In Part 2 we will install beem, a Hive module for Python, and get our hands on some Hive blockchain data. Let's go! This is the second post in the series about coding with Hive APIs and working with Hive data. If you want to run the sample code here, you will first need to install Python. A brief guide on how to do this is provided in [Part 1](https://peakd.com/programming/@learncode/part-1-coding-on-hive-with-python-setting-up). --- ## Installing Beem Module to Interact with Hive Now that you've installed Python, it's time to upgrade it by installing Hive modules. For now, all we need to install is ***[Beem](https://pypi.org/project/beem/)***. Beem is an awesome Python module created by @holger80. It's the "Unofficial Python Library for HIVE and STEEM". ***Beem*** also has excellent documentation here: https://beem.readthedocs.io/ Since we're using Python3 we already have everything we need to install modules. Like in part 1 where we invoked the Python interpreter for the first time, now we can call the same executable to fetch Beem. You can do this by entering the following command into your terminal: `python3 -m pip install beem`. This will download ***Beem*** and all of its dependencies (other modules that beem requires). On Windows the file name is slightly different and the command should instead be `python -m pip install beem` The next step is to fire up the Python interpreter and execute a line: `import beem`. If all goes well and the module is successfully imported, Python will say *nothing* and wait for the next line of input. --- ## Fetching Some Data from Hive Let's keep this simple for now. Hive is a social blockchain! Just a couple lines of code are needed to ask for my follower counts. First, create a beem *Account* object. Then call the object's `get_follow_count()` function. This call will make a network request to a Hivemind API node, and return the API response to our code. Copy-paste this into your Python interpreter terminal to try it out: ``` import beem account = beem.account.Account('learncode') account.get_follow_count() ``` The Python interpreter output looks like this: ``` >>> import beem >>> account = beem.account.Account('learncode') >>> account.get_follow_count() {'account': 'learncode', 'following_count': 0, 'follower_count': 3} ``` The return value of the `get_follow_count()` function is a dictionary. Dictionaries are Python's key-value data type. In this case, the dictionary has three keys (and three values). The response is self-explanatory: my account 'learncode' is following 0 other accounts and is followed by 3 other accounts. Try it yourself: replace `learncode` with your own account name in the example. --- ## Putting it All Together Part 2 explained how to install Python modules using **pip**, and how to import modules using the `import` keyword. Then, we used the **beem** module to fetch some data from the Hive blockchain. --- p.s. Thanks for reading. Please let me know if anything is unclear or incorrect. This is intended to be a living document to be improved and maintained over time. p.p.s This post is brought to you by the [Hive.Pizza team](https://hive.pizza).
author | learncode |
---|---|
permlink | part-2-coding-on-hive-with-python-installing-modules-and-fetching-some-data-from-hive |
category | programming |
json_metadata | "{"app":"peakd/2021.05.4","format":"markdown","description":"Installing the beem Python module and making a simple request to Hive API nodes for data","tags":["programming","coding","hive","python","beem","ctp","proofofbrain","stem","broadhive","leofinance"],"users":["learncode","holger80."],"image":[]}" |
created | 2021-06-01 05:00:39 |
last_update | 2021-06-03 18:37:36 |
depth | 0 |
children | 54 |
last_payout | 2021-06-08 05:00:39 |
cashout_time | 1969-12-31 23:59:59 |
total_payout_value | 1.808 HBD |
curator_payout_value | 1.791 HBD |
pending_payout_value | 0.000 HBD |
promoted | 0.000 HBD |
body_length | 3,184 |
author_reputation | 682,726,195,093 |
root_title | "Part 2: Coding on Hive With Python - Installing Modules and Fetching Some Data from Hive" |
beneficiaries | [] |
max_accepted_payout | 1,000,000.000 HBD |
percent_hbd | 0 |
post_id | 104,056,348 |
net_rshares | 6,253,640,533,266 |
author_curate_reward | "" |
voter | weight | wgt% | rshares | pct | time |
---|---|---|---|---|---|
good-karma | 0 | 24,976,542,674 | 2.62% | ||
steevc | 0 | 640,492,162,115 | 41% | ||
clayboyn | 0 | 33,667,402,547 | 25% | ||
slider2990 | 0 | 4,463,229,643 | 60% | ||
zorg67 | 0 | 642,001,321 | 100% | ||
edb | 0 | 28,926,407,508 | 20.5% | ||
esteemapp | 0 | 9,962,799,861 | 2.62% | ||
voter | 0 | 94,594,105 | 100% | ||
chasmic-cosm | 0 | 23,906,342,628 | 100% | ||
dickturpin | 0 | 6,646,065,994 | 20.5% | ||
skye1 | 0 | 1,985,461,144 | 50% | ||
rmsbodybuilding | 0 | 1,638,840,561 | 100% | ||
enjar | 0 | 778,553,619,581 | 100% | ||
vachemorte | 0 | 29,308,327,266 | 25% | ||
geekgirl | 0 | 1,614,814,956,364 | 100% | ||
juliakponsford | 0 | 371,533,387,452 | 50% | ||
auditoryorgasms | 0 | 689,497,897 | 25% | ||
sanat | 0 | 204,174,786 | 100% | ||
duekie | 0 | 822,427,679 | 100% | ||
esteem.app | 0 | 680,677,603 | 2.62% | ||
mytechtrail | 0 | 52,765,663,543 | 15% | ||
sentipl | 0 | 2,633,347,551 | 100% | ||
fourfourfun | 0 | 7,308,091,507 | 25% | ||
gillianpearce | 0 | 1,401,784,735 | 21% | ||
tonimontana | 0 | 7,371,970,962 | 100% | ||
bushradio | 0 | 2,521,239,699 | 25% | ||
therabbitzone | 0 | 852,013,817 | 100% | ||
achim03 | 0 | 119,007,422,553 | 50% | ||
yintercept | 0 | 143,988,631,171 | 100% | ||
holovision | 0 | 174,204,373,774 | 100% | ||
luppers | 0 | 14,144,019,454 | 1% | ||
skylinebuds | 0 | 97,713,196,832 | 100% | ||
bengiles | 0 | 758,779,014,794 | 100% | ||
cliffagreen | 0 | 6,555,651,888 | 50% | ||
misterc | 0 | 127,831,522,067 | 50% | ||
charitycurator | 0 | 5,128,793,482 | 10% | ||
vagabondspirit | 0 | 5,302,104,251 | 100% | ||
nftshowroom | 0 | 8,697,879,633 | 50% | ||
limka | 0 | 464,964,292 | 100% | ||
photographercr | 0 | 835,013,814 | 0.52% | ||
raspibot | 0 | 1,253,534,069 | 100% | ||
peachymod | 0 | 1,508,311,900 | 50% | ||
stemgeeks | 0 | 66,081,840,819 | 60% | ||
stemcuration | 0 | 2,075,966,646 | 60% | ||
babytarazkp | 0 | 4,400,184,184 | 40% | ||
abh12345.stem | 0 | 2,882,454,339 | 100% | ||
ph1102.ctp | 0 | 8,625,880,442 | 50% | ||
arslan.leo | 0 | 1,440,290,029 | 100% | ||
rootdraws | 0 | 5,330,256,329 | 50% | ||
yggdrasil.laguna | 0 | 125,678,964 | 30% | ||
toni.curation | 0 | 0 | 1% | ||
julesquirin | 0 | 1,744,109,094 | 10% | ||
stuntman.mike | 0 | 2,918,087,505 | 100% | ||
ecency | 0 | 924,836,077,970 | 2.62% | ||
localgrower | 0 | 1,711,007,272 | 10% | ||
p3ntar0u | 0 | 17,149,107,962 | 100% | ||
ecency.stats | 0 | 597,696,620 | 2.62% | ||
stickupboys | 0 | 11,480,947,791 | 28% | ||
focus.folks | 0 | 0 | 100% | ||
pfwaus | 0 | 1,234,706,890 | 100% | ||
dorkpower | 0 | 2,697,938,355 | 100% | ||
ermola | 0 | 285,012,042 | 100% | ||
schindmaehre | 0 | 188,815,753 | 100% | ||
brofund-stem | 0 | 769,505,871 | 30% | ||
rikarivka | 0 | 5,059,137,936 | 100% | ||
nathen007.stem | 0 | 0 | 100% | ||
stemline | 0 | 9,424,456,802 | 100% | ||
meestemboom | 0 | 957,950,897 | 50% | ||
emrebeyler.stem | 0 | 1,483,465,242 | 100% | ||
scientician | 0 | 90,407,209 | 100% | ||
scooter77.stem | 0 | 621,938,707 | 60% | ||
krishu.stem | 0 | 776,482,649 | 100% | ||
scholaris.stem | 0 | 1,396,419,096 | 100% | ||
damla.stem | 0 | 209,426,300 | 100% | ||
dannychain | 0 | 2,063,976,426 | 50% | ||
hypnochain | 0 | 46,252,495 | 50% | ||
adamada.stem | 0 | 569,731,487 | 100% | ||
huzzah | 0 | 30,600,938,878 | 100% | ||
skylinebuds-weed | 0 | 1,024,362,988 | 50% | ||
zdigital222 | 0 | 53,027,307 | 100% | ||
limn | 0 | 7,073,917,859 | 12.5% | ||
minus-pi | 0 | 3,783,202,742 | 100% | ||
nyxlabs | 0 | 3,101,802,181 | 50% | ||
laobin | 0 | 0 | 100% | ||
paoze | 0 | 0 | 100% | ||
lerkfrend | 0 | 2,985,286,582 | 72% | ||
jaxsonmurph | 0 | 735,641,116 | 36.6% | ||
sagardyola | 0 | 179,865,368 | 30% | ||
juecoree.stem | 0 | 532,662,175 | 100% | ||
watt.the.watt | 0 | 1,025,115,445 | 28% | ||
chireerocks.bht | 0 | 100,920,071 | 100% | ||
stickupmusic | 0 | 980,538,068 | 28% | ||
bellou61 | 0 | 1,134,451,704 | 28% | ||
holovision.stem | 0 | 0 | 50% | ||
tupack | 0 | 0 | 100% | ||
dibblers.dabs | 0 | 305,106,416 | 100% | ||
nfthypesquad | 0 | 850,904,292 | 10% | ||
cccf | 0 | 3,823,655,064 | 100% | ||
davemutawe | 0 | 0 | 100% | ||
star.stem | 0 | 1,796,460,370 | 50% | ||
ramon2024 | 0 | 0 | 100% | ||
b8l | 0 | 0 | 100% | ||
fefe99 | 0 | 0 | 100% |
Thanks a lot for this tutorial. Unfortunately I'm already stuck lol. I have python, visual studio installed. I have imported beem but when I run the code above in visual studio it gives me a lot of errors lol. <code> Error: Assert Exception:api_itr != _registered_apis.end(): Could not find API follow_api Lost connection or internal error on node: https://api.hive.blog (1/100) </code>
author | achim03 |
---|---|
permlink | re-learncode-qu131o |
category | programming |
json_metadata | {"tags":["programming"],"app":"peakd/2021.05.5"} |
created | 2021-06-01 15:01:03 |
last_update | 2021-06-01 15:01:03 |
depth | 1 |
children | 8 |
last_payout | 2021-06-08 15:01:03 |
cashout_time | 1969-12-31 23:59:59 |
total_payout_value | 0.013 HBD |
curator_payout_value | 0.000 HBD |
pending_payout_value | 0.000 HBD |
promoted | 0.000 HBD |
body_length | 389 |
author_reputation | 260,723,409,263,008 |
root_title | "Part 2: Coding on Hive With Python - Installing Modules and Fetching Some Data from Hive" |
beneficiaries | [] |
max_accepted_payout | 1,000,000.000 HBD |
percent_hbd | 10,000 |
post_id | 104,065,574 |
net_rshares | 68,571,912,675 |
author_curate_reward | "" |
voter | weight | wgt% | rshares | pct | time |
---|---|---|---|---|---|
torrey.blog | 0 | 68,571,912,675 | 100% |
Yes, I saw the same error. The API node beem tried to connect to doesnβt support the follow_api. Beem will retry other nodes until it finds one that works. To avoid hitting this error, you can specify a list of which API nodes Beem should use.
author | torrey.blog |
---|---|
permlink | re-achim03-qu13i7 |
category | programming |
json_metadata | {"tags":["programming"],"app":"peakd/2021.05.5"} |
created | 2021-06-01 15:10:57 |
last_update | 2021-06-01 15:10:57 |
depth | 2 |
children | 7 |
last_payout | 2021-06-08 15:10:57 |
cashout_time | 1969-12-31 23:59:59 |
total_payout_value | 0.000 HBD |
curator_payout_value | 0.000 HBD |
pending_payout_value | 0.000 HBD |
promoted | 0.000 HBD |
body_length | 244 |
author_reputation | 6,751,817,739,213 |
root_title | "Part 2: Coding on Hive With Python - Installing Modules and Fetching Some Data from Hive" |
beneficiaries | [] |
max_accepted_payout | 1,000,000.000 HBD |
percent_hbd | 10,000 |
post_id | 104,065,791 |
net_rshares | 0 |
Thanks now I have another error lol π€£. I really struggle with python :-)
author | achim03 |
---|---|
permlink | re-torreyblog-qu1dq1 |
category | programming |
json_metadata | {"tags":["programming"],"app":"peakd/2021.05.5"} |
created | 2021-06-01 18:51:39 |
last_update | 2021-06-01 18:52:12 |
depth | 3 |
children | 4 |
last_payout | 2021-06-08 18:51:39 |
cashout_time | 1969-12-31 23:59:59 |
total_payout_value | 0.000 HBD |
curator_payout_value | 0.000 HBD |
pending_payout_value | 0.000 HBD |
promoted | 0.000 HBD |
body_length | 72 |
author_reputation | 260,723,409,263,008 |
root_title | "Part 2: Coding on Hive With Python - Installing Modules and Fetching Some Data from Hive" |
beneficiaries | [] |
max_accepted_payout | 1,000,000.000 HBD |
percent_hbd | 10,000 |
post_id | 104,069,658 |
net_rshares | 0 |
Sorry, I had the same problem. How do we do that please?
author | nathen007.stem |
---|---|
permlink | qu15gs |
category | programming |
json_metadata | {"tags":["stem"],"app":"stemgeeks/0.1","canonical_url":"https://stemgeeks.net/@nathen007.stem/qu15gs"} |
created | 2021-06-01 15:53:18 |
last_update | 2021-06-01 15:53:18 |
depth | 3 |
children | 1 |
last_payout | 2021-06-08 15:53:18 |
cashout_time | 1969-12-31 23:59:59 |
total_payout_value | 0.000 HBD |
curator_payout_value | 0.000 HBD |
pending_payout_value | 0.000 HBD |
promoted | 0.000 HBD |
body_length | 56 |
author_reputation | 166,075,596,964 |
root_title | "Part 2: Coding on Hive With Python - Installing Modules and Fetching Some Data from Hive" |
beneficiaries | [] |
max_accepted_payout | 1,000,000.000 HBD |
percent_hbd | 10,000 |
post_id | 104,066,719 |
net_rshares | 1,254,097,046 |
author_curate_reward | "" |
voter | weight | wgt% | rshares | pct | time |
---|---|---|---|---|---|
amr008.stem | 0 | 1,254,097,046 | 100% |
That's a great tutorial! Very clearly presented, and I like how you've used the `get_follow_count()` function to explain python dictionaries. Keep up the good work! Posted Using [LeoFinance <sup>Beta</sup>](https://leofinance.io/@chasmic-cosm/re-learncode-5osdfn)
author | chasmic-cosm |
---|---|
permlink | re-learncode-5osdfn |
category | programming |
json_metadata | {"app":"leofinance/0.2","format":"markdown","tags":["programming","leofinance","hive-167922"],"canonical_url":"https://leofinance.io/@chasmic-cosm/re-learncode-5osdfn"} |
created | 2021-06-01 13:13:24 |
last_update | 2021-06-01 13:13:24 |
depth | 1 |
children | 1 |
last_payout | 2021-06-08 13:13:24 |
cashout_time | 1969-12-31 23:59:59 |
total_payout_value | 0.000 HBD |
curator_payout_value | 0.000 HBD |
pending_payout_value | 0.000 HBD |
promoted | 0.000 HBD |
body_length | 264 |
author_reputation | 4,159,775,155,640 |
root_title | "Part 2: Coding on Hive With Python - Installing Modules and Fetching Some Data from Hive" |
beneficiaries | [] |
max_accepted_payout | 1,000,000.000 HBD |
percent_hbd | 10,000 |
post_id | 104,063,618 |
net_rshares | 0 |
Thanks for reading and thanks for your support!
author | learncode |
---|---|
permlink | re-chasmic-cosm-qu15un |
category | programming |
json_metadata | {"tags":["programming"],"app":"peakd/2021.05.5"} |
created | 2021-06-01 16:01:36 |
last_update | 2021-06-01 16:01:36 |
depth | 2 |
children | 0 |
last_payout | 2021-06-08 16:01:36 |
cashout_time | 1969-12-31 23:59:59 |
total_payout_value | 0.000 HBD |
curator_payout_value | 0.000 HBD |
pending_payout_value | 0.000 HBD |
promoted | 0.000 HBD |
body_length | 47 |
author_reputation | 682,726,195,093 |
root_title | "Part 2: Coding on Hive With Python - Installing Modules and Fetching Some Data from Hive" |
beneficiaries | [] |
max_accepted_payout | 1,000,000.000 HBD |
percent_hbd | 10,000 |
post_id | 104,066,861 |
net_rshares | 23,106,756,598 |
author_curate_reward | "" |
voter | weight | wgt% | rshares | pct | time |
---|---|---|---|---|---|
chasmic-cosm | 0 | 23,106,756,598 | 100% |
Thanks for sharing. !PIZZA
author | cryptothesis |
---|---|
permlink | re-learncode-2022108t151645713z |
category | programming |
json_metadata | {"tags":["programming","coding","hive","python","beem","ctp","proofofbrain","stem","broadhive","leofinance"],"app":"ecency/3.0.34-mobile","format":"markdown+html"} |
created | 2022-10-08 07:16:45 |
last_update | 2022-10-08 07:16:45 |
depth | 1 |
children | 0 |
last_payout | 2022-10-15 07:16:45 |
cashout_time | 1969-12-31 23:59:59 |
total_payout_value | 0.000 HBD |
curator_payout_value | 0.000 HBD |
pending_payout_value | 0.000 HBD |
promoted | 0.000 HBD |
body_length | 28 |
author_reputation | 86,309,510,216,180 |
root_title | "Part 2: Coding on Hive With Python - Installing Modules and Fetching Some Data from Hive" |
beneficiaries | [] |
max_accepted_payout | 1,000,000.000 HBD |
percent_hbd | 10,000 |
post_id | 117,289,385 |
net_rshares | 0 |
I'll need to find out what beem does so I can either find a C# equivalent or make one.
author | dwinblood |
---|---|
permlink | re-learncode-qwf2wk |
category | programming |
json_metadata | {"tags":["programming"],"app":"peakd/2021.07.2"} |
created | 2021-07-18 01:31:33 |
last_update | 2021-07-18 01:31:33 |
depth | 1 |
children | 1 |
last_payout | 2021-07-25 01:31:33 |
cashout_time | 1969-12-31 23:59:59 |
total_payout_value | 0.000 HBD |
curator_payout_value | 0.000 HBD |
pending_payout_value | 0.000 HBD |
promoted | 0.000 HBD |
body_length | 86 |
author_reputation | 381,811,629,392,797 |
root_title | "Part 2: Coding on Hive With Python - Installing Modules and Fetching Some Data from Hive" |
beneficiaries | [] |
max_accepted_payout | 1,000,000.000 HBD |
percent_hbd | 10,000 |
post_id | 104,983,280 |
net_rshares | 0 |
Itβs just a convenient wrapper for Hive RPC API calls.
author | learncode |
---|---|
permlink | re-dwinblood-2021717t184311209z |
category | programming |
json_metadata | {"tags":["programming"],"app":"ecency/3.0.18-mobile","format":"markdown+html"} |
created | 2021-07-18 01:43:12 |
last_update | 2021-07-18 01:43:12 |
depth | 2 |
children | 0 |
last_payout | 2021-07-25 01:43:12 |
cashout_time | 1969-12-31 23:59:59 |
total_payout_value | 0.000 HBD |
curator_payout_value | 0.000 HBD |
pending_payout_value | 0.000 HBD |
promoted | 0.000 HBD |
body_length | 54 |
author_reputation | 682,726,195,093 |
root_title | "Part 2: Coding on Hive With Python - Installing Modules and Fetching Some Data from Hive" |
beneficiaries | [] |
max_accepted_payout | 1,000,000.000 HBD |
percent_hbd | 10,000 |
post_id | 104,983,380 |
net_rshares | 0 |
**Yay!** π€<br>Your content has been **boosted with Ecency Points**, by @torrey.blog. <br>Use Ecency daily to boost your growth on platform! <br><br><b>Support Ecency</b><br>[Vote for Proposal](https://hivesigner.com/sign/update-proposal-votes?proposal_ids=%5B141%5D&approve=true)<br>[Delegate HP and earn more](https://ecency.com/hive-125125/@ecency/daily-100-curation-rewards)
author | ecency |
---|---|
permlink | re-202162t22947682z |
category | programming |
json_metadata | {"tags":["ecency"],"app":"ecency/3.0.16-welcome","format":"markdown+html"} |
created | 2021-06-02 02:29:48 |
last_update | 2021-06-02 02:29:48 |
depth | 1 |
children | 0 |
last_payout | 2021-06-09 02:29:48 |
cashout_time | 1969-12-31 23:59:59 |
total_payout_value | 0.000 HBD |
curator_payout_value | 0.000 HBD |
pending_payout_value | 0.000 HBD |
promoted | 0.000 HBD |
body_length | 377 |
author_reputation | 549,971,524,037,747 |
root_title | "Part 2: Coding on Hive With Python - Installing Modules and Fetching Some Data from Hive" |
beneficiaries | [] |
max_accepted_payout | 1,000,000.000 HBD |
percent_hbd | 10,000 |
post_id | 104,076,474 |
net_rshares | 0 |
Step 2: check! ``` >>> account = beem.account.Account('fireguardian') >>> account.get_follow_count() {'account': 'fireguardian', 'follower_count': 629, 'following_count': 66}
author | fireguardian |
---|---|
permlink | re-learncode-qxjn2p |
category | programming |
json_metadata | {"tags":["programming"],"app":"peakd/2021.07.5"} |
created | 2021-08-08 23:11:18 |
last_update | 2021-08-08 23:11:18 |
depth | 1 |
children | 0 |
last_payout | 2021-08-15 23:11:18 |
cashout_time | 1969-12-31 23:59:59 |
total_payout_value | 0.000 HBD |
curator_payout_value | 0.000 HBD |
pending_payout_value | 0.000 HBD |
promoted | 0.000 HBD |
body_length | 177 |
author_reputation | 12,625,048,693,836 |
root_title | "Part 2: Coding on Hive With Python - Installing Modules and Fetching Some Data from Hive" |
beneficiaries | [] |
max_accepted_payout | 1,000,000.000 HBD |
percent_hbd | 10,000 |
post_id | 105,410,013 |
net_rshares | 0 |
Is the command prompt thing the "terminal"? When I paste "python3 -m pip install beem" I get the same result as I did in Lesson 1. Am I supposed to put cmd in front of "python3 -m pip install beem". It doesn't work either way. 😢 --- <center><sub>Posted via [proofofbrain.io](https://www.proofofbrain.io/@gillianpearce/qu4sp6)</sub></center>
author | gillianpearce |
---|---|
permlink | qu4sp6 |
category | programming |
json_metadata | {"tags":["proofofbrain"],"app":"proofofbrain/0.1","canonical_url":"https://www.proofofbrain.io/@gillianpearce/qu4sp6"} |
created | 2021-06-03 15:07:57 |
last_update | 2021-06-03 15:07:57 |
depth | 1 |
children | 11 |
last_payout | 2021-06-10 15:07:57 |
cashout_time | 1969-12-31 23:59:59 |
total_payout_value | 0.000 HBD |
curator_payout_value | 0.000 HBD |
pending_payout_value | 0.000 HBD |
promoted | 0.000 HBD |
body_length | 355 |
author_reputation | 51,237,062,991,594 |
root_title | "Part 2: Coding on Hive With Python - Installing Modules and Fetching Some Data from Hive" |
beneficiaries | [] |
max_accepted_payout | 1,000,000.000 HBD |
percent_hbd | 10,000 |
post_id | 104,106,872 |
net_rshares | 0 |
On Windows, the file naming is slightly different. Please instead try `python -m pip install beem`.
author | learncode |
---|---|
permlink | re-gillianpearce-202163t5368244z |
category | programming |
json_metadata | {"tags":["proofofbrain"],"app":"ecency/3.0.18-mobile","format":"markdown+html"} |
created | 2021-06-03 15:36:09 |
last_update | 2021-06-03 15:36:09 |
depth | 2 |
children | 10 |
last_payout | 2021-06-10 15:36:09 |
cashout_time | 1969-12-31 23:59:59 |
total_payout_value | 0.000 HBD |
curator_payout_value | 0.000 HBD |
pending_payout_value | 0.000 HBD |
promoted | 0.000 HBD |
body_length | 100 |
author_reputation | 682,726,195,093 |
root_title | "Part 2: Coding on Hive With Python - Installing Modules and Fetching Some Data from Hive" |
beneficiaries | [] |
max_accepted_payout | 1,000,000.000 HBD |
percent_hbd | 10,000 |
post_id | 104,107,313 |
net_rshares | 732,609,292 |
author_curate_reward | "" |
voter | weight | wgt% | rshares | pct | time |
---|---|---|---|---|---|
gillianpearce | 0 | 732,609,292 | 11% |
Yeah. I tried that too and got the same error as in Lesson 1, namely . . .  Posted Using [LeoFinance <sup>Beta</sup>](https://leofinance.io/@gillianpearce/re-learncode-t2nlp)
author | gillianpearce |
---|---|
permlink | re-learncode-t2nlp |
category | programming |
json_metadata | {"app":"leofinance/0.2","format":"markdown","tags":["programming","leofinance","hive-167922"],"canonical_url":"https://leofinance.io/@gillianpearce/re-learncode-t2nlp","image":["https://images.hive.blog/DQmaCQarAhT734bwKe7E3JwiCrSjFi5uBPztT5Wk5Hqc8AK/image.png"]} |
created | 2021-06-03 15:48:09 |
last_update | 2021-06-03 15:48:09 |
depth | 3 |
children | 9 |
last_payout | 2021-06-10 15:48:09 |
cashout_time | 1969-12-31 23:59:59 |
total_payout_value | 0.000 HBD |
curator_payout_value | 0.000 HBD |
pending_payout_value | 0.000 HBD |
promoted | 0.000 HBD |
body_length | 272 |
author_reputation | 51,237,062,991,594 |
root_title | "Part 2: Coding on Hive With Python - Installing Modules and Fetching Some Data from Hive" |
beneficiaries | [] |
max_accepted_payout | 1,000,000.000 HBD |
percent_hbd | 10,000 |
post_id | 104,107,524 |
net_rshares | 497,616,116 |
author_curate_reward | "" |
voter | weight | wgt% | rshares | pct | time |
---|---|---|---|---|---|
learncode | 0 | 497,616,116 | 100% |
I've tried to learn Python a few times without success. I'm hoping this time I'll be able to learn enough with your tutorials to get some data from the blockchain. Having help with a specific application should be easier. Here's hoping . . . Posted Using [LeoFinance <sup>Beta</sup>](https://leofinance.io/@gillianpearce/re-learncode-2tjgd3)
author | gillianpearce |
---|---|
permlink | re-learncode-2tjgd3 |
category | programming |
json_metadata | {"app":"leofinance/0.2","format":"markdown","tags":["programming","leofinance","hive-167922"],"canonical_url":"https://leofinance.io/@gillianpearce/re-learncode-2tjgd3"} |
created | 2021-06-01 16:19:57 |
last_update | 2021-06-01 16:19:57 |
depth | 1 |
children | 5 |
last_payout | 2021-06-08 16:19:57 |
cashout_time | 1969-12-31 23:59:59 |
total_payout_value | 0.000 HBD |
curator_payout_value | 0.000 HBD |
pending_payout_value | 0.000 HBD |
promoted | 0.000 HBD |
body_length | 342 |
author_reputation | 51,237,062,991,594 |
root_title | "Part 2: Coding on Hive With Python - Installing Modules and Fetching Some Data from Hive" |
beneficiaries | [] |
max_accepted_payout | 1,000,000.000 HBD |
percent_hbd | 10,000 |
post_id | 104,067,146 |
net_rshares | 0 |
Hi Gillian, glad you found me. If you donβt mind my asking, where did you get hung up before? I will try to cover those concepts here to cover the gaps.
author | learncode |
---|---|
permlink | re-gillianpearce-202161t63455655z |
category | programming |
json_metadata | {"tags":["programming","leofinance","hive-167922"],"app":"ecency/3.0.18-mobile","format":"markdown+html"} |
created | 2021-06-01 16:34:57 |
last_update | 2021-06-01 16:34:57 |
depth | 2 |
children | 4 |
last_payout | 2021-06-08 16:34:57 |
cashout_time | 1969-12-31 23:59:59 |
total_payout_value | 0.000 HBD |
curator_payout_value | 0.000 HBD |
pending_payout_value | 0.000 HBD |
promoted | 0.000 HBD |
body_length | 154 |
author_reputation | 682,726,195,093 |
root_title | "Part 2: Coding on Hive With Python - Installing Modules and Fetching Some Data from Hive" |
beneficiaries | [] |
max_accepted_payout | 1,000,000.000 HBD |
percent_hbd | 10,000 |
post_id | 104,067,384 |
net_rshares | 1,350,138,913 |
author_curate_reward | "" |
voter | weight | wgt% | rshares | pct | time |
---|---|---|---|---|---|
gillianpearce | 0 | 1,350,138,913 | 19% |
>where did you get hung up before I can't remember the specifics. I just found it hard learning stuff when it wasn't applied to a problem that I actually wanted to solve. Primarily I want to be able to download my data with dates and amounts etc of the various tokens in a csv friendly format to make filing taxes easier. I'm assuming that's possible with python but I could be wrong. 😊 Posted Using [LeoFinance <sup>Beta</sup>](https://leofinance.io/@gillianpearce/re-learncode-6lkjve)
author | gillianpearce |
---|---|
permlink | re-learncode-6lkjve |
category | programming |
json_metadata | {"app":"leofinance/0.2","format":"markdown","tags":["programming","leofinance","hive-167922"],"canonical_url":"https://leofinance.io/@gillianpearce/re-learncode-6lkjve"} |
created | 2021-06-02 09:20:45 |
last_update | 2021-06-02 09:20:45 |
depth | 3 |
children | 3 |
last_payout | 2021-06-09 09:20:45 |
cashout_time | 1969-12-31 23:59:59 |
total_payout_value | 0.000 HBD |
curator_payout_value | 0.000 HBD |
pending_payout_value | 0.000 HBD |
promoted | 0.000 HBD |
body_length | 498 |
author_reputation | 51,237,062,991,594 |
root_title | "Part 2: Coding on Hive With Python - Installing Modules and Fetching Some Data from Hive" |
beneficiaries | [] |
max_accepted_payout | 1,000,000.000 HBD |
percent_hbd | 10,000 |
post_id | 104,080,816 |
net_rshares | 0 |
Congratulations @learncode! You have completed the following achievement on the Hive blockchain and have been rewarded with new badge(s) : <table><tr><td><img src="https://images.hive.blog/60x70/http://hivebuzz.me/@learncode/upvoted.png?202106010901"></td><td>You received more than 50 upvotes.<br>Your next target is to reach 100 upvotes.</td></tr> </table> <sub>_You can view your badges on [your board](https://hivebuzz.me/@learncode) and compare yourself to others in the [Ranking](https://hivebuzz.me/ranking)_</sub> <sub>_If you no longer want to receive notifications, reply to this comment with the word_ `STOP`</sub> **Check out the last post from @hivebuzz:** <table><tr><td><a href="/hivebuzz/@hivebuzz/pud-202106"><img src="https://images.hive.blog/64x128/https://i.imgur.com/805FIIt.jpg"></a></td><td><a href="/hivebuzz/@hivebuzz/pud-202106">Hive Power Up Day - June 1st 2021 - Hive Power Delegation</a></td></tr></table> ###### Support the HiveBuzz project. [Vote](https://hivesigner.com/sign/update_proposal_votes?proposal_ids=%5B%22109%22%5D&approve=true) for [our proposal](https://peakd.com/me/proposals/147)!
author | hivebuzz |
---|---|
permlink | hivebuzz-notify-learncode-20210601t091247000z |
category | programming |
json_metadata | {"image":["http://hivebuzz.me/notify.t6.png"]} |
created | 2021-06-01 09:12:45 |
last_update | 2021-06-01 09:12:45 |
depth | 1 |
children | 0 |
last_payout | 2021-06-08 09:12:45 |
cashout_time | 1969-12-31 23:59:59 |
total_payout_value | 0.000 HBD |
curator_payout_value | 0.000 HBD |
pending_payout_value | 0.000 HBD |
promoted | 0.000 HBD |
body_length | 1,133 |
author_reputation | 367,965,646,463,563 |
root_title | "Part 2: Coding on Hive With Python - Installing Modules and Fetching Some Data from Hive" |
beneficiaries | [] |
max_accepted_payout | 1,000,000.000 HBD |
percent_hbd | 10,000 |
post_id | 104,060,239 |
net_rshares | 0 |
Thanks for your write up. I'm running into issues getting beem installed, hope you can help. This is on my Windows 10 system. Thanks. * crypto_aes.c scrypt-1.2.1/libcperciva/crypto/crypto_aes.c(6): fatal error C1083: Cannot open include file: 'openssl/aes.h': No such file or directory error: command 'C:\\Program Files (x86)\\Microsoft Visual Studio 14.0\\VC\\BIN\\x86_amd64\\cl.exe' failed with exit code 2 [end of output] note: This error originates from a subprocess, and is likely not a problem with pip. ERROR: Failed building wheel for scrypt Failed to build scrypt ERROR: Could not build wheels for scrypt, which is required to install pyproject.toml-based projects*
author | mindblaster |
---|---|
permlink | re-learncode-r9a514 |
category | programming |
json_metadata | {"tags":["programming"],"app":"peakd/2022.03.7"} |
created | 2022-03-25 02:46:24 |
last_update | 2022-03-25 02:46:24 |
depth | 1 |
children | 2 |
last_payout | 2022-04-01 02:46:24 |
cashout_time | 1969-12-31 23:59:59 |
total_payout_value | 0.000 HBD |
curator_payout_value | 0.000 HBD |
pending_payout_value | 0.000 HBD |
promoted | 0.000 HBD |
body_length | 705 |
author_reputation | 1,951,411,193 |
root_title | "Part 2: Coding on Hive With Python - Installing Modules and Fetching Some Data from Hive" |
beneficiaries | [] |
max_accepted_payout | 1,000,000.000 HBD |
percent_hbd | 10,000 |
post_id | 111,665,876 |
net_rshares | 0 |
Do you find a solution? I have the same problem
author | quekery |
---|---|
permlink | re-mindblaster-rdxulm |
category | programming |
json_metadata | {"tags":["programming"],"app":"peakd/2022.05.9"} |
created | 2022-06-23 16:17:45 |
last_update | 2022-06-23 16:17:45 |
depth | 2 |
children | 0 |
last_payout | 2022-06-30 16:17:45 |
cashout_time | 1969-12-31 23:59:59 |
total_payout_value | 0.020 HBD |
curator_payout_value | 0.021 HBD |
pending_payout_value | 0.000 HBD |
promoted | 0.000 HBD |
body_length | 49 |
author_reputation | 69,685,398,785,959 |
root_title | "Part 2: Coding on Hive With Python - Installing Modules and Fetching Some Data from Hive" |
beneficiaries | [] |
max_accepted_payout | 1,000,000.000 HBD |
percent_hbd | 10,000 |
post_id | 114,272,371 |
net_rshares | 57,655,630,225 |
author_curate_reward | "" |
voter | weight | wgt% | rshares | pct | time |
---|---|---|---|---|---|
stimialiti | 0 | 7,175,449,853 | 30% | ||
dustsweeper | 0 | 50,480,180,372 | 6.75% |
Find one! Just install [openSSL](https://slproweb.com/products/Win32OpenSSL.html)
author | quekery |
---|---|
permlink | re-mindblaster-rdy17c |
category | programming |
json_metadata | {"tags":["programming"],"app":"peakd/2022.05.9"} |
created | 2022-06-23 18:40:24 |
last_update | 2022-06-23 18:40:24 |
depth | 2 |
children | 0 |
last_payout | 2022-06-30 18:40:24 |
cashout_time | 1969-12-31 23:59:59 |
total_payout_value | 0.000 HBD |
curator_payout_value | 0.000 HBD |
pending_payout_value | 0.000 HBD |
promoted | 0.000 HBD |
body_length | 82 |
author_reputation | 69,685,398,785,959 |
root_title | "Part 2: Coding on Hive With Python - Installing Modules and Fetching Some Data from Hive" |
beneficiaries | [] |
max_accepted_payout | 1,000,000.000 HBD |
percent_hbd | 10,000 |
post_id | 114,275,862 |
net_rshares | 0 |
If you post from stemgeeks.net you will earn extra STEM tokens.
author | mytechtrail |
---|---|
permlink | re-learncode-qub9p0 |
category | programming |
json_metadata | {"tags":["programming"],"app":"peakd/2021.05.5"} |
created | 2021-06-07 03:00:36 |
last_update | 2021-06-07 03:00:36 |
depth | 1 |
children | 1 |
last_payout | 2021-06-14 03:00:36 |
cashout_time | 1969-12-31 23:59:59 |
total_payout_value | 0.000 HBD |
curator_payout_value | 0.000 HBD |
pending_payout_value | 0.000 HBD |
promoted | 0.000 HBD |
body_length | 64 |
author_reputation | 18,957,346,112,072 |
root_title | "Part 2: Coding on Hive With Python - Installing Modules and Fetching Some Data from Hive" |
beneficiaries | [] |
max_accepted_payout | 1,000,000.000 HBD |
percent_hbd | 10,000 |
post_id | 104,178,082 |
net_rshares | 0 |
Thanks for the tip!
author | learncode |
---|---|
permlink | re-mytechtrail-202166t20517881z |
category | programming |
json_metadata | {"tags":["programming"],"app":"ecency/3.0.18-mobile","format":"markdown+html"} |
created | 2021-06-07 03:05:18 |
last_update | 2021-06-07 03:05:18 |
depth | 2 |
children | 0 |
last_payout | 2021-06-14 03:05:18 |
cashout_time | 1969-12-31 23:59:59 |
total_payout_value | 0.000 HBD |
curator_payout_value | 0.000 HBD |
pending_payout_value | 0.000 HBD |
promoted | 0.000 HBD |
body_length | 19 |
author_reputation | 682,726,195,093 |
root_title | "Part 2: Coding on Hive With Python - Installing Modules and Fetching Some Data from Hive" |
beneficiaries | [] |
max_accepted_payout | 1,000,000.000 HBD |
percent_hbd | 10,000 |
post_id | 104,178,316 |
net_rshares | 0 |
<center>PIZZA! PIZZA Holders sent <strong>$PIZZA</strong> tips in this post's comments: @cryptothesis<sub>(2/10)</sub> tipped @learncode (x1) <sub>Join us in <a href="https://discord.gg/hivepizza">Discord</a>!</sub></center>
author | pizzabot |
---|---|
permlink | re-part-2-coding-on-hive-with-python-installing-modules-and-fetching-some-data-from-hive-20221008t071754z |
category | programming |
json_metadata | "{"app": "beem/0.24.19"}" |
created | 2022-10-08 07:17:54 |
last_update | 2022-10-08 07:17:54 |
depth | 1 |
children | 0 |
last_payout | 2022-10-15 07:17:54 |
cashout_time | 1969-12-31 23:59:59 |
total_payout_value | 0.000 HBD |
curator_payout_value | 0.000 HBD |
pending_payout_value | 0.000 HBD |
promoted | 0.000 HBD |
body_length | 228 |
author_reputation | 6,161,089,492,455 |
root_title | "Part 2: Coding on Hive With Python - Installing Modules and Fetching Some Data from Hive" |
beneficiaries | [] |
max_accepted_payout | 1,000,000.000 HBD |
percent_hbd | 10,000 |
post_id | 117,289,400 |
net_rshares | 0 |
How can I fetch other data like mana of an account?
author | princekham |
---|---|
permlink | re-learncode-2023729t1805884z |
category | programming |
json_metadata | {"tags":["programming","coding","hive","python","beem","ctp","proofofbrain","stem","broadhive","leofinance"],"app":"ecency/3.0.35-vision","format":"markdown+html"} |
created | 2023-07-29 11:30:57 |
last_update | 2023-07-29 11:30:57 |
depth | 1 |
children | 0 |
last_payout | 2023-08-05 11:30:57 |
cashout_time | 1969-12-31 23:59:59 |
total_payout_value | 0.000 HBD |
curator_payout_value | 0.000 HBD |
pending_payout_value | 0.000 HBD |
promoted | 0.000 HBD |
body_length | 52 |
author_reputation | 88,012,342,837,339 |
root_title | "Part 2: Coding on Hive With Python - Installing Modules and Fetching Some Data from Hive" |
beneficiaries | [] |
max_accepted_payout | 1,000,000.000 HBD |
percent_hbd | 10,000 |
post_id | 125,743,437 |
net_rshares | 0 |
So in the film you are making is it like giant snakes vs bees?
author | stickupboys |
---|---|
permlink | re-learncode-qu1qs5 |
category | programming |
json_metadata | {"tags":["programming"],"app":"peakd/2021.05.5"} |
created | 2021-06-01 23:33:39 |
last_update | 2021-06-01 23:33:39 |
depth | 1 |
children | 5 |
last_payout | 2021-06-08 23:33:39 |
cashout_time | 1969-12-31 23:59:59 |
total_payout_value | 0.018 HBD |
curator_payout_value | 0.018 HBD |
pending_payout_value | 0.000 HBD |
promoted | 0.000 HBD |
body_length | 62 |
author_reputation | 409,258,469,233,840 |
root_title | "Part 2: Coding on Hive With Python - Installing Modules and Fetching Some Data from Hive" |
beneficiaries | [] |
max_accepted_payout | 1,000,000.000 HBD |
percent_hbd | 10,000 |
post_id | 104,074,008 |
net_rshares | 92,933,723,732 |
author_curate_reward | "" |
voter | weight | wgt% | rshares | pct | time |
---|---|---|---|---|---|
steevc | 0 | 92,933,723,732 | 6% |
You lost me mate!
author | learncode |
---|---|
permlink | re-stickupboys-202161t133529828z |
category | programming |
json_metadata | {"tags":["programming"],"app":"ecency/3.0.18-mobile","format":"markdown+html"} |
created | 2021-06-01 23:35:30 |
last_update | 2021-06-01 23:35:30 |
depth | 2 |
children | 4 |
last_payout | 2021-06-08 23:35:30 |
cashout_time | 1969-12-31 23:59:59 |
total_payout_value | 0.000 HBD |
curator_payout_value | 0.000 HBD |
pending_payout_value | 0.000 HBD |
promoted | 0.000 HBD |
body_length | 17 |
author_reputation | 682,726,195,093 |
root_title | "Part 2: Coding on Hive With Python - Installing Modules and Fetching Some Data from Hive" |
beneficiaries | [] |
max_accepted_payout | 1,000,000.000 HBD |
percent_hbd | 10,000 |
post_id | 104,074,042 |
net_rshares | 17,455,518,618 |
author_curate_reward | "" |
voter | weight | wgt% | rshares | pct | time |
---|---|---|---|---|---|
stickupboys | 0 | 17,455,518,618 | 43% |
You lost me with the title....lol bees...snakes....animals....no? I don't know any coding jokes.... 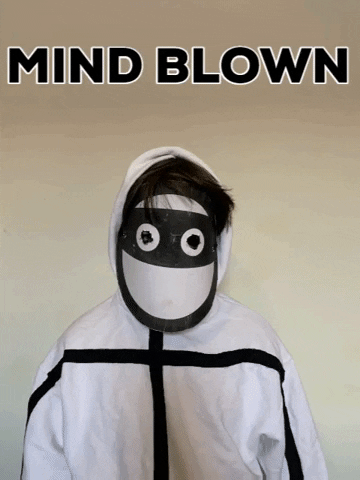
author | stickupboys |
---|---|
permlink | re-learncode-qu1r5j |
category | programming |
json_metadata | {"tags":["programming"],"app":"peakd/2021.05.5"} |
created | 2021-06-01 23:41:42 |
last_update | 2021-06-01 23:41:42 |
depth | 3 |
children | 3 |
last_payout | 2021-06-08 23:41:42 |
cashout_time | 1969-12-31 23:59:59 |
total_payout_value | 0.000 HBD |
curator_payout_value | 0.000 HBD |
pending_payout_value | 0.000 HBD |
promoted | 0.000 HBD |
body_length | 259 |
author_reputation | 409,258,469,233,840 |
root_title | "Part 2: Coding on Hive With Python - Installing Modules and Fetching Some Data from Hive" |
beneficiaries | [] |
max_accepted_payout | 1,000,000.000 HBD |
percent_hbd | 10,000 |
post_id | 104,074,134 |
net_rshares | 0 |
That documentation link was extremely nice. I had tried to learn beem without any documentation in the past. I figured out how to query information, but I never figured out how to execute transactions. !wine
author | yintercept |
---|---|
permlink | qu1djo |
category | programming |
json_metadata | {"app":"hiveblog/0.1"} |
created | 2021-06-01 18:47:51 |
last_update | 2021-06-01 18:47:51 |
depth | 1 |
children | 1 |
last_payout | 2021-06-08 18:47:51 |
cashout_time | 1969-12-31 23:59:59 |
total_payout_value | 0.032 HBD |
curator_payout_value | 0.033 HBD |
pending_payout_value | 0.000 HBD |
promoted | 0.000 HBD |
body_length | 210 |
author_reputation | 23,962,343,431,134 |
root_title | "Part 2: Coding on Hive With Python - Installing Modules and Fetching Some Data from Hive" |
beneficiaries | [] |
max_accepted_payout | 1,000,000.000 HBD |
percent_hbd | 10,000 |
post_id | 104,069,584 |
net_rshares | 164,302,627,015 |
author_curate_reward | "" |
voter | weight | wgt% | rshares | pct | time |
---|---|---|---|---|---|
yintercept | 0 | 158,221,336,305 | 100% | ||
vagabondspirit | 0 | 5,577,340,981 | 100% | ||
learncode | 0 | 503,949,729 | 100% |
Happy to help! Since this example did some reading data from Hive, Iβm thinking the next one will write some data to Hive. So you can see how to set up the keys to make transactions.
author | learncode |
---|---|
permlink | re-yintercept-202161t13649319z |
category | programming |
json_metadata | {"tags":["ecency"],"app":"ecency/3.0.18-mobile","format":"markdown+html"} |
created | 2021-06-01 23:06:48 |
last_update | 2021-06-01 23:06:48 |
depth | 2 |
children | 0 |
last_payout | 2021-06-08 23:06:48 |
cashout_time | 1969-12-31 23:59:59 |
total_payout_value | 0.030 HBD |
curator_payout_value | 0.030 HBD |
pending_payout_value | 0.000 HBD |
promoted | 0.000 HBD |
body_length | 182 |
author_reputation | 682,726,195,093 |
root_title | "Part 2: Coding on Hive With Python - Installing Modules and Fetching Some Data from Hive" |
beneficiaries | [] |
max_accepted_payout | 1,000,000.000 HBD |
percent_hbd | 10,000 |
post_id | 104,073,611 |
net_rshares | 151,485,910,643 |
author_curate_reward | "" |
voter | weight | wgt% | rshares | pct | time |
---|---|---|---|---|---|
yintercept | 0 | 151,485,910,643 | 100% |
After installing Beem I saw this message on the terminal: >WARNING: The script beempy is installed in '/home/user/.local/bin' which is not on PATH. Consider adding this directory to PATH or, if you prefer to suppress this warning, use --no-warn-script-location Do you know what this means? I replicated the example that you provided with no issues but I don't know what that warning means.
author | zorg67 |
---|---|
permlink | re-learncode-qu1i5k |
category | programming |
json_metadata | {"tags":["programming"],"app":"peakd/2021.05.5"} |
created | 2021-06-01 20:27:39 |
last_update | 2021-06-01 20:27:39 |
depth | 1 |
children | 3 |
last_payout | 2021-06-08 20:27:39 |
cashout_time | 1969-12-31 23:59:59 |
total_payout_value | 0.000 HBD |
curator_payout_value | 0.000 HBD |
pending_payout_value | 0.000 HBD |
promoted | 0.000 HBD |
body_length | 393 |
author_reputation | 384,908,390,168 |
root_title | "Part 2: Coding on Hive With Python - Installing Modules and Fetching Some Data from Hive" |
beneficiaries | [] |
max_accepted_payout | 1,000,000.000 HBD |
percent_hbd | 10,000 |
post_id | 104,071,245 |
net_rshares | 0 |
Beem also has a command line executable you can call directly instead of via Python script. The warning is telling you if you try to invoke `beempy` from your terminal, the command wonβt be found. You will need to append the path to your PATH environment variable, or use the full path.
author | learncode |
---|---|
permlink | re-zorg67-202161t13926258z |
category | programming |
json_metadata | {"tags":["programming"],"app":"ecency/3.0.18-mobile","format":"markdown+html"} |
created | 2021-06-01 23:09:27 |
last_update | 2021-06-01 23:09:27 |
depth | 2 |
children | 2 |
last_payout | 2021-06-08 23:09:27 |
cashout_time | 1969-12-31 23:59:59 |
total_payout_value | 0.000 HBD |
curator_payout_value | 0.000 HBD |
pending_payout_value | 0.000 HBD |
promoted | 0.000 HBD |
body_length | 286 |
author_reputation | 682,726,195,093 |
root_title | "Part 2: Coding on Hive With Python - Installing Modules and Fetching Some Data from Hive" |
beneficiaries | [] |
max_accepted_payout | 1,000,000.000 HBD |
percent_hbd | 10,000 |
post_id | 104,073,646 |
net_rshares | 0 |
Thank you for the response, I had already figured it out and appended the path to the PATH environment. I learned something about linux in the meantime :)
author | zorg67 |
---|---|
permlink | re-learncode-quak0h |
category | programming |
json_metadata | {"tags":["programming"],"app":"peakd/2021.05.5"} |
created | 2021-06-06 17:45:57 |
last_update | 2021-06-06 17:45:57 |
depth | 3 |
children | 1 |
last_payout | 2021-06-13 17:45:57 |
cashout_time | 1969-12-31 23:59:59 |
total_payout_value | 0.000 HBD |
curator_payout_value | 0.000 HBD |
pending_payout_value | 0.000 HBD |
promoted | 0.000 HBD |
body_length | 154 |
author_reputation | 384,908,390,168 |
root_title | "Part 2: Coding on Hive With Python - Installing Modules and Fetching Some Data from Hive" |
beneficiaries | [] |
max_accepted_payout | 1,000,000.000 HBD |
percent_hbd | 10,000 |
post_id | 104,170,774 |
net_rshares | 613,620,161 |
author_curate_reward | "" |
voter | weight | wgt% | rshares | pct | time |
---|---|---|---|---|---|
learncode | 0 | 613,620,161 | 100% |