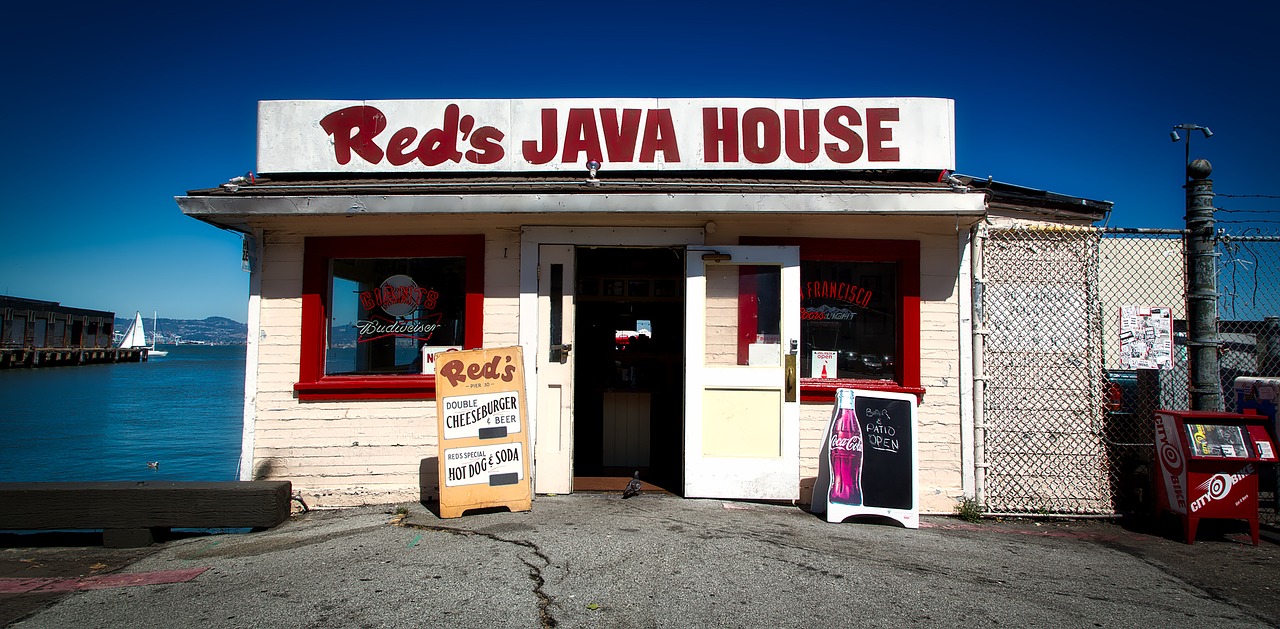 image source: [pixabay](https://pixabay.com/) *** 자동화 테스트에서 가장 중요한 것은 기대결과가 예상대로 노출되었는지 확인하는 것이다. 예를 들면 글 목록이 정상적으로 노출되는지, 목록의 글 제목, 저자명, 명성, 글 내용, 저자 썸네일 등이 노출되는지를 모두 체크해야 한다. 또한 어떤 태그가 노출되지 않았는지, 무슨 이유로 노출되지 않았는지 정확하게 기록하는 것도 필요하다. TestNG Asserting은 바로 이러한 것을 처리하기 위해 존재하며 주로 아래와 같은 asserting이 가장 많이 사용된다. (적어도 내 코드에는 자주 나타난다.) ``` // object가 null이 아닌지 체크, fail 발생했을 경우 리포트에 message 추가 Assert.assertNotNull(object, message); // condition이 True인지 체크, fail 발생했을 경우 리포트에 message 추가 Assert.assertTrue(condition, message); // Assert.assertTrue와 반대의 개념 Assert.assertFalse(condition, message); //actual과 expected가 똑같은지 체크(타입도 체크함), fail 발생했을 경우 리포트에 message 추가 Assert.assertEquals(actual, expected, message); ``` 👆 먼저 `import org.testng.Assert.*;` 라이브러리 import 후 코드는 위처럼 작성하면 된다. static 으로 import 하면 코드에 Assert.는 안적어도 된다. 👇 ``` import static org.testng.Assert.assertEquals; import static org.testng.Assert.assertNotNull; import static org.testng.Assert.assertTrue; ... ... assertNotNull(object, message); assertTrue(condition, message); assertFalse(condition, message); assertEquals(actual, expected, message); ``` 👇 아래 코드는 1)글 목록이 노출되었는지 2)비밀번호 태그를 불러왔는지 3)글쓰기 버튼이 노출되는지를 체크한다. 3번은 일부러 fail이 발생하게 체크했다. 스팀잇 페이지 dom에 글쓰기 버튼이 하나가 있는데 일부러 두개 있는지 체크하여 fail을 발생시킨다. ``` package com.steem.webatuo; import static org.testng.Assert.assertEquals; import static org.testng.Assert.assertNotNull; import static org.testng.Assert.assertTrue; import java.util.List; import java.util.concurrent.TimeUnit; import org.openqa.selenium.By; import org.openqa.selenium.Keys; import org.openqa.selenium.WebDriver; import org.openqa.selenium.WebElement; import org.openqa.selenium.chrome.ChromeDriver; import org.testng.annotations.AfterClass; import org.testng.annotations.BeforeClass; import org.testng.annotations.Test; import io.github.bonigarcia.wdm.WebDriverManager; public class Steemit { WebDriver driver; @BeforeClass public void SetUp() { WebDriverManager.chromedriver().setup(); driver = new ChromeDriver(); driver.get("STEEMIT URL"); driver.manage().window().maximize(); driver.manage().timeouts().implicitlyWait(3, TimeUnit.SECONDS); } @Test public void CASE_01_GetPosts() { List<WebElement> list = driver.findElements(By.xpath("//div[@id='posts_list']/ul/li")); assertTrue(!list.isEmpty(), "글 목록이 있는지 확인"); for (WebElement post : list) { String title = post.findElement(By.xpath("descendant::h2/a")).getText(); String author = post.findElement(By.xpath("descendant::span[@class='user__name']")).getText(); System.out.println(author + "님의 글: " + title); } } @Test public void CASE_02_Login() throws InterruptedException { // Click the login button driver.findElement(By.linkText("로그인")).click(); // type id driver.findElement(By.name("username")).sendKeys("june0620"); // type pw and submit WebElement pw = driver.findElement(By.name("password")); assertNotNull(pw, "비밀번호 태그가 노출되는지 확인"); pw.sendKeys(Password.getPw()); pw.submit(); Thread.sleep(5000); } @Test public void CASE_03_Write() throws InterruptedException { // Click the write Button List<WebElement> writeBtns = driver.findElements(By.xpath("//a[@href='/submit.html']")); assertEquals(writeBtns.size(), 2, "글쓰기 버튼이 노출되는지 확인"); for (WebElement writeBtn : writeBtns) { if (writeBtn.isDisplayed()) { writeBtn.click(); Thread.sleep(2000); // type Text and Keys WebElement editor = driver.findElement(By.xpath("//textarea[@name='body']")); String keys = Keys.chord(Keys.LEFT_SHIFT, Keys.ENTER); editor.sendKeys("hello!! world", keys, "hello!!! STEEMIT", Keys.ENTER, "안녕, 스팀잇", Keys.ENTER, "你好!似提姆"); break; } } Thread.sleep(5000); } @AfterClass public void tearDownClass() { driver.quit(); } } ``` 실행 해 보면 아래와 같이 fail 이 발생하고 리포트에 정확하게 내가 작성한 메시지가 찍히는 것을 볼 수 있다. 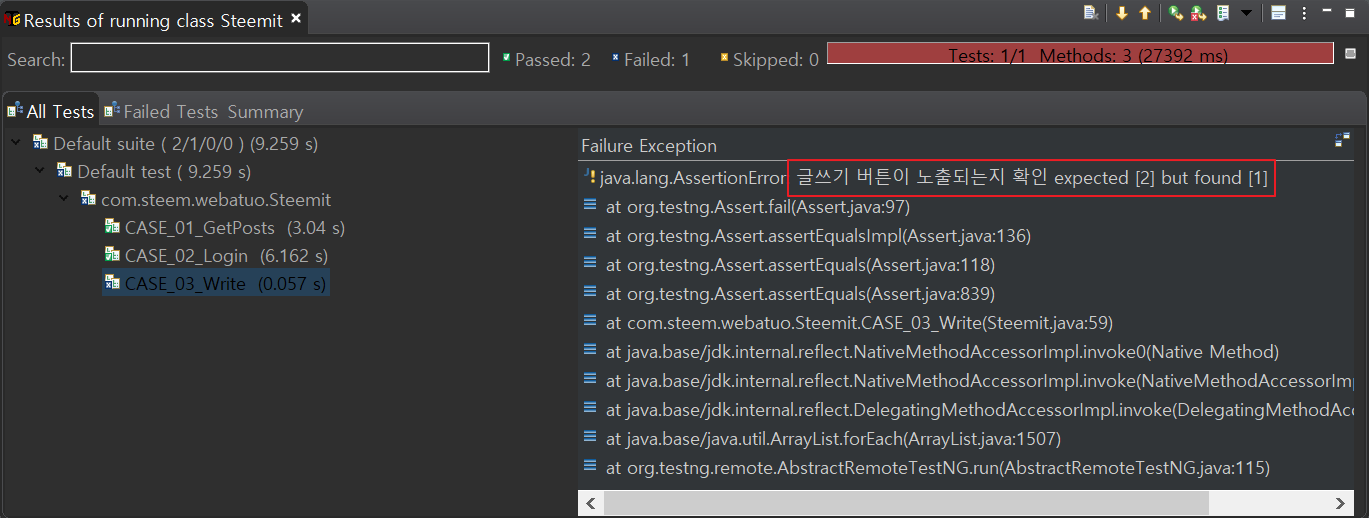 <sub> **만약 리포트에 한글이 깨질 경우 Eclipse가 설치된 경로에서 eclipse.ini 파일 맨 아래에 `-Dfile.encoding=UTF-8`를 넣으면 해결된다. 보통 경로는 `C:\Users\{사용자 컴퓨터 이름}\eclipse`이다.** 👇</sub> 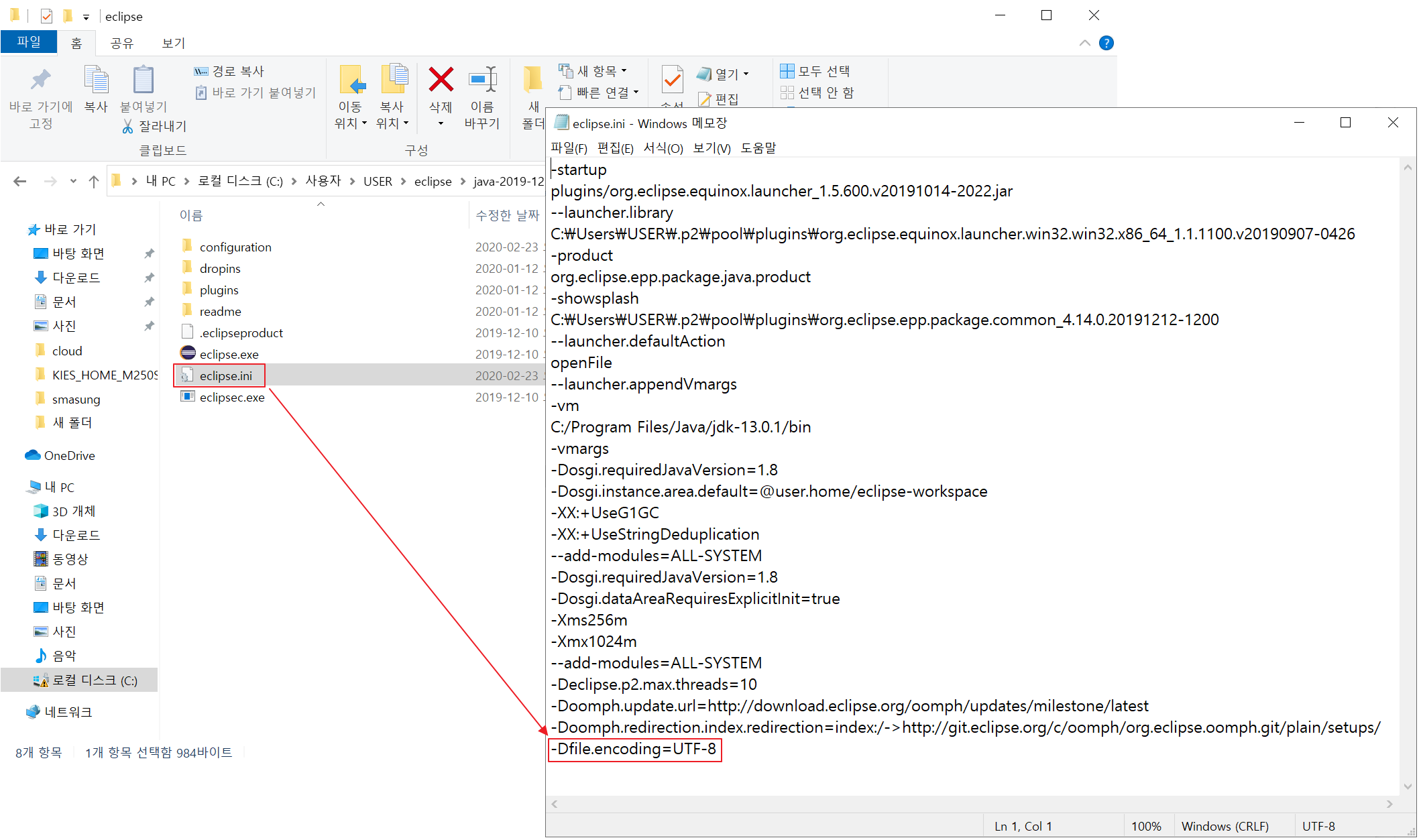 추가로 아래와 같은 메소드도 지원하지만 자주 다뤄보지 않아서 잘 모르겠다. 나중에 좀 친해져 보도록 하고 오늘은 이만 자즈~~아👇 ``` assertEqualsDeep(actual, expected); assertEqualsNoOrder(actual, expected); assertNotEquals(actual, expected); assertNotEqualsDeep(actual, expected); assertSame(actual, expected); assertNotSame(actual, expected); assertNull(object, message); assertThrows(throwableClass, runnable); ``` . . . . [Cookie 😅] Seleniun java lib version: 3.141.59 java version: 13.0.1
author | june0620 |
---|---|
permlink | java-13-web-automation-13-selenium-with-testng-2-asserting-kr |
category | hive-101145 |
json_metadata | {"tags":["hive-101145","sct-kr","sct-freeboard","kr","mini","steemstem","zzan","palnet","dblog","sct"],"image":["https://files.steempeak.com/file/steempeak/june0620/DKldjbG3-reds-java-house-1591357_1280.jpg","https://files.steempeak.com/file/steempeak/june0620/dtKozYeX-image.png","https://files.steempeak.com/file/steempeak/june0620/eg63R0EO-image.png"],"links":["https://pixabay.com/"],"app":"steemcoinpan/0.1","format":"markdown","canonical_url":"https://www.steemcoinpan.com/@june0620/java-13-web-automation-13-selenium-with-testng-2-asserting-kr"} |
created | 2020-03-10 13:12:42 |
last_update | 2020-03-10 13:12:42 |
depth | 0 |
children | 13 |
last_payout | 2020-03-17 13:12:42 |
cashout_time | 1969-12-31 23:59:59 |
total_payout_value | 4.675 HBD |
curator_payout_value | 3.664 HBD |
pending_payout_value | 0.000 HBD |
promoted | 0.000 HBD |
body_length | 4,815 |
author_reputation | 118,592,211,436,406 |
root_title | "[JAVA #13] [Web Automation #13] Selenium With TestNG #2 Asserting [KR]" |
beneficiaries | [] |
max_accepted_payout | 1,000,000.000 HBD |
percent_hbd | 10,000 |
post_id | 96,234,019 |
net_rshares | 34,686,296,683,441 |
author_curate_reward | "" |
voter | weight | wgt% | rshares | pct | time |
---|---|---|---|---|---|
gerber | 0 | 47,054,314,219 | 2% | ||
daan | 0 | 169,963,632,707 | 8% | ||
slowwalker | 0 | 556,768,499,036 | 7% | ||
exyle | 0 | 694,664,341,548 | 7% | ||
magicmonk | 0 | 1,797,229,584,383 | 100% | ||
tumutanzi | 0 | 1,794,980,001 | 50% | ||
oldstone | 0 | 246,362,849,995 | 5% | ||
cjsdns | 0 | 1,509,777,419,889 | 6% | ||
ramires | 0 | 6,918,748,496 | 25% | ||
coldhair | 0 | 892,445,338 | 50% | ||
ucukertz | 0 | 525,539,399 | 25% | ||
imperfect-one | 0 | 535,968,924 | 5.01% | ||
mys | 0 | 9,620,988,255 | 5.01% | ||
lotusofmymom | 0 | 7,685,938,485 | 7% | ||
allsale | 0 | 394,007,193,205 | 100% | ||
virus707 | 0 | 3,382,229,722,900 | 19% | ||
shenchensucc | 0 | 120,544,995,524 | 30% | ||
ew-and-patterns | 0 | 169,898,247,825 | 8% | ||
techken | 0 | 0 | 0.14% | ||
whd | 0 | 1,183,180,181 | 1.01% | ||
khaiyoui | 0 | 956,627,940,350 | 25% | ||
jesus.christ | 0 | 467,019,625 | 5% | ||
ilovemylife | 0 | 680,960,561,154 | 30% | ||
tipu | 0 | 6,856,540,735,715 | 10% | ||
seapy | 0 | 8,250,497,339 | 60% | ||
accelerator | 0 | 32,649,636,339 | 2% | ||
june0620 | 0 | 1,152,378,927,765 | 100% | ||
roleerob | 0 | 624,916,352 | 0.2% | ||
xiaoshancun | 0 | 12,323,319,782 | 100% | ||
minloulou | 0 | 2,235,781,100 | 10% | ||
toocurious | 0 | 997,610,533 | 1.98% | ||
lindalex | 0 | 580,290,767 | 50% | ||
blockbrothers | 0 | 95,358,694,605 | 3.5% | ||
cnbuddy | 0 | 1,659,023,132,365 | 100% | ||
banjjakism | 0 | 5,989,860,431 | 9.5% | ||
funtraveller | 0 | 725,603,143,973 | 50% | ||
gamezine | 0 | 4,472,882,700 | 25% | ||
stupid | 0 | 705,565,166 | 40% | ||
mehta | 0 | 136,449,943,660 | 40% | ||
suhunter | 0 | 953,109,410 | 50% | ||
udabeu | 0 | 12,858,540,679 | 30% | ||
marsswim | 0 | 107,206,995,491 | 51% | ||
cadawg | 0 | 4,910,348,135 | 1.4% | ||
photoholic | 0 | 349,222,114,417 | 7% | ||
maikuraki | 0 | 5,459,443,131 | 19% | ||
fego | 0 | 4,609,507,150 | 3.5% | ||
earlmonk | 0 | 827,360,125 | 7% | ||
beleg | 0 | 1,062,779,486 | 2.52% | ||
bestboom | 0 | 16,526,960,210 | 2% | ||
minuteman111 | 0 | 3,371,359,649 | 100% | ||
stmdev | 0 | 209,174,731 | 2% | ||
lucky2015 | 0 | 352,808,744,037 | 100% | ||
alitavirgen | 0 | 17,865,715,361 | 11% | ||
gghite | 0 | 385,910,917,791 | 60% | ||
angelinafx | 0 | 5,901,288,382 | 2.1% | ||
jaydih | 0 | 485,608,308,716 | 11% | ||
andrewma | 0 | 12,157,250,139 | 50% | ||
kimseun | 0 | 70,851,735,035 | 11% | ||
wisdomandjustice | 0 | 365,425,602,506 | 5% | ||
pilimili | 0 | 462,761,559 | 95% | ||
freegon | 0 | 192,842,624,359 | 100% | ||
kanadaramagi123 | 0 | 48,235,783,590 | 11% | ||
suonghuynh | 0 | 285,017,628,155 | 2.1% | ||
loveecho | 0 | 86,330,198,712 | 11% | ||
wanggang | 0 | 151,003,852,937 | 20% | ||
mightypanda | 0 | 1,910,954,221 | 3.5% | ||
pagliozzo | 0 | 16,563,864,874 | 20% | ||
angelslake | 0 | 18,355,258,930 | 11% | ||
smartvote | 0 | 210,032,650,969 | 2.1% | ||
julian2013 | 0 | 1,689,693,671 | 0.45% | ||
dlike | 0 | 191,639,805,417 | 2.95% | ||
triptolemus | 0 | 8,109,679,860 | 2% | ||
melaniewang | 0 | 8,172,469,635 | 50% | ||
wordit | 0 | 17,755,537,159 | 11% | ||
changxiu | 0 | 5,053,333,843 | 50% | ||
sj-jeong | 0 | 580,841,536,213 | 11% | ||
wondumyungga | 0 | 39,273,902,757 | 11% | ||
laissez-faire | 0 | 55,796,745 | 100% | ||
aquawink | 0 | 46,473,096,820 | 11% | ||
cherryzz | 0 | 179,035,133,563 | 50% | ||
djusti | 0 | 986,838,262,086 | 100% | ||
ringit | 0 | 30,193,066,070 | 11% | ||
coreabeforekorea | 0 | 354,528,717,526 | 11% | ||
talkative-bk | 0 | 456,326,777,049 | 11% | ||
nineteensixteen | 0 | 674,017,147,368 | 100% | ||
sindong | 0 | 607,634,103,733 | 80% | ||
steemfriends | 0 | 4,743,736,422 | 100% | ||
swiftbot | 0 | 578,235,915 | 5% | ||
cyberrn | 0 | 728,430,616,259 | 16% | ||
sklara | 0 | 53,070,590,554 | 30% | ||
mia-cc | 0 | 593,505,012 | 5% | ||
tina3721 | 0 | 4,865,573,237 | 50% | ||
andyhsia | 0 | 6,736,005,620 | 100% | ||
gregoryhedrich | 0 | 58,120,132 | 49% | ||
minigame | 0 | 2,769,039,528,643 | 8% | ||
whatdidshewear | 0 | 90,254,890,347 | 11% | ||
jjm13 | 0 | 17,974,171,640 | 19% | ||
sjgod4018 | 0 | 73,683,147,030 | 11% | ||
mustard-seed | 0 | 35,814,918,494 | 11% | ||
freizeitcenter | 0 | 204,698,653 | 50% | ||
steemindian | 0 | 728,151,449 | 3.5% | ||
fsm-liquid | 0 | 18,934,261,494 | 7% | ||
jalentakesphotos | 0 | 2,646,611,838 | 10% | ||
zzan.hmy | 0 | 1,137,309,027 | 2% | ||
oldstone.sct | 0 | 3,809,765,053 | 7% | ||
triplea.bot | 0 | 2,169,024,010 | 7% | ||
olaf123 | 0 | 1,191,258,541 | 1% | ||
steem.leo | 0 | 58,856,706,144 | 1.98% | ||
mini.sct | 0 | 2,396,927,967 | 40% | ||
leo.voter | 0 | 96,891,177,779 | 1.92% | ||
cn-sct | 0 | 1,907,041,841 | 2% | ||
freddio.sport | 0 | 42,024,692,127 | 15% | ||
sct1004 | 0 | 402,284,434 | 20% | ||
freegon.sct | 0 | 9,452,765,072 | 100% | ||
tokenindustry | 0 | 3,457,935,818 | 80% | ||
sct.krwp | 0 | 156,209,592,710 | 0.23% | ||
leo.syndication | 0 | 933,742,371 | 2% | ||
one.life | 0 | 17,447,869,093 | 1.99% | ||
maxuva | 0 | 999,151,233 | 2% | ||
maxuvb | 0 | 996,999,967 | 2% | ||
maxuvc | 0 | 946,679,368 | 2% | ||
maxuvd | 0 | 945,444,737 | 2% | ||
dappstats | 0 | 6,715,467,363 | 15% | ||
sct.curator | 0 | 20,275,416,527 | 24.39% | ||
therealyme | 0 | 5,600,812,934 | 5.6% | ||
jaykayw | 0 | 24,583,323,318 | 11% | ||
cnbuddy-reward | 0 | 999,395,098,452 | 100% | ||
roseofmylife | 0 | 123,916,169,959 | 7% | ||
springflower | 0 | 2,237,066,799 | 7% | ||
mychoco | 0 | 1,320,203,929 | 7% | ||
morningcare | 0 | 3,574,595,739 | 5% | ||
real3earch | 0 | 8,065,017,402 | 100% | ||
unpopular | 0 | 34,779,939,461 | 40% | ||
we-together | 0 | 377,837,969,489 | 7% | ||
steem-agora | 0 | 1,630,620,634 | 7% | ||
dblogvoter | 0 | 95,538,490 | 1.95% | ||
reward.tier2 | 0 | 2,064,259,499 | 100% | ||
p-translation | 0 | 1,824,620,912 | 7% | ||
sammivo | 0 | 1,273,298,736 | 89% | ||
bilpcoinpower | 0 | 194,871,627 | 10% | ||
toni.pal | 0 | 0 | 0.66% | ||
steemcityrewards | 0 | 8,339,319,707 | 1.2% |
Hi🙋 @tipu curate 2 !shop Posted using [Partiko Android](https://partiko.app/referral/annepink)
author | annepink |
---|---|
permlink | annepink-re-june0620-java-13-web-automation-13-selenium-with-testng-2-asserting-kr-20200310t131645972z |
category | hive-101145 |
json_metadata | {"app":"partiko","client":"android"} |
created | 2020-03-10 13:16:45 |
last_update | 2020-03-10 13:16:45 |
depth | 1 |
children | 3 |
last_payout | 2020-03-17 13:16:45 |
cashout_time | 1969-12-31 23:59:59 |
total_payout_value | 0.000 HBD |
curator_payout_value | 0.000 HBD |
pending_payout_value | 0.000 HBD |
promoted | 0.000 HBD |
body_length | 95 |
author_reputation | 1,031,665,409,251,374 |
root_title | "[JAVA #13] [Web Automation #13] Selenium With TestNG #2 Asserting [KR]" |
beneficiaries | [] |
max_accepted_payout | 1,000,000.000 HBD |
percent_hbd | 10,000 |
post_id | 96,234,144 |
net_rshares | 0 |
晚上哈喽啊 😁 多谢萍萍无私的奉献 哈哈
author | june0620 | ||||||
---|---|---|---|---|---|---|---|
permlink | re-annepink-2020310t2221929z | ||||||
category | hive-101145 | ||||||
json_metadata | {"tags":["esteem"],"app":"esteem/2.2.4-mobile","format":"markdown+html","community":"hive-125125"} | ||||||
created | 2020-03-10 13:21:09 | ||||||
last_update | 2020-03-10 13:21:09 | ||||||
depth | 2 | ||||||
children | 1 | ||||||
last_payout | 2020-03-17 13:21:09 | ||||||
cashout_time | 1969-12-31 23:59:59 | ||||||
total_payout_value | 0.000 HBD | ||||||
curator_payout_value | 0.000 HBD | ||||||
pending_payout_value | 0.000 HBD | ||||||
promoted | 0.000 HBD | ||||||
body_length | 21 | ||||||
author_reputation | 118,592,211,436,406 | ||||||
root_title | "[JAVA #13] [Web Automation #13] Selenium With TestNG #2 Asserting [KR]" | ||||||
beneficiaries |
| ||||||
max_accepted_payout | 1,000,000.000 HBD | ||||||
percent_hbd | 10,000 | ||||||
post_id | 96,234,255 | ||||||
net_rshares | 0 |
我没奉献神马呀😅 Posted using [Partiko Android](https://partiko.app/referral/annepink)
author | annepink |
---|---|
permlink | annepink-re-june0620-re-annepink-2020310t2221929z-20200310t145345130z |
category | hive-101145 |
json_metadata | {"app":"partiko","client":"android"} |
created | 2020-03-10 14:53:45 |
last_update | 2020-03-10 14:53:45 |
depth | 3 |
children | 0 |
last_payout | 2020-03-17 14:53:45 |
cashout_time | 1969-12-31 23:59:59 |
total_payout_value | 0.000 HBD |
curator_payout_value | 0.000 HBD |
pending_payout_value | 0.000 HBD |
promoted | 0.000 HBD |
body_length | 79 |
author_reputation | 1,031,665,409,251,374 |
root_title | "[JAVA #13] [Web Automation #13] Selenium With TestNG #2 Asserting [KR]" |
beneficiaries | [] |
max_accepted_payout | 1,000,000.000 HBD |
percent_hbd | 10,000 |
post_id | 96,236,673 |
net_rshares | 0 |
<a href="https://tipu.online/curator?annepink" target="_blank">Upvoted 👌</a> (Mana: 10/20 - <a href="https://steempeak.com/steem/@tipu/tipu-curate-project-update-recharging-curation-mana" target="_blank">need recharge</a>?)
author | tipu |
---|---|
permlink | re-annepink-re-june0620-java-13-web-automation-13-selenium-with-testng-2-asserting-kr-20200310t131645972z-20200310t131709 |
category | hive-101145 |
json_metadata | "" |
created | 2020-03-10 13:17:03 |
last_update | 2020-03-10 13:17:03 |
depth | 2 |
children | 0 |
last_payout | 2020-03-17 13:17:03 |
cashout_time | 1969-12-31 23:59:59 |
total_payout_value | 0.000 HBD |
curator_payout_value | 0.000 HBD |
pending_payout_value | 0.000 HBD |
promoted | 0.000 HBD |
body_length | 232 |
author_reputation | 55,920,881,392,558 |
root_title | "[JAVA #13] [Web Automation #13] Selenium With TestNG #2 Asserting [KR]" |
beneficiaries | [] |
max_accepted_payout | 1,000,000.000 HBD |
percent_hbd | 10,000 |
post_id | 96,234,154 |
net_rshares | 0 |
### According to the Bible, *The Book of Judas: Should You Believe It?* ### Watch the Video below to know the Answer... ***(Sorry for sending this comment. We are not looking for our self profit, our intentions is to preach the words of God in any means possible.)*** https://youtu.be/cozSKLCYYwQ Comment what you understand of our Youtube Video to receive our full votes. We have 30,000 #SteemPower. It's our little way to **Thank you, our beloved friend.** Check our [Discord Chat](https://discord.gg/vzHFNd6) Join our Official Community: https://steemit.com/created/hive-182074
author | olaf123 |
---|---|
permlink | eq6tm5zztq |
category | hive-101145 |
json_metadata | "" |
created | 2020-03-10 13:20:57 |
last_update | 2020-03-10 13:20:57 |
depth | 1 |
children | 0 |
last_payout | 2020-03-17 13:20:57 |
cashout_time | 1969-12-31 23:59:59 |
total_payout_value | 0.000 HBD |
curator_payout_value | 0.000 HBD |
pending_payout_value | 0.000 HBD |
promoted | 0.000 HBD |
body_length | 585 |
author_reputation | -10,813,981,844,129 |
root_title | "[JAVA #13] [Web Automation #13] Selenium With TestNG #2 Asserting [KR]" |
beneficiaries | [] |
max_accepted_payout | 10,000.000 HBD |
percent_hbd | 100 |
post_id | 96,234,249 |
net_rshares | -24,817,924,703 |
author_curate_reward | "" |
voter | weight | wgt% | rshares | pct | time |
---|---|---|---|---|---|
mack-bot | 0 | -26,004,151,765 | -0.25% | ||
olaf123 | 0 | 1,186,227,062 | 1% |
@tipu curate
author | ravenkim |
---|---|
permlink | re-june0620-java-13-web-automation-13-selenium-with-testng-2-asserting-kr-20200310t131641007z |
category | hive-101145 |
json_metadata | {"community":"busy","app":"busy/2.5.6","format":"markdown","tags":["hive-101145"],"users":["tipu"],"links":["/@tipu"],"image":[]} |
created | 2020-03-10 13:16:42 |
last_update | 2020-03-10 13:16:42 |
depth | 1 |
children | 6 |
last_payout | 2020-03-17 13:16:42 |
cashout_time | 1969-12-31 23:59:59 |
total_payout_value | 0.000 HBD |
curator_payout_value | 0.000 HBD |
pending_payout_value | 0.000 HBD |
promoted | 0.000 HBD |
body_length | 12 |
author_reputation | 107,302,486,367,072 |
root_title | "[JAVA #13] [Web Automation #13] Selenium With TestNG #2 Asserting [KR]" |
beneficiaries | [] |
max_accepted_payout | 1,000,000.000 HBD |
percent_hbd | 10,000 |
post_id | 96,234,140 |
net_rshares | 0 |
擦 算你的还是算我的呢😂 Posted using [Partiko Android](https://partiko.app/referral/annepink)
author | annepink |
---|---|
permlink | annepink-re-ravenkim-re-june0620-java-13-web-automation-13-selenium-with-testng-2-asserting-kr-20200310t132027454z |
category | hive-101145 |
json_metadata | {"app":"partiko","client":"android"} |
created | 2020-03-10 13:20:27 |
last_update | 2020-03-10 13:20:27 |
depth | 2 |
children | 3 |
last_payout | 2020-03-17 13:20:27 |
cashout_time | 1969-12-31 23:59:59 |
total_payout_value | 0.000 HBD |
curator_payout_value | 0.000 HBD |
pending_payout_value | 0.000 HBD |
promoted | 0.000 HBD |
body_length | 83 |
author_reputation | 1,031,665,409,251,374 |
root_title | "[JAVA #13] [Web Automation #13] Selenium With TestNG #2 Asserting [KR]" |
beneficiaries | [] |
max_accepted_payout | 1,000,000.000 HBD |
percent_hbd | 10,000 |
post_id | 96,234,243 |
net_rshares | 0 |
应该是算你的了 😁😀
author | june0620 | ||||||
---|---|---|---|---|---|---|---|
permlink | re-annepink-2020310t22224745z | ||||||
category | hive-101145 | ||||||
json_metadata | {"tags":["esteem"],"app":"esteem/2.2.4-mobile","format":"markdown+html","community":"hive-125125"} | ||||||
created | 2020-03-10 13:22:48 | ||||||
last_update | 2020-03-10 13:22:48 | ||||||
depth | 3 | ||||||
children | 1 | ||||||
last_payout | 2020-03-17 13:22:48 | ||||||
cashout_time | 1969-12-31 23:59:59 | ||||||
total_payout_value | 0.000 HBD | ||||||
curator_payout_value | 0.000 HBD | ||||||
pending_payout_value | 0.000 HBD | ||||||
promoted | 0.000 HBD | ||||||
body_length | 10 | ||||||
author_reputation | 118,592,211,436,406 | ||||||
root_title | "[JAVA #13] [Web Automation #13] Selenium With TestNG #2 Asserting [KR]" | ||||||
beneficiaries |
| ||||||
max_accepted_payout | 1,000,000.000 HBD | ||||||
percent_hbd | 10,000 | ||||||
post_id | 96,234,295 | ||||||
net_rshares | 0 |
?
author | ravenkim |
---|---|
permlink | re-annepink-annepink-re-ravenkim-re-june0620-java-13-web-automation-13-selenium-with-testng-2-asserting-kr-20200310t134500418z |
category | hive-101145 |
json_metadata | {"community":"busy","app":"busy/2.5.6","format":"markdown","tags":["hive-101145"],"users":[],"links":[],"image":[]} |
created | 2020-03-10 13:45:03 |
last_update | 2020-03-10 13:45:03 |
depth | 3 |
children | 0 |
last_payout | 2020-03-17 13:45:03 |
cashout_time | 1969-12-31 23:59:59 |
total_payout_value | 0.000 HBD |
curator_payout_value | 0.000 HBD |
pending_payout_value | 0.000 HBD |
promoted | 0.000 HBD |
body_length | 1 |
author_reputation | 107,302,486,367,072 |
root_title | "[JAVA #13] [Web Automation #13] Selenium With TestNG #2 Asserting [KR]" |
beneficiaries | [] |
max_accepted_payout | 1,000,000.000 HBD |
percent_hbd | 10,000 |
post_id | 96,234,836 |
net_rshares | 0 |
감사합니다^^
author | june0620 | ||||||
---|---|---|---|---|---|---|---|
permlink | re-ravenkim-2020310t222218717z | ||||||
category | hive-101145 | ||||||
json_metadata | {"tags":["esteem"],"app":"esteem/2.2.4-mobile","format":"markdown+html","community":"hive-125125"} | ||||||
created | 2020-03-10 13:22:21 | ||||||
last_update | 2020-03-10 13:22:21 | ||||||
depth | 2 | ||||||
children | 0 | ||||||
last_payout | 2020-03-17 13:22:21 | ||||||
cashout_time | 1969-12-31 23:59:59 | ||||||
total_payout_value | 0.000 HBD | ||||||
curator_payout_value | 0.000 HBD | ||||||
pending_payout_value | 0.000 HBD | ||||||
promoted | 0.000 HBD | ||||||
body_length | 7 | ||||||
author_reputation | 118,592,211,436,406 | ||||||
root_title | "[JAVA #13] [Web Automation #13] Selenium With TestNG #2 Asserting [KR]" | ||||||
beneficiaries |
| ||||||
max_accepted_payout | 1,000,000.000 HBD | ||||||
percent_hbd | 10,000 | ||||||
post_id | 96,234,284 | ||||||
net_rshares | 0 |
<a href="https://tipu.online/curator?ravenkim" target="_blank">Upvoted 👌</a> (Mana: 15/25 - <a href="https://steempeak.com/steem/@tipu/tipu-curate-project-update-recharging-curation-mana" target="_blank">need recharge</a>?)
author | tipu |
---|---|
permlink | re-re-june0620-java-13-web-automation-13-selenium-with-testng-2-asserting-kr-20200310t131641007z-20200310t131700 |
category | hive-101145 |
json_metadata | "" |
created | 2020-03-10 13:16:57 |
last_update | 2020-03-10 13:16:57 |
depth | 2 |
children | 0 |
last_payout | 2020-03-17 13:16:57 |
cashout_time | 1969-12-31 23:59:59 |
total_payout_value | 0.000 HBD |
curator_payout_value | 0.000 HBD |
pending_payout_value | 0.000 HBD |
promoted | 0.000 HBD |
body_length | 232 |
author_reputation | 55,920,881,392,558 |
root_title | "[JAVA #13] [Web Automation #13] Selenium With TestNG #2 Asserting [KR]" |
beneficiaries | [] |
max_accepted_payout | 1,000,000.000 HBD |
percent_hbd | 10,000 |
post_id | 96,234,148 |
net_rshares | 0 |
你好鸭,june0620! @annepink给您叫了一份外卖! 由 @team-cn 新手村 迎着飓风 骑着村长家后院的鹿 给您送来 **烤肉** <br>  吃饱了吗?跟我猜拳吧! **石头,剪刀,布~** 如果您对我的服务满意,请不要吝啬您的点赞~ @onepagex
author | teamcn-shop |
---|---|
permlink | annepink-re-june0620-java-13-web-automation-13-selenium-with-testng-2-asserting-kr-20200310t131645972z |
category | hive-101145 |
json_metadata | "{"app":"teamcn-shop bot/1.0"}" |
created | 2020-03-10 13:16:57 |
last_update | 2020-03-10 13:16:57 |
depth | 1 |
children | 0 |
last_payout | 2020-03-17 13:16:57 |
cashout_time | 1969-12-31 23:59:59 |
total_payout_value | 0.000 HBD |
curator_payout_value | 0.000 HBD |
pending_payout_value | 0.000 HBD |
promoted | 0.000 HBD |
body_length | 219 |
author_reputation | 11,393,746,055,281 |
root_title | "[JAVA #13] [Web Automation #13] Selenium With TestNG #2 Asserting [KR]" |
beneficiaries | [] |
max_accepted_payout | 1,000,000.000 HBD |
percent_hbd | 10,000 |
post_id | 96,234,150 |
net_rshares | 0 |