 3,380,482,061 | 50% | ||
yoogyart | 0 | 39,328,045,082 | 50% | ||
bronkong | 0 | 365,388,195,592 | 100% | ||
vikisecrets | 0 | 363,869,207,054 | 30% | ||
khalil319 | 0 | 941,736,003 | 50% | ||
onetin84 | 0 | 938,682,372,105 | 100% | ||
felander | 0 | 97,990,309,652 | 18.8% | ||
santigs | 0 | 26,873,316,349 | 65% | ||
bashadow | 0 | 53,649,427,359 | 25% | ||
tipu | 0 | 7,915,365,470,060 | 20.01% | ||
kimzwarch | 0 | 9,118,456,088 | 4% | ||
jedigeiss | 0 | 1,311,077,864,179 | 100% | ||
massivevibration | 0 | 27,394,373,033 | 15% | ||
onartbali | 0 | 10,701,873,029 | 50% | ||
crokkon | 0 | 27,372,501,629 | 100% | ||
fbslo | 0 | 3,887,568,686 | 0.94% | ||
accelerator | 0 | 46,805,842,380 | 5% | ||
yogacoach | 0 | 8,767,217,711 | 9.4% | ||
therealwolf | 0 | 6,874,349,363,378 | 25% | ||
robertoueti | 0 | 1,592,728,666 | 3% | ||
deathwing | 0 | 20,739,931,449 | 18.8% | ||
revisesociology | 0 | 348,422,344,524 | 30% | ||
superbing | 0 | 574,331,794 | 90% | ||
isnochys | 0 | 29,846,534,359 | 18% | ||
gringo211985 | 0 | 2,788,802,925 | 100% | ||
shadflyfilms | 0 | 9,289,382,767 | 100% | ||
pitboy | 0 | 54,847,627,001 | 100% | ||
daisyphotography | 0 | 44,340,740,102 | 100% | ||
investegg | 0 | 127,585,426,479 | 2.83% | ||
liverpool-fan | 0 | 1,075,987,498 | 10% | ||
arabisouri | 0 | 51,986,730,299 | 100% | ||
mrsyria | 0 | 988,674,732 | 100% | ||
joseph1956 | 0 | 18,852,901,525 | 100% | ||
tradingideas | 0 | 13,250,559,380 | 100% | ||
kipling | 0 | 548,323,294 | 100% | ||
jexus77 | 0 | 1,496,139,712 | 100% | ||
quekery | 0 | 142,479,642,922 | 100% | ||
omstavan | 0 | 7,890,495,985 | 100% | ||
emrebeyler | 0 | 385,564,126,749 | 18.8% | ||
smartsteem | 0 | 1,446,757,049,862 | 25% | ||
shaotech | 0 | 247,747,388 | 100% | ||
banner8888 | 0 | 320,915,969,735 | 100% | ||
sarakey | 0 | 12,095,102,537 | 100% | ||
anli | 0 | 6,282,061,035 | 99% | ||
andrepol | 0 | 5,542,190,429 | 99% | ||
citizensmith | 0 | 128,521,472,147 | 18.8% | ||
mytechtrail | 0 | 39,196,668,518 | 15% | ||
lebin | 0 | 197,895,163,285 | 100% | ||
cloris | 0 | 1,226,763,527 | 100% | ||
itchyfeetdonica | 0 | 13,987,743,590 | 30% | ||
funtraveller | 0 | 3,729,733,733 | 18.8% | ||
nokodemion | 0 | 23,311,980,964 | 100% | ||
kamchore | 0 | 132,037,063,629 | 100% | ||
smooms | 0 | 42,294,397,423 | 100% | ||
steembasicincome | 0 | 2,544,005,015,254 | 100% | ||
jpphotography | 0 | 4,424,352,960 | 36.64% | ||
wiseagent | 0 | 102,196,987,807 | 21.99% | ||
fourfourfun | 0 | 6,818,462,972 | 25% | ||
candyboy | 0 | 5,381,660,501 | 100% | ||
jim888 | 0 | 248,457,798,559 | 22% | ||
phortun | 0 | 217,722,670,203 | 30% | ||
abitcoinskeptic | 0 | 45,475,232,792 | 20% | ||
kissi | 0 | 14,385,789,005 | 75% | ||
jewel-lover | 0 | 1,650,470,943 | 100% | ||
jongolson | 0 | 468,015,326,870 | 50% | ||
nerdtopiade | 0 | 22,286,147,645 | 65% | ||
bartheek | 0 | 28,824,331,852 | 10% | ||
slashformotion | 0 | 476,729,695 | 100% | ||
payger | 0 | 5,590,362,021 | 50% | ||
korinkrafting | 0 | 804,167,080 | 22.5% | ||
matheusggr | 0 | 44,374,320,337 | 21.99% | ||
nealmcspadden | 0 | 383,123,303,826 | 18.8% | ||
bala41288 | 0 | 41,164,745,151 | 20% | ||
socialmediaseo | 0 | 857,558,159 | 50% | ||
mermaidvampire | 0 | 13,234,786,385 | 45% | ||
afiqsejuk | 0 | 549,876,336,350 | 100% | ||
ahmedsy | 0 | 2,582,495,866 | 100% | ||
manncpt | 0 | 62,592,797,309 | 100% | ||
purefood | 0 | 302,091,409,237 | 18.8% | ||
chrismadcboy2016 | 0 | 27,023,287,383 | 100% | ||
jimcustodio | 0 | 1,803,687,378 | 50% | ||
tmarisco | 0 | 1,455,739,745 | 21.99% | ||
vaansteam | 0 | 2,330,072,390 | 30% | ||
jnmarteau | 0 | 23,151,901,445 | 100% | ||
casberp | 0 | 30,699,550,384 | 21.99% | ||
philnewton | 0 | 2,929,587,216 | 37.5% | ||
tobias-g | 0 | 11,790,572,810 | 18.75% | ||
chronocrypto | 0 | 185,434,029,679 | 18.8% | ||
nobyeni | 0 | 2,506,886,571 | 5% | ||
holger80 | 0 | 3,284,429,189,634 | 75% | ||
peeyush | 0 | 0 | 4% | ||
unconditionalove | 0 | 1,974,216,211 | 9.4% | ||
minerspost | 0 | 1,578,158,731 | 50% | ||
ericburgoyne | 0 | 3,618,678,634 | 25% | ||
sweetkathy | 0 | 2,192,087,807 | 100% | ||
sudefteri | 0 | 21,326,620,573 | 100% | ||
happy-soul | 0 | 91,392,193,188 | 10% | ||
fireguardian | 0 | 577,834,744 | 21.99% | ||
pkocjan | 0 | 8,071,915,895 | 15.04% | ||
maxpatternman | 0 | 26,470,549,428 | 100% | ||
condeas | 0 | 1,321,083,428,272 | 100% | ||
anikys3reasure | 0 | 2,735,990,864 | 50% | ||
darkpylon | 0 | 2,904,046,389 | 100% | ||
barge | 0 | 242,495,585,426 | 100% | ||
onlavu | 0 | 4,419,723,556 | 15% | ||
asgarth | 0 | 2,420,062,424,945 | 77% | ||
flugschwein | 0 | 32,934,633,190 | 100% | ||
hasmez | 0 | 95,795,156,461 | 100% | ||
cst90 | 0 | 56,445,111,681 | 100% | ||
matschi | 0 | 3,589,115,717 | 100% | ||
memesdaily | 0 | 7,303,451,305 | 100% | ||
jjal | 0 | 1,061,239,907 | 100% | ||
whack.science | 0 | 131,937,170,903 | 35% | ||
akifane | 0 | 3,699,845,847 | 100% | ||
russellstockley | 0 | 2,133,734,430 | 10% | ||
futurecurrency | 0 | 19,986,993,209 | 34% | ||
siphon | 0 | 13,106,138,433 | 30% | ||
losi | 0 | 5,456,307,075 | 100% | ||
dunkman | 0 | 862,027,912 | 76% | ||
leeyh | 0 | 510,118,137,082 | 100% | ||
backinblackdevil | 0 | 648,044,053,644 | 75% | ||
oadissin | 0 | 31,763,762,103 | 100% | ||
properfraction | 0 | 42,940,912,185 | 100% | ||
erikah | 0 | 211,568,610,235 | 25% | ||
satren | 0 | 92,388,740,012 | 30% | ||
lauchmelder | 0 | 4,909,880,842 | 100% | ||
kargul09 | 0 | 7,300,846,701 | 100% | ||
amico | 0 | 960,009,930,100 | 97.69% | ||
beleg | 0 | 824,557,916 | 1.88% | ||
marcus0alameda | 0 | 937,873,970 | 50% | ||
andablackwidow | 0 | 11,864,197,608 | 100% | ||
tiababi | 0 | 447,699,464 | 100% | ||
bestboom | 0 | 75,820,011,041 | 18.8% | ||
eunsik | 0 | 293,844,490,842 | 50% | ||
onepercentbetter | 0 | 160,437,988,514 | 100% | ||
dotwin1981 | 0 | 38,650,411,806 | 20% | ||
ronaldoavelino | 0 | 157,924,082,965 | 40% | ||
lesmouths-travel | 0 | 8,103,105,431 | 100% | ||
shawmeow | 0 | 5,528,798,898 | 82% | ||
sbi2 | 0 | 1,846,770,388,539 | 100% | ||
dera123 | 0 | 1,194,607,409,871 | 100% | ||
altobee | 0 | 61,480,678,713 | 65% | ||
elleok | 0 | 3,892,773,861 | 100% | ||
freddio | 0 | 13,398,041,314 | 15% | ||
blainjones | 0 | 1,379,570,935 | 7.5% | ||
alitavirgen | 0 | 484,828,363 | 15.4% | ||
gadrian | 0 | 160,418,074,496 | 100% | ||
themightyvolcano | 0 | 27,102,028,930 | 18.8% | ||
iseeyouvee | 0 | 1,134,347,835 | 18.8% | ||
ericahan | 0 | 337,590,932 | 100% | ||
dreimaldad | 0 | 83,623,669,705 | 40% | ||
quochuy | 0 | 332,949,208,760 | 25.03% | ||
aiyumi | 0 | 5,614,167,832 | 21.99% | ||
saboin | 0 | 99,785,107,809 | 11% | ||
ifunnymemes | 0 | 5,040,946,152 | 9.4% | ||
stefannikolov | 0 | 993,206,348 | 10% | ||
globalschool | 0 | 40,938,994,638 | 50% | ||
morellys2004 | 0 | 12,230,159,410 | 100% | ||
sbi3 | 0 | 1,296,933,749,444 | 100% | ||
gagago | 0 | 10,125,088,626 | 100% | ||
promobot | 0 | 90,964,292,182 | 100% | ||
superlao | 0 | 21,012,474,408 | 100% | ||
hranhuk | 0 | 7,222,957,325 | 40% | ||
glodniwiedzy | 0 | 5,334,060,489 | 17.86% | ||
sbi4 | 0 | 944,749,976,913 | 100% | ||
opt2o | 0 | 2,867,075,046 | 50% | ||
fw206 | 0 | 1,094,742,065,633 | 100% | ||
mynotsofitlife | 0 | 1,059,029,754 | 10% | ||
slobberchops | 0 | 2,418,541,217,711 | 50% | ||
atanas007 | 0 | 8,656,050,154 | 100% | ||
hatoto | 0 | 166,722,210,935 | 48% | ||
carlpei | 0 | 311,571,351,794 | 100% | ||
meins0815 | 0 | 8,221,124,924 | 23% | ||
blockchainstudio | 0 | 5,214,006,314 | 100% | ||
crimo | 0 | 711,435,958 | 11.5% | ||
eraizel | 0 | 684,194,926 | 21.99% | ||
davidesimoncini | 0 | 8,642,053,938 | 25% | ||
crypticat | 0 | 5,515,867,472 | 100% | ||
archisteem | 0 | 2,558,806,386 | 7.5% | ||
spamfarmer | 0 | 1,117,852,129 | 25% | ||
linnyplant | 0 | 43,336,190,651 | 100% | ||
commonlaw | 0 | 4,482,407,284 | 35% | ||
cryptoandcoffee | 0 | 588,060,454,977 | 50% | ||
bluewall | 0 | 30,269,790,523 | 100% | ||
corinadiaz | 0 | 19,541,499,854 | 100% | ||
solarwarrior | 0 | 1,325,601,835,505 | 100% | ||
merlion | 0 | 36,471,232,306 | 100% | ||
alejandra.her | 0 | 79,275,388,328 | 100% | ||
simplegame | 0 | 122,660,382,533 | 100% | ||
sbi5 | 0 | 691,211,333,094 | 100% | ||
swisswitness | 0 | 11,066,362,698 | 18.8% | ||
kahvesizlik | 0 | 1,265,656,728 | 100% | ||
inteligente | 0 | 30,147,653 | 21.99% | ||
moneybaby | 0 | 816,219,841 | 2.5% | ||
imtase | 0 | 515,323,679 | 65% | ||
longer | 0 | 2,414,062,428 | 5% | ||
sbi6 | 0 | 505,163,541,851 | 100% | ||
libuska | 0 | 1,971,364,799 | 100% | ||
drsensor | 0 | 2,213,652,738 | 80% | ||
pizzajohn | 0 | 630,859,364 | 50% | ||
urdreamscometrue | 0 | 25,377,885,575 | 100% | ||
gallerani | 0 | 1,157,016,575 | 18.8% | ||
pagliozzo | 0 | 354,454,855 | 20% | ||
izzynoel | 0 | 844,678,603 | 50% | ||
sonder-an | 0 | 366,954,828 | 100% | ||
akioexzgamer | 0 | 15,667,860 | 65% | ||
gerdtrudroepke | 0 | 25,508,959,551 | 50% | ||
daath | 0 | 1,735,153,514 | 100% | ||
teamvn | 0 | 33,254,666,505 | 50.03% | ||
dalz | 0 | 27,086,304,597 | 9.4% | ||
smartvote | 0 | 177,550,034,769 | 6% | ||
deividluchi | 0 | 1,013,527,687 | 21.99% | ||
yuza | 0 | 1,013,749,095 | 65% | ||
pvinny69 | 0 | 6,905,816,973 | 25% | ||
sbi7 | 0 | 350,834,022,094 | 100% | ||
julian2013 | 0 | 798,120,008 | 0.84% | ||
dlike | 0 | 227,546,938,071 | 18.8% | ||
triptolemus | 0 | 1,765,524,809 | 18.8% | ||
cryptoyzzy | 0 | 110,567,985,286 | 100% | ||
abduljalil.mbo | 0 | 16,531,351,699 | 100% | ||
gorbisan | 0 | 883,945,910 | 0.94% | ||
ataliba | 0 | 5,193,806,735 | 40% | ||
fullnodeupdate | 0 | 20,923,553,149 | 75% | ||
ten-years-before | 0 | 117,344,589 | 65% | ||
engrave | 0 | 250,185,078,100 | 17.86% | ||
altonos | 0 | 32,948,730,893 | 25% | ||
tipsybosphorus | 0 | 467,020,179,425 | 100% | ||
furioslosi | 0 | 1,459,915,579 | 100% | ||
kr-coffeesteem | 0 | 48,592,550,397 | 100% | ||
a-bot | 0 | 34,971,051,645 | 100% | ||
bobby.madagascar | 0 | 6,508,213,235 | 4.7% | ||
laissez-faire | 0 | 42,391,695 | 100% | ||
voter003 | 0 | 127,126,881,854 | 69.83% | ||
muscara | 0 | 32,646,280,785 | 20% | ||
cultus-forex | 0 | 81,057,538,437 | 100% | ||
quediceharry | 0 | 13,094,682,903 | 100% | ||
musinka | 0 | 1,932,196,320 | 100% | ||
puza | 0 | 828,618,424 | 65% | ||
crypto.story | 0 | 57,949,702 | 65% | ||
sbi8 | 0 | 260,927,764,304 | 100% | ||
gamer0815 | 0 | 774,707,447 | 20% | ||
univers.crypto | 0 | 184,318,953 | 65% | ||
mintrawa | 0 | 954,697,588 | 65% | ||
ldp | 0 | 2,996,298,517 | 18.8% | ||
steemitcuration | 0 | 1,774,824,036 | 25% | ||
sbi9 | 0 | 165,263,785,208 | 100% | ||
actifit-peter | 0 | 433,445,225,805 | 99.06% | ||
curart38 | 0 | 11,499,300,651 | 100% | ||
besheda | 0 | 1,062,284,181 | 43% | ||
diegor | 0 | 31,144,119,768 | 100% | ||
thrasher666 | 0 | 1,701,755,863 | 60% | ||
everyoung | 0 | 710,158,972 | 100% | ||
florino | 0 | 810,572,157 | 15% | ||
linuxbot | 0 | 657,614,955 | 100% | ||
forecasteem | 0 | 99,675,726,375 | 100% | ||
eliasseth | 0 | 5,318,236,337 | 100% | ||
geeklania | 0 | 11,072,774,199 | 100% | ||
followjohngalt | 0 | 107,319,067,644 | 18.8% | ||
mistia | 0 | 6,551,905,079 | 100% | ||
glastar | 0 | 1,490,414,501,392 | 100% | ||
sbi10 | 0 | 158,481,849,680 | 100% | ||
dein-problem | 0 | -78,815,199 | -0.75% | ||
moneytron | 0 | 15,071,690,241 | 100% | ||
starrouge | 0 | 609,095,514 | 30% | ||
wherein | 0 | 394,364,190,267 | 60% | ||
shainemata | 0 | 15,597,588,782 | 20% | ||
zerofive | 0 | 571,245,399 | 30% | ||
j-p-bs | 0 | 1,010,553,274 | 50% | ||
thehealthylife | 0 | 3,854,583,302 | 100% | ||
smon-fan | 0 | 2,005,719,029 | 100% | ||
jacuzzi | 0 | 4,131,222,462 | 10% | ||
kaldewei | 0 | 4,026,045,467 | 20% | ||
riyuuhi | 0 | 0 | 100% | ||
steemillu | 0 | 38,390,656,975 | 100% | ||
smoner | 0 | 1,443,689,763 | 100% | ||
flyingbolt | 0 | 1,395,238,546 | 18.8% | ||
determine | 0 | 1,128,782,438 | 18.8% | ||
cnstm | 0 | 183,941,691,109 | 60% | ||
tonalddrump | 0 | 1,340,559,694 | 50% | ||
permaculturedude | 0 | 3,746,781,408 | 9.4% | ||
agent14 | 0 | 29,393,563,215 | 35% | ||
meins0816 | 0 | 2,681,525,624 | 75% | ||
jamesbattler | 0 | 133,582,941,942 | 100% | ||
grimgriz | 0 | 0 | 100% | ||
smon-joa | 0 | 17,549,091,445 | 100% | ||
lianjingmedia | 0 | 582,403,305 | 60% | ||
broxi | 0 | 9,932,020,038 | 50% | ||
curationvoter | 0 | 22,634,238,588 | 50% | ||
curationhelper | 0 | 23,203,663,288 | 100% | ||
redaktion | 0 | 1,018,432,184 | 100% | ||
realgoodcontent | 0 | 963,229,631 | 100% | ||
richie.rich | 0 | 8,684,325,390 | 100% | ||
hungrybear | 0 | 3,480,837,132 | 100% | ||
hamsa.quality | 0 | 6,499,537,008 | 100% | ||
dcommerce | 0 | 898,697,005,368 | 100% | ||
abbenay | 0 | 10,904,363,022 | 50% | ||
sm-skynet | 0 | 898,433,927 | 100% | ||
smonbear | 0 | 1,691,492,266 | 100% | ||
denizcakmak | 0 | 536,360,578 | 50% | ||
hungryharish | 0 | 1,318,782,998 | 4.7% | ||
cpt-sparrow | 0 | 9,482,020,614 | 100% | ||
newsticker | 0 | 908,673,930 | 100% | ||
witcher73 | 0 | 1,454,366,967 | 100% | ||
marymi | 0 | 480,124,706 | 100% | ||
ttg | 0 | 379,477,974,037 | 100% | ||
triple-paradox | 0 | 140,298,072 | 100% | ||
kryptogames | 0 | 91,473,817,893 | 20.01% | ||
mfblack | 0 | 14,658,550,271 | 17.86% | ||
steemcartel | 0 | 7,392,891,191 | 100% | ||
szf | 0 | 965,220,369 | 50% | ||
blue.rabbit | 0 | 719,607,943,914 | 100% | ||
actifit-devil | 0 | 17,185,822,100 | 100% | ||
samujaeger | 0 | 32,885,727,095 | 100% | ||
epicdice | 0 | 55,033,954,839 | 7.5% | ||
battlegames | 0 | 420,780,567,934 | 99% | ||
raspibot | 0 | 3,745,087,924 | 100% | ||
blockchainpeople | 0 | 116,053,025 | 21.99% | ||
gadrian-sp | 0 | 1,391,553,160 | 100% | ||
helgalubevi | 0 | 3,773,178,676 | 15% | ||
minigame | 0 | 1,053,866,720,294 | 100% | ||
monsterjamgold | 0 | 149,439,411,968 | 100% | ||
gulf41 | 0 | 6,185,584,467 | 100% | ||
sm-silva | 0 | 2,160,102,004 | 9.4% | ||
naltedtirt | 0 | 1,970,245,973 | 50% | ||
steementertainer | 0 | 7,396,865,447 | 65% | ||
stickstore | 0 | 855,336,768 | 100% | ||
likwid | 0 | 9,994,117,824,322 | 100% | ||
swedishdragon76 | 0 | 2,488,827,304 | 50% | ||
dachcolony | 0 | 18,831,624,350 | 100% | ||
tradingideas2 | 0 | 195,223,619 | 100% | ||
plankton.token | 0 | 27,841,005,818 | 30% | ||
captain.kirk | 0 | 4,403,898,980 | 50% | ||
iamjohn | 0 | 1,504,337,204 | 25% | ||
firefuture | 0 | 1,016,424,524 | 18.8% | ||
ilovecanada | 0 | 1,023,781,364 | 50% | ||
steemindian | 0 | 1,782,606,289 | 9.4% | ||
nalexadre | 0 | 300,021,312 | 65% | ||
cryptogambit | 0 | 1,444,869,138 | 7.5% | ||
tomoyan | 0 | 115,891,327,296 | 100% | ||
restcity | 0 | 0 | 100% | ||
imbartley | 0 | 1,572,605,779 | 50% | ||
leeyh3 | 0 | 1,840,680,366 | 50% | ||
shimozurdo | 0 | 23,482,039,087 | 100% | ||
milu-the-dog | 0 | 8,266,992,670 | 18.8% | ||
lrekt01 | 0 | 2,454,500,248 | 100% | ||
triplea.bot | 0 | 6,903,840,818 | 18.8% | ||
steem.leo | 0 | 495,212,515,968 | 18.8% | ||
condeas.pal | 0 | 837,884,304 | 90% | ||
holycow2019 | 0 | 287,121,509 | 100% | ||
votebetting | 0 | 616,232,634,831 | 50% | ||
scotauto | 0 | 4,113,062,463 | 100% | ||
freddio.sport | 0 | 3,837,226,242 | 15% | ||
zaku-pal | 0 | 665,361,795 | 18.8% | ||
zaku-leo | 0 | 687,668,515 | 18.8% | ||
babytarazkp | 0 | 3,287,543,189 | 50% | ||
finex | 0 | 901,414,155 | 100% | ||
asteroids | 0 | 92,055,950,335 | 16.92% | ||
mapxv | 0 | 4,342,827,466 | 29% | ||
acguitar1 | 0 | 0 | 100% | ||
ticketyboo | 0 | 12,892,444,853 | 100% | ||
ticketywoof | 0 | 12,890,628,021 | 100% | ||
happiness19 | 0 | 411,432,933 | 100% | ||
sbi-tokens | 0 | 13,973,419,139 | 35.19% | ||
one.life | 0 | 2,383,269,588 | 18.76% | ||
re2pair | 0 | 249,909,616 | 100% | ||
maxuvv | 0 | 288,690,260 | 9% | ||
maxuvb | 0 | 1,506,081,272 | 70% | ||
maxuvd | 0 | 20,635,701,312 | 6% | ||
maxuve | 0 | 27,986,965,464 | 6% | ||
dappcoder | 0 | 2,818,907,994 | 33% | ||
btscn | 0 | 493,314,917,024 | 100% | ||
borbina | 0 | 12,828,688,865 | 100% | ||
dnflsms | 0 | 1,631,503,039 | 100% | ||
ctl001 | 0 | 597,123,744 | 100% | ||
bcm | 0 | 15,020,466,696 | 15% | ||
bilpcoinrecords | 0 | 613,401,307 | 12.5% | ||
abh12345.medi | 0 | 40,032,059 | 25% | ||
dappstats | 0 | 3,639,961,578 | 15% | ||
bigbr | 0 | 805,241,170 | 17.59% | ||
therealyme | 0 | 1,172,361,565 | 15.04% | ||
blocktvnews | 0 | 1,165,793,884 | 9.4% | ||
kleingeldpilotin | 0 | 3,470,842,865 | 100% | ||
steemillu.random | 0 | 531,434,685 | 100% | ||
qwertm | 0 | 3,106,508,066 | 50% | ||
keep-keep | 0 | 243,717,272 | 100% | ||
huaren.news | 0 | 114,226,471,762 | 12% | ||
detetive | 0 | 26,149,246 | 21.99% | ||
gerbo | 0 | 192,168,949 | 18.8% | ||
kryptoformator | 0 | 3,491,901,593 | 3.75% | ||
hjmarseille | 0 | 13,076,870,999 | 100% | ||
curationstudio | 0 | 5,831,687,307 | 100% | ||
ribary | 0 | 6,217,190,094 | 9.4% | ||
bilpcoinbpc | 0 | 880,409,977 | 10% | ||
mice-k | 0 | 81,809,353,435 | 18.8% | ||
bela29 | 0 | 300,243,990 | 100% | ||
staryao | 0 | 3,971,750,068 | 30% | ||
cellonoob | 0 | 3,534,096,310 | 100% | ||
curamax | 0 | 8,031,934,798 | 18.8% | ||
waqasrizvi | 0 | 1,806,372,091 | 100% | ||
bib15hash | 0 | 803,300,132 | 100% | ||
mehmetfix | 0 | 21,518,392,497 | 100% | ||
sujaytechnicals | 0 | 54,443,578 | 100% | ||
steemcityrewards | 0 | 2,664,417,582 | 18.8% | ||
hive-127039 | 0 | 1,908,862,912 | 50% | ||
dpend.active | 0 | 6,349,861,032 | 3.76% | ||
aboshliz | 0 | 0 | 100% | ||
shinoxl | 0 | 11,055,806,863 | 100% | ||
dapplr | 0 | 34,426,772,318 | 100% | ||
stuntman.mike | 0 | 48,056,199,694 | 100% | ||
fengchao | 0 | 1,072,977,938 | 1% | ||
artdescry | 0 | 1,066,999,999 | 18.8% | ||
hivewatchers | 0 | 226,607,371,860 | 65% | ||
hellohive | 0 | 1,086,550,102,576 | 100% | ||
mountaingod | 0 | 390,604,872,381 | 100% | ||
hiveyoda | 0 | 6,900,836,637 | 2% | ||
hiq | 0 | 76,391,113,269 | 100% | ||
folklure | 0 | 1,492,100,021 | 9.4% | ||
nulledgh0st | 0 | 1,155,591,288,867 | 50% | ||
gitplait | 0 | 60,028,622,687 | 100% | ||
softworld | 0 | 717,072,164,854 | 51% | ||
polish.hive | 0 | 50,660,701,679 | 18.8% | ||
quello | 0 | 136,821,808,550 | 18.75% | ||
sadvil | 0 | 2,097,119,907 | 82% | ||
dcityrewards | 0 | 423,889,922,663 | 18.8% | ||
freakeao | 0 | 15,006,790,303 | 100% | ||
feiderman | 0 | 10,219,835,892 | 100% | ||
hextech | 0 | 3,845,035,158 | 66% | ||
sketching | 0 | 946,725,370 | 9.4% | ||
hiveonboard | 0 | 16,127,106,706 | 18.75% | ||
hivelist | 0 | 16,824,825,277 | 9.4% | ||
ninnu | 0 | 7,003,049,899 | 15% | ||
traduzindojogos | 0 | 1,810,721,769 | 100% | ||
wlslink | 0 | 5,566,585,721 | 100% | ||
waytolifecare | 0 | 0 | 100% | ||
raven.icu | 0 | 2,563,954,640 | 100% | ||
chicovieira09 | 0 | 0 | 100% | ||
ayhamyou | 0 | 1,892,088,731 | 100% | ||
ronavel | 0 | 237,845,299,610 | 15% | ||
hivecur | 0 | 472,228,636,928 | 18.8% | ||
patronpass | 0 | 2,231,831,196 | 100% | ||
greentealover | 0 | 1,536,974,654 | 100% | ||
plusvault | 0 | 1,960,022,530 | 100% | ||
hivebuilderteam | 0 | 2,199,072,463 | 25% | ||
mutabor78 | 0 | 0 | 100% | ||
chartreader | 0 | 139,968,695,453 | 18.8% | ||
hivecur2 | 0 | 7,771,964,498 | 20% | ||
yousep20 | 0 | 0 | 100% | ||
recoveryinc | 0 | 1,806,338,559,199 | 100% | ||
qdolphin | 0 | 1,550,000,000 | 100% | ||
gladysgarcia | 0 | 0 | 100% | ||
hive-108278 | 0 | 3,044,253,797 | 9.37% | ||
unknowngamer | 0 | 0 | 100% | ||
cryptosimplify | 0 | 512,969,451 | 100% | ||
herm3s | 0 | 1,112,297,574 | 100% | ||
marionaem | 0 | 0 | 100% | ||
larrycompany | 0 | 66,268,128 | 100% | ||
mfumid001 | 0 | 0 | 100% | ||
blazing07 | 0 | 0 | 100% | ||
trangtran23 | 0 | 0 | 100% | ||
mystic0601 | 0 | 0 | 100% |
Great jobs đ
author | ayhamyou |
---|---|
permlink | qe99ei |
category | bug |
json_metadata | {"app":"hiveblog/0.1"} |
created | 2020-07-30 00:01:30 |
last_update | 2020-07-30 00:01:30 |
depth | 1 |
children | 1 |
last_payout | 2020-08-06 00:01:30 |
cashout_time | 1969-12-31 23:59:59 |
total_payout_value | 0.000 HBD |
curator_payout_value | 0.000 HBD |
pending_payout_value | 0.000 HBD |
promoted | 0.000 HBD |
body_length | 12 |
author_reputation | 773,684,538,220 |
root_title | "Duplicate account history entries on STEEM: How to detect and fix them" |
beneficiaries | [] |
max_accepted_payout | 1,000,000.000 HBD |
percent_hbd | 10,000 |
post_id | 98,790,502 |
net_rshares | 0 |
[Source](https://hive.blog/@ayhamyou/comments) Spamming comments is frowned upon by the community. Continued comment spamming may result in the account being Blacklisted.
author | hivewatchers |
---|---|
permlink | re-ayhamyou-qe99ei-20200730t110814241z |
category | bug |
json_metadata | {"app":"hivewatchers/0.5.1","format":"markdown+html"} |
created | 2020-07-30 11:08:15 |
last_update | 2020-07-30 11:08:15 |
depth | 2 |
children | 0 |
last_payout | 2020-08-06 11:08:15 |
cashout_time | 1969-12-31 23:59:59 |
total_payout_value | 0.458 HBD |
curator_payout_value | 0.459 HBD |
pending_payout_value | 0.000 HBD |
promoted | 0.000 HBD |
body_length | 170 |
author_reputation | 616,559,617,248,338 |
root_title | "Duplicate account history entries on STEEM: How to detect and fix them" |
beneficiaries | [] |
max_accepted_payout | 1,000,000.000 HBD |
percent_hbd | 10,000 |
post_id | 98,797,975 |
net_rshares | 3,261,625,859,090 |
author_curate_reward | "" |
voter | weight | wgt% | rshares | pct | time |
---|---|---|---|---|---|
adm | 0 | 3,209,422,368,931 | 9.5% | ||
sc-fund | 0 | 52,629,765,169 | 12.5% | ||
informativebot | 0 | -426,275,010 | -50% |
I have picked your post for my daily hive voting initiative, Keep it up and Hive On!!
author | chitty |
---|---|
permlink | re-duplicate-account-history-entries-on-steem-how-to-detect-and-fix-them-20200803t000954 |
category | bug |
json_metadata | "" |
created | 2020-08-03 00:09:57 |
last_update | 2020-08-03 00:09:57 |
depth | 1 |
children | 0 |
last_payout | 2020-08-10 00:09:57 |
cashout_time | 1969-12-31 23:59:59 |
total_payout_value | 0.000 HBD |
curator_payout_value | 0.000 HBD |
pending_payout_value | 0.000 HBD |
promoted | 0.000 HBD |
body_length | 86 |
author_reputation | 86,901,300,608,582 |
root_title | "Duplicate account history entries on STEEM: How to detect and fix them" |
beneficiaries | [] |
max_accepted_payout | 1,000,000.000 HBD |
percent_hbd | 10,000 |
post_id | 98,861,462 |
net_rshares | 0 |
.
author | diem |
---|---|
permlink | qkon2f |
category | bug |
json_metadata | {"app":"hiveblog/0.1"} |
created | 2020-12-01 22:42:18 |
last_update | 2020-12-07 07:12:30 |
depth | 1 |
children | 0 |
last_payout | 2020-12-08 22:42:18 |
cashout_time | 1969-12-31 23:59:59 |
total_payout_value | 0.000 HBD |
curator_payout_value | 0.000 HBD |
pending_payout_value | 0.000 HBD |
promoted | 0.000 HBD |
body_length | 1 |
author_reputation | 0 |
root_title | "Duplicate account history entries on STEEM: How to detect and fix them" |
beneficiaries | [] |
max_accepted_payout | 1,000,000.000 HBD |
percent_hbd | 10,000 |
post_id | 100,770,541 |
net_rshares | 0 |
Can you explain in layman terms how to use this script, and what is the result it returns? I mean, 6279 entries, 65.316 HIVE, 0.063 HBD does not seem to be the hive you made last year :D
author | ew-and-patterns |
---|---|
permlink | re-holger80-qe9knb |
category | bug |
json_metadata | {"tags":["bug"],"app":"peakd/2020.07.2"} |
created | 2020-07-30 04:04:27 |
last_update | 2020-07-30 04:04:27 |
depth | 1 |
children | 2 |
last_payout | 2020-08-06 04:04:27 |
cashout_time | 1969-12-31 23:59:59 |
total_payout_value | 0.048 HBD |
curator_payout_value | 0.049 HBD |
pending_payout_value | 0.000 HBD |
promoted | 0.000 HBD |
body_length | 186 |
author_reputation | 138,435,312,451,522 |
root_title | "Duplicate account history entries on STEEM: How to detect and fix them" |
beneficiaries | [] |
max_accepted_payout | 1,000,000.000 HBD |
percent_hbd | 10,000 |
post_id | 98,793,124 |
net_rshares | 439,244,147,688 |
author_curate_reward | "" |
voter | weight | wgt% | rshares | pct | time |
---|---|---|---|---|---|
holger80 | 0 | 439,244,147,688 | 10% | ||
dein-problem | 0 | 0 | -0.1% |
The script counts all ingoing and outgoing HIVE and HBD. Only when the balance is correct after checking the last history item, It can be used further. 65.316 HIVE, 0.063 HBD is my current HIVE/HBD liquid balance. 6279 entries are history items that have modified my balance. I'm working on a second script that writes an excel file, I will post this as well.
author | holger80 |
---|---|
permlink | re-ew-and-patterns-qe9q9v |
category | bug |
json_metadata | {"tags":["bug"],"app":"peakd/2020.07.2"} |
created | 2020-07-30 06:05:57 |
last_update | 2020-07-30 06:05:57 |
depth | 2 |
children | 1 |
last_payout | 2020-08-06 06:05:57 |
cashout_time | 1969-12-31 23:59:59 |
total_payout_value | 0.012 HBD |
curator_payout_value | 0.012 HBD |
pending_payout_value | 0.000 HBD |
promoted | 0.000 HBD |
body_length | 360 |
author_reputation | 358,857,509,568,825 |
root_title | "Duplicate account history entries on STEEM: How to detect and fix them" |
beneficiaries | [] |
max_accepted_payout | 1,000,000.000 HBD |
percent_hbd | 10,000 |
post_id | 98,794,264 |
net_rshares | 118,170,682,699 |
author_curate_reward | "" |
voter | weight | wgt% | rshares | pct | time |
---|---|---|---|---|---|
anli | 0 | 6,595,950,849 | 99% | ||
andrepol | 0 | 5,542,190,429 | 99% | ||
dustsweeper | 0 | 105,498,285,912 | 10.42% | ||
holycow2019 | 0 | 287,121,509 | 100% | ||
acguitar1 | 0 | 0 | 100% | ||
re2pair | 0 | 247,134,000 | 100% |
Does this include staking rewards?
author | sepracore |
---|---|
permlink | re-holger80-qeb91d |
category | bug |
json_metadata | {"tags":["bug"],"app":"peakd/2020.07.3"} |
created | 2020-07-31 01:49:00 |
last_update | 2020-07-31 01:49:00 |
depth | 3 |
children | 0 |
last_payout | 2020-08-07 01:49:00 |
cashout_time | 1969-12-31 23:59:59 |
total_payout_value | 0.000 HBD |
curator_payout_value | 0.000 HBD |
pending_payout_value | 0.000 HBD |
promoted | 0.000 HBD |
body_length | 34 |
author_reputation | 19,850,143,429,858 |
root_title | "Duplicate account history entries on STEEM: How to detect and fix them" |
beneficiaries | [] |
max_accepted_payout | 1,000,000.000 HBD |
percent_hbd | 10,000 |
post_id | 98,809,934 |
net_rshares | 0 |
author_curate_reward | "" |
voter | weight | wgt% | rshares | pct | time |
---|---|---|---|---|---|
acguitar1 | 0 | 0 | 100% |
innovation by taxation ;)
author | felix.herrmann |
---|---|
permlink | qe9xwo |
category | bug |
json_metadata | {"app":"hiveblog/0.1"} |
created | 2020-07-30 08:50:51 |
last_update | 2020-07-30 08:50:51 |
depth | 1 |
children | 0 |
last_payout | 2020-08-06 08:50:51 |
cashout_time | 1969-12-31 23:59:59 |
total_payout_value | 0.000 HBD |
curator_payout_value | 0.000 HBD |
pending_payout_value | 0.000 HBD |
promoted | 0.000 HBD |
body_length | 25 |
author_reputation | 106,963,781,638,598 |
root_title | "Duplicate account history entries on STEEM: How to detect and fix them" |
beneficiaries | [] |
max_accepted_payout | 1,000,000.000 HBD |
percent_hbd | 10,000 |
post_id | 98,796,183 |
net_rshares | 0 |
Thanks for sharing interesting information. Regards.
author | gladysgarcia |
---|---|
permlink | qeawfn |
category | bug |
json_metadata | {"app":"hiveblog/0.1"} |
created | 2020-07-30 20:46:00 |
last_update | 2020-07-30 20:46:00 |
depth | 1 |
children | 0 |
last_payout | 2020-08-06 20:46:00 |
cashout_time | 1969-12-31 23:59:59 |
total_payout_value | 0.000 HBD |
curator_payout_value | 0.000 HBD |
pending_payout_value | 0.000 HBD |
promoted | 0.000 HBD |
body_length | 52 |
author_reputation | 57,028,368,382 |
root_title | "Duplicate account history entries on STEEM: How to detect and fix them" |
beneficiaries | [] |
max_accepted_payout | 1,000,000.000 HBD |
percent_hbd | 10,000 |
post_id | 98,806,360 |
net_rshares | 0 |
The adjective for the adverb 'identically' is 'identical'. Use the phrase 'they are' rather than 'there are' when we are talking about things as in "...they are not in order...". Only use 'there are' when we are talking about existence as in "...there are more entries..."
author | leprechaun |
---|---|
permlink | re-holger80-qeab2o |
category | bug |
json_metadata | {"tags":["bug"],"app":"peakd/2020.07.2"} |
created | 2020-07-30 13:35:27 |
last_update | 2020-07-30 13:35:27 |
depth | 1 |
children | 0 |
last_payout | 2020-08-06 13:35:27 |
cashout_time | 1969-12-31 23:59:59 |
total_payout_value | 0.000 HBD |
curator_payout_value | 0.000 HBD |
pending_payout_value | 0.000 HBD |
promoted | 0.000 HBD |
body_length | 275 |
author_reputation | 36,778,764,966,701 |
root_title | "Duplicate account history entries on STEEM: How to detect and fix them" |
beneficiaries | [] |
max_accepted_payout | 1,000,000.000 HBD |
percent_hbd | 10,000 |
post_id | 98,800,201 |
net_rshares | 0 |
Hello @holger80! I am trying to sign on behalf of @leprechaun from @steemfiles. I pull a post that is on Steem and its a Comment object, p. Then I create a Hive Blockchain instance and try to post to it. I guess I must be doing something wrong. Can you tell me what? ```` tx = TransactionBuilder(blockchain_instance=bci) tx.appendOps(p) tx.appendSigner('steemfiles', 'posting') tx.verify_authority() tx.broadcast() ```` I keep getting this strange error: ```` File "lib/mirror2.py", line 85, in <module> tx.verify_authority() File "/home/ubuntu/.local/lib/python3.6/site-packages/beem/transactionbuilder.py", line 462, in verify_authority raise e File "/home/ubuntu/.local/lib/python3.6/site-packages/beem/transactionbuilder.py", line 453, in verify_authority args = {'trx': self.json()} File "/home/ubuntu/.local/lib/python3.6/site-packages/beem/transactionbuilder.py", line 135, in json self.constructTx() File "/home/ubuntu/.local/lib/python3.6/site-packages/beem/transactionbuilder.py", line 339, in constructTx ops.extend([Operation(op, appbase=appbase, prefix=self.blockchain.prefix)]) File "/home/ubuntu/.local/lib/python3.6/site-packages/beembase/objects.py", line 115, in __init__ super(Operation, self).__init__(*args, **kwargs) File "/home/ubuntu/.local/lib/python3.6/site-packages/beemgraphenebase/objects.py", line 35, in __init__ if len(op["type"]) > 10 and op["type"][-9:] == "operation": File "/home/ubuntu/.local/lib/python3.6/site-packages/beem/blockchainobject.py", line 210, in __getitem__ return super(BlockchainObject, self).__getitem__(key) KeyError: 'type' ````
author | leprechaun |
---|---|
permlink | re-holger80-qkrj9y |
category | bug |
json_metadata | {"tags":["bug"],"app":"peakd/2020.11.4"} |
created | 2020-12-03 12:13:12 |
last_update | 2020-12-03 12:13:12 |
depth | 1 |
children | 0 |
last_payout | 2020-12-10 12:13:12 |
cashout_time | 1969-12-31 23:59:59 |
total_payout_value | 0.000 HBD |
curator_payout_value | 0.000 HBD |
pending_payout_value | 0.000 HBD |
promoted | 0.000 HBD |
body_length | 1,642 |
author_reputation | 36,778,764,966,701 |
root_title | "Duplicate account history entries on STEEM: How to detect and fix them" |
beneficiaries | [] |
max_accepted_payout | 1,000,000.000 HBD |
percent_hbd | 10,000 |
post_id | 100,792,714 |
net_rshares | 0 |
Hi @holger80, you have received a small bonus upvote from MAXUV. This is to inform you that you now have [new MPATH tokens](https://hive-engine.com/?p=market&t=MPATH) in your Hive-Engine wallet. Please [read this post](https://peakd.com/hive-167922/@mpath/mpath-weekly-report-and-token-distribution-26-april-2020) for more information. Thanks for being a member of both MAXUV *and* MPATH!
author | maxuvv |
---|---|
permlink | re-duplicate-account-history-entries-on-steem-how-to-detect-and-fix-them-20200729t230552z |
category | bug |
json_metadata | "{"app": "rewarding/0.1.0"}" |
created | 2020-07-29 23:05:54 |
last_update | 2020-07-29 23:05:54 |
depth | 1 |
children | 0 |
last_payout | 2020-08-05 23:05:54 |
cashout_time | 1969-12-31 23:59:59 |
total_payout_value | 0.000 HBD |
curator_payout_value | 0.000 HBD |
pending_payout_value | 0.000 HBD |
promoted | 0.000 HBD |
body_length | 393 |
author_reputation | 32,074,948,443 |
root_title | "Duplicate account history entries on STEEM: How to detect and fix them" |
beneficiaries | [] |
max_accepted_payout | 1,000,000.000 HBD |
percent_hbd | 10,000 |
post_id | 98,789,867 |
net_rshares | 0 |
Interesting discovery, does each node server return the same replication pairs? At first glance, the core seems to be correct since the error only appears at the API level and and does not seem to be present on the Hive API? A problem occurred since they changed some things to control the data displayed on third party's frontend? <hr> <sub> [Witness FR - Gen X - Geek đ¤ Gamer đŽ Traveler âŠī¸](/@mintrawa/introducing-a-gen-x-geek-gamer-traveler-witness) </sub>
author | mintrawa |
---|---|
permlink | re-holger80-qe99i4 |
category | bug |
json_metadata | {"tags":["bug"],"app":"peakd/2020.07.2"} |
created | 2020-07-30 00:03:42 |
last_update | 2020-07-30 00:03:42 |
depth | 1 |
children | 4 |
last_payout | 2020-08-06 00:03:42 |
cashout_time | 1969-12-31 23:59:59 |
total_payout_value | 0.048 HBD |
curator_payout_value | 0.048 HBD |
pending_payout_value | 0.000 HBD |
promoted | 0.000 HBD |
body_length | 464 |
author_reputation | 18,694,802,429,423 |
root_title | "Duplicate account history entries on STEEM: How to detect and fix them" |
beneficiaries | [] |
max_accepted_payout | 1,000,000.000 HBD |
percent_hbd | 10,000 |
post_id | 98,790,528 |
net_rshares | 429,894,485,228 |
author_curate_reward | "" |
voter | weight | wgt% | rshares | pct | time |
---|---|---|---|---|---|
holger80 | 0 | 429,894,485,228 | 10% | ||
dein-problem | 0 | 0 | -0.1% |
I checked the results on several nodes and the results for my account were the same. I think the error can also appear on Hive. It just did not happen currently for my account. The error is on the API level, the balances that are returned for an account are correct.
author | holger80 |
---|---|
permlink | re-mintrawa-qe9qh1 |
category | bug |
json_metadata | {"tags":["bug"],"app":"peakd/2020.07.2"} |
created | 2020-07-30 06:10:18 |
last_update | 2020-07-30 06:10:18 |
depth | 2 |
children | 3 |
last_payout | 2020-08-06 06:10:18 |
cashout_time | 1969-12-31 23:59:59 |
total_payout_value | 0.012 HBD |
curator_payout_value | 0.012 HBD |
pending_payout_value | 0.000 HBD |
promoted | 0.000 HBD |
body_length | 268 |
author_reputation | 358,857,509,568,825 |
root_title | "Duplicate account history entries on STEEM: How to detect and fix them" |
beneficiaries | [] |
max_accepted_payout | 1,000,000.000 HBD |
percent_hbd | 10,000 |
post_id | 98,794,286 |
net_rshares | 116,520,775,953 |
author_curate_reward | "" |
voter | weight | wgt% | rshares | pct | time |
---|---|---|---|---|---|
anli | 0 | 6,468,890,597 | 99% | ||
andrepol | 0 | 5,434,843,673 | 99% | ||
dustsweeper | 0 | 102,561,567,933 | 10.11% | ||
mintrawa | 0 | 1,533,356,354 | 100% | ||
holycow2019 | 0 | 280,582,757 | 100% | ||
re2pair | 0 | 241,534,639 | 100% |
Thanks for the information, I just made the test via Postman with the function `get_ops_in_block` and all the transactions of the block **41868606** are duplicated in the answer ``` { "jsonrpc":"2.0", "method":"account_history_api.get_ops_in_block", "params":{"block_num":41868606,"only_virtual":false}, "id":1 } ``` So after some cross-checking I went back up to block **41868590** to finally have a block where the transactions inside are not duplicated. In the other direction, I had to get to block **41868612** for it to finally stop. <hr> <sub> [Witness FR - Gen X - Geek đ¤ Gamer đŽ Traveler âŠī¸](/@mintrawa/introducing-a-gen-x-geek-gamer-traveler-witness) </sub>
author | mintrawa |
---|---|
permlink | re-holger80-qe9uo4 |
category | bug |
json_metadata | {"tags":["bug"],"app":"peakd/2020.07.2"} |
created | 2020-07-30 07:40:54 |
last_update | 2020-07-30 07:40:54 |
depth | 3 |
children | 2 |
last_payout | 2020-08-06 07:40:54 |
cashout_time | 1969-12-31 23:59:59 |
total_payout_value | 0.000 HBD |
curator_payout_value | 0.000 HBD |
pending_payout_value | 0.000 HBD |
promoted | 0.000 HBD |
body_length | 679 |
author_reputation | 18,694,802,429,423 |
root_title | "Duplicate account history entries on STEEM: How to detect and fix them" |
beneficiaries | [] |
max_accepted_payout | 1,000,000.000 HBD |
percent_hbd | 10,000 |
post_id | 98,795,299 |
net_rshares | 0 |
Any chance an App can be made to calculate Hive taxes? I'd be willing to pay in Hive, HBD, or fiat for reports every year. I imagine there are others for whom this would be useful. I have not found any crypto tax service that can import Hive transactions.
author | shainemata |
---|---|
permlink | re-holger80-qeagg6 |
category | bug |
json_metadata | {"tags":["bug"],"app":"peakd/2020.07.2"} |
created | 2020-07-30 15:31:18 |
last_update | 2020-07-30 15:31:18 |
depth | 1 |
children | 5 |
last_payout | 2020-08-06 15:31:18 |
cashout_time | 1969-12-31 23:59:59 |
total_payout_value | 0.018 HBD |
curator_payout_value | 0.019 HBD |
pending_payout_value | 0.000 HBD |
promoted | 0.000 HBD |
body_length | 256 |
author_reputation | 16,815,853,019,995 |
root_title | "Duplicate account history entries on STEEM: How to detect and fix them" |
beneficiaries | [] |
max_accepted_payout | 1,000,000.000 HBD |
percent_hbd | 10,000 |
post_id | 98,801,842 |
net_rshares | 174,340,870,385 |
author_curate_reward | "" |
voter | weight | wgt% | rshares | pct | time |
---|---|---|---|---|---|
darkflame | 0 | 0 | 50% | ||
nulledgh0st | 0 | 174,340,870,385 | 100% |
We literally just launched our Beta for this exact dApp - https://HiveBit.io (@HiveBit). https://peakd.com/hive-183630/@nulledgh0st/hivebit-io-beta-export-your-hive-transaction-history-in-seconds-and-import-sync-to-tax-and-portfolio-svcs-koinly-cointracker-and We are currently connecting with various tax/accounting apps and companies out there to directly integrate HiveBit into their sites, so that if you join our premium service, you can API / sync you data instantly with ease. Currently, we offer a FREE data export via CSV file (which can be imported into various services). So far, ours works perfectly with Koinly.io, and we are close to being near perfect with CoinTracker.io. Visit our website and submit a request (we ask for your username and an email to send your CSV to) and we'll send you a CSV that you can try out for yourself. Our final version should be launching in a few days. :) It'll be free for CSV exports, and a paid version for the API/sync version with various companies. Also, join our community (@HiveHustlers) and Discord (https://discord.gg/yA7BYHQ) to stay up to date with everything that goes on in our ecosystem - which HiveBit is a part of.
author | nulledgh0st |
---|---|
permlink | re-shainemata-qemq2e |
category | bug |
json_metadata | {"tags":["bug"],"app":"peakd/2020.07.4"} |
created | 2020-08-06 06:30:15 |
last_update | 2020-08-06 06:33:27 |
depth | 2 |
children | 4 |
last_payout | 2020-08-13 06:30:15 |
cashout_time | 1969-12-31 23:59:59 |
total_payout_value | 0.012 HBD |
curator_payout_value | 0.012 HBD |
pending_payout_value | 0.000 HBD |
promoted | 0.000 HBD |
body_length | 1,185 |
author_reputation | 28,154,041,116,036 |
root_title | "Duplicate account history entries on STEEM: How to detect and fix them" |
beneficiaries | [] |
max_accepted_payout | 1,000,000.000 HBD |
percent_hbd | 10,000 |
post_id | 98,920,246 |
net_rshares | 99,687,393,873 |
author_curate_reward | "" |
voter | weight | wgt% | rshares | pct | time |
---|---|---|---|---|---|
shainemata | 0 | 99,687,393,873 | 100% |
This is great news. Thanks. I use Taxbit.com for my crypto taxes. They are able to work with CSV files. However, it would be awesome to have automated import.
author | shainemata |
---|---|
permlink | re-nulledgh0st-qenbqy |
category | bug |
json_metadata | {"tags":["bug"],"app":"peakd/2020.08.1"} |
created | 2020-08-06 14:18:33 |
last_update | 2020-08-06 14:18:33 |
depth | 3 |
children | 3 |
last_payout | 2020-08-13 14:18:33 |
cashout_time | 1969-12-31 23:59:59 |
total_payout_value | 0.000 HBD |
curator_payout_value | 0.000 HBD |
pending_payout_value | 0.000 HBD |
promoted | 0.000 HBD |
body_length | 161 |
author_reputation | 16,815,853,019,995 |
root_title | "Duplicate account history entries on STEEM: How to detect and fix them" |
beneficiaries | [] |
max_accepted_payout | 1,000,000.000 HBD |
percent_hbd | 10,000 |
post_id | 98,926,467 |
net_rshares | 0 |
I spoke to you and roadscape about this a long time ago, it was a change in how RocksDB handles things and there can be duplicate entries and you have to check if the IDs are the same. I am surprised it does not happen on Hive as I didn't hear of a fix. I had missing Json entries issue as well.
author | themarkymark |
---|---|
permlink | re-holger80-qe9eez |
category | bug |
json_metadata | {"tags":["bug"],"app":"peakd/2020.07.2"} |
created | 2020-07-30 01:49:48 |
last_update | 2020-07-30 01:49:48 |
depth | 1 |
children | 3 |
last_payout | 2020-08-06 01:49:48 |
cashout_time | 1969-12-31 23:59:59 |
total_payout_value | 0.036 HBD |
curator_payout_value | 0.036 HBD |
pending_payout_value | 0.000 HBD |
promoted | 0.000 HBD |
body_length | 297 |
author_reputation | 1,669,691,544,273,522 |
root_title | "Duplicate account history entries on STEEM: How to detect and fix them" |
beneficiaries | [] |
max_accepted_payout | 1,000,000.000 HBD |
percent_hbd | 10,000 |
post_id | 98,791,623 |
net_rshares | 334,527,284,112 |
author_curate_reward | "" |
voter | weight | wgt% | rshares | pct | time |
---|---|---|---|---|---|
r351574nc3 | 0 | -94,564,767,693 | -100% | ||
holger80 | 0 | 440,038,752,380 | 10% | ||
salty-mcgriddles | 0 | -14,303,941,488 | -100% | ||
dein-problem | 0 | 0 | -0.1% | ||
sultan-indo | 0 | 3,357,240,913 | 100% |
I run the script on your account and found 3 duplicate entries: ``` {'producer': 'themarkymark', 'vesting_shares': {'amount': '487047858', 'precision': 6, 'nai': '@@000000037'}, 'trx_id': '0000000000000000000000000000000000000000', 'block': 42304342, 'trx_in_block': 4294967295, 'op_in_trx': 0, 'virtual_op': 1, 'timestamp': '2020-04-06T13:01:27', 'account': 'themarkymark', 'type': 'producer_reward', '_id': '5efd96c86758e62daab6251b4fd40e286ab6f3c2', 'index': 2411563} {'producer': 'themarkymark', 'vesting_shares': {'amount': '487047842', 'precision': 6, 'nai': '@@000000037'}, 'trx_id': '0000000000000000000000000000000000000000', 'block': 42304354, 'trx_in_block': 4294967295, 'op_in_trx': 0, 'virtual_op': 1, 'timestamp': '2020-04-06T13:02:03', 'account': 'themarkymark', 'type': 'producer_reward', '_id': 'fa4ff605eaec77ec4949b475fba30f5372c70d69', 'index': 2411564} {'producer': 'themarkymark', 'vesting_shares': {'amount': '487047858', 'precision': 6, 'nai': '@@000000037'}, 'trx_id': '0000000000000000000000000000000000000000', 'block': 42304342, 'trx_in_block': 4294967295, 'op_in_trx': 0, 'virtual_op': 1, 'timestamp': '2020-04-06T13:01:27', 'account': 'themarkymark', 'type': 'producer_reward', '_id': '5efd96c86758e62daab6251b4fd40e286ab6f3c2', 'index': 2411565} {'producer': 'themarkymark', 'vesting_shares': {'amount': '487047842', 'precision': 6, 'nai': '@@000000037'}, 'trx_id': '0000000000000000000000000000000000000000', 'block': 42304354, 'trx_in_block': 4294967295, 'op_in_trx': 0, 'virtual_op': 1, 'timestamp': '2020-04-06T13:02:03', 'account': 'themarkymark', 'type': 'producer_reward', '_id': 'fa4ff605eaec77ec4949b475fba30f5372c70d69', 'index': 2411566} {'producer': 'themarkymark', 'vesting_shares': {'amount': '476907066', 'precision': 6, 'nai': '@@000000037'}, 'trx_id': '0000000000000000000000000000000000000000', 'block': 45322425, 'trx_in_block': 4294967295, 'op_in_trx': 0, 'virtual_op': 1, 'timestamp': '2020-07-20T15:27:15', 'account': 'themarkymark', 'type': 'producer_reward', '_id': 'a4409a3b8798fef22a0b3831e0be451433976298', 'index': 2637227} {'producer': 'themarkymark', 'vesting_shares': {'amount': '476907066', 'precision': 6, 'nai': '@@000000037'}, 'trx_id': '0000000000000000000000000000000000000000', 'block': 45322425, 'trx_in_block': 4294967295, 'op_in_trx': 0, 'virtual_op': 1, 'timestamp': '2020-07-20T15:27:15', 'account': 'themarkymark', 'type': 'producer_reward', '_id': 'a4409a3b8798fef22a0b3831e0be451433976298', 'index': 2637228} ``` There are not in order. As only one producer_reward is possible per block, these 6 entries are only 3 valid ones. The duplicate entries can also check directly on the API: ``` curl -s --data '{"jsonrpc":"2.0", "method":"account_history_api.get_account_history", "params":{"account":"themarkymark", "start":2411566, "limit":3}, "id":1}' https://api.hive.blog ```
author | holger80 |
---|---|
permlink | re-themarkymark-qe9trc |
category | bug |
json_metadata | {"tags":["bug"],"app":"peakd/2020.07.2"} |
created | 2020-07-30 07:21:15 |
last_update | 2020-07-30 07:30:06 |
depth | 2 |
children | 0 |
last_payout | 2020-08-06 07:21:15 |
cashout_time | 1969-12-31 23:59:59 |
total_payout_value | 0.012 HBD |
curator_payout_value | 0.012 HBD |
pending_payout_value | 0.000 HBD |
promoted | 0.000 HBD |
body_length | 2,852 |
author_reputation | 358,857,509,568,825 |
root_title | "Duplicate account history entries on STEEM: How to detect and fix them" |
beneficiaries | [] |
max_accepted_payout | 1,000,000.000 HBD |
percent_hbd | 10,000 |
post_id | 98,795,084 |
net_rshares | 119,359,292,687 |
author_curate_reward | "" |
voter | weight | wgt% | rshares | pct | time |
---|---|---|---|---|---|
anli | 0 | 6,413,695,490 | 99% | ||
andrepol | 0 | 5,381,350,145 | 99% | ||
dustsweeper | 0 | 107,045,650,302 | 10.53% | ||
holycow2019 | 0 | 277,293,153 | 100% | ||
re2pair | 0 | 241,303,597 | 100% |
Does using or not using MIRA change anything about this problem? <hr> <sub> [Witness FR - Gen X - Geek đ¤ Gamer đŽ Traveler âŠī¸](/@mintrawa/introducing-a-gen-x-geek-gamer-traveler-witness) </sub>
author | mintrawa |
---|---|
permlink | re-themarkymark-qe9g9n |
category | bug |
json_metadata | {"tags":["bug"],"app":"peakd/2020.07.2"} |
created | 2020-07-30 02:29:48 |
last_update | 2020-07-30 02:29:48 |
depth | 2 |
children | 1 |
last_payout | 2020-08-06 02:29:48 |
cashout_time | 1969-12-31 23:59:59 |
total_payout_value | 0.000 HBD |
curator_payout_value | 0.000 HBD |
pending_payout_value | 0.000 HBD |
promoted | 0.000 HBD |
body_length | 195 |
author_reputation | 18,694,802,429,423 |
root_title | "Duplicate account history entries on STEEM: How to detect and fix them" |
beneficiaries | [] |
max_accepted_payout | 1,000,000.000 HBD |
percent_hbd | 10,000 |
post_id | 98,792,077 |
net_rshares | 0 |
Hi @mintrawa. Please be aware the you have commented on a post (or comment) from themarkymark.The user have been kicked off other social media platforms and is harassing other users by continually downvoting them. The user also control and use the buildawhale account (An old voting bot) for this and are controlling several self controlled and doubtable blacklists. <center>We strongly recommend not to upvote and comment this user</center><center>Doing so will post you on our blacklist</center>
author | luext |
---|---|
permlink | 20200730t022956630z |
category | bug |
json_metadata | {"tags":["comment"],"app":"peakd/2020.07.1"} |
created | 2020-07-30 02:29:57 |
last_update | 2020-07-30 02:29:57 |
depth | 3 |
children | 0 |
last_payout | 2020-08-06 02:29:57 |
cashout_time | 1969-12-31 23:59:59 |
total_payout_value | 0.000 HBD |
curator_payout_value | 0.000 HBD |
pending_payout_value | 0.000 HBD |
promoted | 0.000 HBD |
body_length | 498 |
author_reputation | -776,273,771,260 |
root_title | "Duplicate account history entries on STEEM: How to detect and fix them" |
beneficiaries | [] |
max_accepted_payout | 1,000,000.000 HBD |
percent_hbd | 10,000 |
post_id | 98,792,081 |
net_rshares | 0 |