Greetings to my favorite science community online, StemSocial. It's @skyehi and I'm really excited to be back to continue my series on Android App development tutorials for beginners. Yesterday's tutorial on building our first News Reader App got quite a lot of attention and I'm grateful to both StemSocial and @empo.voter for all the support. > Because I'm so happy about yesterday's post success, I've decided to make more like a bonus tutorial. We'll be building our first Android Game App. This would be a basic dice rolling game. There are a lot of dice rolling games where the user presses a "Roll dice" button and gets a number. Some game developers use it for developing Play-to-earn games or gambling sites. Site's like freebitco and other crypto faucet P2E games use the Rolling Dice technique to build their games and platforms. > Ever wondered how they did it?. Well we'll be developing a dice roller game and you'll see exactly how that works. 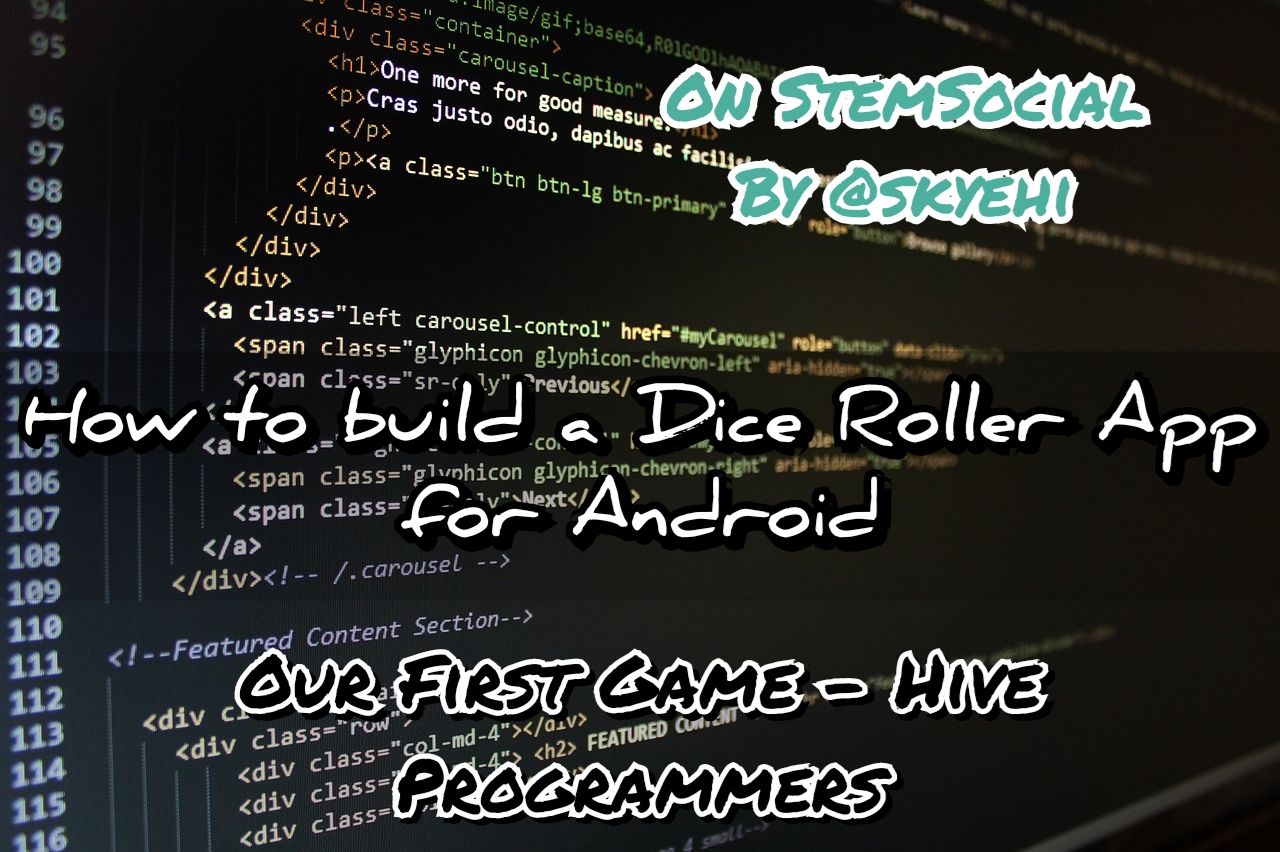[Original Image Source](https://pixabay.com/photos/website-code-html-coding-647013/) by lmonk72 from Pixabay  ### Prerequisites For the sake of all the newcomers to my series, before you can build an Android app, you would need to make sure you have Android Studio and Java Development Kit, JDK installed on your computer. Here's the link to the main download page. You can download the software from [Android Developer website](https://developer.android.com/studio). If you're having any issue downloading or installing both softwares on your computer, please let me know in the comments guys. ### Creating a new Android Studio Project At this point, I'll assume that my readers have successfully installed Android Studio IDE. The first thing we need to do is to create a new Android Studio Project. We'll do that by opening Android Studio and clicking on "Start a new Android Studio project" On the next page, you will be able to create a name for your App and choose the package name. I would also recommend that you choose Java as the programming language because that's what I'll be using for the beginner's tutorial series. As we keep making progress in our series, I'll start using Kotlin which would allow us to create simpler Apps with less bugs. Please choose "Empty Activity" as the template to make developing the game simpler.  ### Designing the Layout Now that we're all set with creating a new Android studio Project for our app, it's time to work on the frontend design. This is the part that the user will see and interact with. The layout design of our app will be done inside the `res/layout/activity_main.xml` file. We will be creating a simple dice roller app so we will only need a Button the user would use to roll the dice and a TextView to display the result. > Here's how your code should look like; ```xml <?xml version="1.0" encoding="utf-8"?> <RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" tools:context=".MainActivity"> <Button android:id="@+id/rollButton" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="Roll Dice" android:layout_centerInParent="true"/> <TextView android:id="@+id/resultTextView" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="Result will be displayed here." android:layout_below="@id/rollButton" android:layout_marginTop="16dp" android:layout_centerHorizontal="true"/> </RelativeLayout> ``` ### Creating the logic of our game Our Dice app would have random numbers from "1" to "6". When the user presses the button, any of those numbers can be shown as a result. In more advanced projects, we would create a reward system where the user can earn points based on the results of the dice rolling. It's a really basic code and after finishing our project, I'm hopeful that you'll be proud of your first Android dice game. The logic code of our app will be written inside `MainActivity.java` > Here's how your code should look like guys. ```java import android.os.Bundle; import android.view.View; import android.widget.Button; import android.widget.TextView; import androidx.appcompat.app.AppCompatActivity; import java.util.Random; public class MainActivity extends AppCompatActivity { private Button rollButton; private TextView resultTextView; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); rollButton = findViewById(R.id.rollButton); resultTextView = findViewById(R.id.resultTextView); rollButton.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View view) { rollDice(); } }); } private void rollDice() { Random random = new Random(); int diceResult = random.nextInt(6) + 1; // Generate a random number between 1 and 6 resultTextView.setText("Dice Result: " + diceResult); } } ```  ### Running the Dice Roller Game Congratulations guys, we just finished building a great and simple Dice Roller App. It's now time to see the results and play the game. To run the app, you can either connect your Android device or use an emulator. Like I always say, I personally prefer running my Apps on my physical Android device so I'll see how the App would look on an actual phone. Please do not forget to turn on USB debugging in order to be able to run the App on your device. When the app launches, click the "Roll Dice" button, and you should see the result displayed in the TextView.  Thank you so much for taking the time to read today's tutorial blog. I hope you enjoyed today's tutorial guys. We are gradually make progress and we'll soon build more complex Apps that you'll be proud of. As always guys, if you're having troubles writing the code, installing the IDE or JDK or even running the App, please let me know in the comments section and I'll be of help. > Have a lovely day and catch you next time on StemSocial. Goodbye ✍️ <center> You Can Follow Me @skyehi For More Like This And Others </center>
author | skyehi | ||||||
---|---|---|---|---|---|---|---|
permlink | how-to-build-a-dice-roller-app-for-android-our-first-game-hive-programmers | ||||||
category | hive-196387 | ||||||
json_metadata | {"app":"peakd/2023.11.3","format":"markdown","tags":["health","stemng","stemgeeks","neoxian","waivio","cent","proofofbrain","chessbrothers","alive","stemsocial"],"users":["skyehi","empo.voter","Override"],"image":["https://files.peakd.com/file/peakd-hive/skyehi/23tbPiVkwLBkHxkt3nZRofynPNDxRBLN1xbafFoXuAN87YLReVGXHiXzhuHkVjKyECHqF.jpg","https://files.peakd.com/file/peakd-hive/skyehi/23swrbKc3yjMYSUJMwmHkXUC71AufZXrkQ315XAST7nXzaKhpkvsAScnV3nBnpS55SJ2r.png","https://files.peakd.com/file/peakd-hive/skyehi/EoAYDi9ZKfkBZi7rMWbojnS8KytoTpbSepvPmfeZmL2V2h8FDZ3xRpC58MZDkf1LeRQ.png"]} | ||||||
created | 2023-11-30 15:23:57 | ||||||
last_update | 2023-11-30 15:23:57 | ||||||
depth | 0 | ||||||
children | 2 | ||||||
last_payout | 2023-12-07 15:23:57 | ||||||
cashout_time | 1969-12-31 23:59:59 | ||||||
total_payout_value | 1.130 HBD | ||||||
curator_payout_value | 1.149 HBD | ||||||
pending_payout_value | 0.000 HBD | ||||||
promoted | 0.000 HBD | ||||||
body_length | 7,759 | ||||||
author_reputation | 103,283,026,686,970 | ||||||
root_title | "How to build a Dice Roller App for Android: Our First Game - Hive Programmers" | ||||||
beneficiaries |
| ||||||
max_accepted_payout | 1,000,000.000 HBD | ||||||
percent_hbd | 10,000 | ||||||
post_id | 129,297,761 | ||||||
net_rshares | 4,969,073,835,672 | ||||||
author_curate_reward | "" |
voter | weight | wgt% | rshares | pct | time |
---|---|---|---|---|---|
kevinwong | 0 | 3,793,393,681 | 0.6% | ||
eric-boucher | 0 | 3,110,033,455 | 0.6% | ||
thecryptodrive | 0 | 14,234,366,426 | 0.24% | ||
roelandp | 0 | 60,143,589,815 | 7.5% | ||
cloh76 | 0 | 799,182,537 | 0.6% | ||
lichtblick | 0 | 511,468,396 | 0.36% | ||
lordvader | 0 | 7,060,175,368 | 1.2% | ||
lemouth | 0 | 395,998,021,607 | 15% | ||
lamouthe | 0 | 1,139,412,775 | 15% | ||
tfeldman | 0 | 1,103,915,959 | 0.6% | ||
metabs | 0 | 1,637,135,679 | 15% | ||
mcsvi | 0 | 135,765,316,294 | 50% | ||
cnfund | 0 | 2,376,897,577 | 1.2% | ||
justyy | 0 | 5,682,697,390 | 1.2% | ||
michelle.gent | 0 | 685,580,225 | 0.24% | ||
curie | 0 | 68,687,464,789 | 1.2% | ||
modernzorker | 0 | 743,928,305 | 0.84% | ||
techslut | 0 | 39,837,353,051 | 6% | ||
steemstem | 0 | 272,966,747,550 | 15% | ||
walterjay | 0 | 93,693,300,076 | 7.5% | ||
metroair | 0 | 4,561,804,075 | 1.2% | ||
dna-replication | 0 | 540,970,899 | 15% | ||
dhimmel | 0 | 77,207,130,932 | 3.75% | ||
oluwatobiloba | 0 | 474,858,398 | 15% | ||
detlev | 0 | 2,994,009,893 | 0.36% | ||
federacion45 | 0 | 1,742,177,292 | 0.6% | ||
gamersclassified | 0 | 930,624,352 | 0.6% | ||
iansart | 0 | 1,057,105,778 | 0.6% | ||
mobbs | 0 | 24,194,282,198 | 7.5% | ||
eliel | 0 | 2,561,760,278 | 1.2% | ||
jerrybanfield | 0 | 3,462,014,603 | 1.2% | ||
rt395 | 0 | 2,032,992,936 | 1.5% | ||
bitrocker2020 | 0 | 1,819,233,437 | 0.18% | ||
arunava | 0 | 4,044,079,137 | 0.48% | ||
samminator | 0 | 9,452,983,391 | 7.5% | ||
enjar | 0 | 9,221,292,341 | 1.08% | ||
lorenzor | 0 | 1,342,783,326 | 50% | ||
alexander.alexis | 0 | 9,058,366,718 | 15% | ||
professorbromide | 0 | 113,968,595,835 | 100% | ||
dandesign86 | 0 | 16,039,924,477 | 8% | ||
jayna | 0 | 1,642,742,914 | 0.24% | ||
princessmewmew | 0 | 1,603,644,653 | 0.6% | ||
joeyarnoldvn | 0 | 474,600,408 | 1.47% | ||
gunthertopp | 0 | 15,928,453,873 | 0.3% | ||
empath | 0 | 845,735,939 | 0.6% | ||
simonpeter35 | 0 | 1,268,614,219 | 100% | ||
minnowbooster | 0 | 1,071,801,350,195 | 20% | ||
felt.buzz | 0 | 2,167,682,129 | 0.3% | ||
howo | 0 | 480,921,614,150 | 15% | ||
tsoldovieri | 0 | 1,538,444,654 | 7.5% | ||
steemwizards | 0 | 842,516,314 | 1.2% | ||
neumannsalva | 0 | 906,922,229 | 0.6% | ||
stayoutoftherz | 0 | 33,131,548,467 | 0.3% | ||
abigail-dantes | 0 | 5,449,124,201 | 15% | ||
coindevil | 0 | 534,477,939 | 0.96% | ||
cranium | 0 | 592,490,450 | 25% | ||
zonguin | 0 | 764,897,174 | 3.75% | ||
investingpennies | 0 | 4,353,653,161 | 1.2% | ||
khalil319 | 0 | 1,978,258,681 | 10% | ||
val.halla | 0 | 2,869,753,262 | 10% | ||
iamphysical | 0 | 804,295,341 | 90% | ||
zyx066 | 0 | 855,680,347 | 0.36% | ||
azulear | 0 | 1,373,149,887 | 100% | ||
psicoluigi | 0 | 850,151,467 | 50% | ||
thelordsharvest | 0 | 1,003,432,282 | 1.2% | ||
sumant | 0 | 571,186,062 | 0.6% | ||
splash-of-angs63 | 0 | 7,659,018,368 | 60% | ||
meno | 0 | 5,408,428,020 | 0.6% | ||
buttcoins | 0 | 1,796,047,487 | 0.24% | ||
doifeellucky | 0 | 1,067,513,977 | 0.6% | ||
enzor | 0 | 821,606,949 | 15% | ||
dandays | 0 | 3,517,531,476 | 0.22% | ||
notb4mycoffee | 0 | 639,140,324 | 1.2% | ||
anadolu | 0 | 142,259,645,480 | 25% | ||
sunsea | 0 | 485,185,094 | 0.6% | ||
bluefinstudios | 0 | 743,215,670 | 0.36% | ||
steveconnor | 0 | 971,257,364 | 0.6% | ||
dbddv01 | 0 | 509,883,886 | 3.75% | ||
nicole-st | 0 | 549,711,862 | 0.6% | ||
aboutcoolscience | 0 | 3,190,555,688 | 15% | ||
sandracarrascal | 0 | 486,583,165 | 50% | ||
turkiye | 0 | 882,232,707 | 25% | ||
kenadis | 0 | 3,833,468,828 | 15% | ||
madridbg | 0 | 6,983,197,655 | 15% | ||
punchline | 0 | 1,402,731,573 | 1.2% | ||
ennyta | 0 | 974,228,411 | 50% | ||
juecoree | 0 | 1,974,955,075 | 10.5% | ||
carn | 0 | 730,015,218 | 1.08% | ||
branbello | 0 | 699,730,645 | 7.5% | ||
hetty-rowan | 0 | 591,954,098 | 0.6% | ||
ydavgonzalez | 0 | 2,226,694,549 | 10% | ||
intrepidphotos | 0 | 3,617,577,230 | 11.25% | ||
fineartnow | 0 | 803,886,295 | 0.6% | ||
fragmentarion | 0 | 8,541,279,166 | 15% | ||
utube | 0 | 939,281,937 | 1.2% | ||
coinmeria | 0 | 726,075,173 | 35% | ||
dynamicrypto | 0 | 3,222,530,139 | 1% | ||
neneandy | 0 | 1,341,906,925 | 1.2% | ||
marc-allaria | 0 | 887,144,910 | 0.6% | ||
sportscontest | 0 | 1,243,006,284 | 1.2% | ||
gribouille | 0 | 670,541,806 | 15% | ||
pandasquad | 0 | 3,057,361,243 | 1.2% | ||
miguelangel2801 | 0 | 788,442,754 | 50% | ||
emiliomoron | 0 | 2,235,966,077 | 7.5% | ||
dexterdev | 0 | 575,471,222 | 7.5% | ||
photohunt | 0 | 802,670,592 | 1.2% | ||
geopolis | 0 | 951,275,585 | 15% | ||
robertbira | 0 | 1,580,492,793 | 3.75% | ||
alexdory | 0 | 1,710,630,715 | 15% | ||
irgendwo | 0 | 4,616,379,091 | 1.2% | ||
flugschwein | 0 | 1,798,352,706 | 12.75% | ||
charitybot | 0 | 5,033,664,285 | 100% | ||
francostem | 0 | 2,023,670,274 | 15% | ||
endopediatria | 0 | 692,859,180 | 20% | ||
croctopus | 0 | 1,445,530,955 | 100% | ||
putu300 | 0 | 549,162,171 | 5% | ||
zipporah | 0 | 550,877,764 | 0.24% | ||
superlotto | 0 | 3,284,755,670 | 1.2% | ||
movingman | 0 | 516,801,517 | 20% | ||
tomastonyperez | 0 | 16,947,703,171 | 50% | ||
bil.prag | 0 | 501,907,444 | 0.06% | ||
elvigia | 0 | 11,052,341,002 | 50% | ||
qberry | 0 | 790,957,581 | 0.6% | ||
greddyforce | 0 | 827,521,607 | 0.44% | ||
fotogruppemunich | 0 | 842,940,147 | 0.3% | ||
therising | 0 | 20,141,081,202 | 1.2% | ||
de-stem | 0 | 8,139,643,604 | 14.85% | ||
serylt | 0 | 645,328,148 | 14.7% | ||
josedelacruz | 0 | 4,835,653,243 | 50% | ||
charitymemes | 0 | 522,832,419 | 100% | ||
erickyoussif | 0 | 615,080,140 | 100% | ||
deholt | 0 | 828,662,425 | 12.75% | ||
minerthreat | 0 | 698,662,410 | 0.6% | ||
temitayo-pelumi | 0 | 1,335,626,143 | 15% | ||
andrick | 0 | 857,252,466 | 50% | ||
uche-nna | 0 | 943,280,168 | 0.96% | ||
yougotavote | 0 | 3,429,957,621 | 100% | ||
cheese4ead | 0 | 928,250,311 | 0.6% | ||
mafufuma | 0 | 7,407,574,329 | 1% | ||
apshamilton | 0 | 2,641,108,011 | 0.15% | ||
gaottantacinque | 0 | 516,626,243 | 100% | ||
nattybongo | 0 | 28,533,585,259 | 15% | ||
bflanagin | 0 | 1,019,757,164 | 0.6% | ||
ubaldonet | 0 | 2,432,974,256 | 80% | ||
armandosodano | 0 | 676,463,340 | 0.6% | ||
hamismsf | 0 | 820,393,752 | 0.15% | ||
goblinknackers | 0 | 74,055,088,281 | 7% | ||
reinaseq | 0 | 7,528,718,258 | 100% | ||
gasaeightyfive | 0 | 898,032,423 | 100% | ||
ozeryilmaz | 0 | 496,252,121 | 50% | ||
fran.frey | 0 | 4,171,980,642 | 50% | ||
bagasadi | 0 | 2,461,054,269 | 100% | ||
thelittlebank | 0 | 4,149,147,793 | 0.6% | ||
pboulet | 0 | 27,817,845,397 | 12% | ||
stem-espanol | 0 | 13,234,977,746 | 100% | ||
cribbio | 0 | 2,175,081,703 | 100% | ||
aleestra | 0 | 12,933,003,292 | 80% | ||
palasatenea | 0 | 668,497,743 | 0.6% | ||
brianoflondon | 0 | 15,027,892,056 | 0.3% | ||
giulyfarci52 | 0 | 1,705,280,816 | 50% | ||
steemcryptosicko | 0 | 1,920,666,391 | 0.24% | ||
cakemonster | 0 | 685,854,704 | 1.2% | ||
stem.witness | 0 | 853,318,981 | 15% | ||
jpbliberty | 0 | 1,353,508,638 | 0.3% | ||
double-negative | 0 | 521,138,088 | 20% | ||
steemstorage | 0 | 1,460,994,463 | 1.2% | ||
crowdwitness | 0 | 40,034,217,191 | 7.5% | ||
hairgistix | 0 | 641,318,488 | 0.6% | ||
steemean | 0 | 9,845,046,116 | 5% | ||
tinyhousecryptos | 0 | 478,621,400 | 5% | ||
zeruxanime | 0 | 2,254,228,146 | 50% | ||
reggaesteem | 0 | 476,720,485 | 5% | ||
stemgeeks | 0 | 1,150,237,854 | 50% | ||
babytarazkp | 0 | 1,331,745,209 | 40% | ||
nazer | 0 | 548,517,325 | 7.5% | ||
stem.alfa | 0 | 3,945,328,725 | 100% | ||
steemstem-trig | 0 | 270,274,770 | 15% | ||
baltai | 0 | 1,165,544,929 | 0.6% | ||
yggdrasil.laguna | 0 | 0 | 25% | ||
ibt-survival | 0 | 36,313,820,114 | 10% | ||
xerxes.alpha | 0 | 3,256,344,327 | 100% | ||
curacer | 0 | 3,471,737,159 | 50% | ||
zirky | 0 | 534,565,899 | 1.02% | ||
lightpaintershub | 0 | 661,192,261 | 1% | ||
yunnie | 0 | 751,470,280 | 100% | ||
stemsocial | 0 | 123,302,061,537 | 15% | ||
noelyss | 0 | 4,824,722,048 | 7.5% | ||
quinnertronics | 0 | 14,566,386,080 | 7% | ||
aabcent | 0 | 641,993,084 | 0.96% | ||
altleft | 0 | 4,582,536,681 | 0.01% | ||
dhedge | 0 | 761,703,946,017 | 7.81% | ||
meritocracy | 0 | 13,320,804,898 | 0.12% | ||
jmsansan | 0 | 953,060,041 | 0.6% | ||
sillybilly | 0 | 528,412,616 | 100% | ||
krishu.stem | 0 | 349,281,900 | 100% | ||
endrius | 0 | 2,885,124,076 | 75% | ||
adamada.stem | 0 | 4,981,335,426 | 100% | ||
failingforwards | 0 | 585,352,882 | 0.6% | ||
imno | 0 | 9,792,079,610 | 10% | ||
nfttunz | 0 | 1,754,780,974 | 0.12% | ||
okluvmee | 0 | 541,369,925 | 0.6% | ||
holovision.cash | 0 | 1,773,126,105 | 100% | ||
holovision.stem | 0 | 285,223,525 | 100% | ||
podping | 0 | 1,630,171,712 | 0.3% | ||
pinkfloyd878 | 0 | 4,195,321,202 | 100% | ||
duncanek | 0 | 641,806,810 | 25% | ||
solominer.stem | 0 | 817,049,485 | 100% | ||
laviesm | 0 | 5,766,994,776 | 50% | ||
untzuntzuntz | 0 | 5,287,995,936 | 25% | ||
aries90 | 0 | 8,299,313,558 | 1.2% | ||
uknowm3 | 0 | 1,145,123,183 | 100% | ||
kingz1337 | 0 | 1,318,755,994 | 100% | ||
centtoken | 0 | 201,396,045,927 | 25% | ||
kingz1338 | 0 | 753,470,743 | 100% | ||
yixn | 0 | 8,003,739,398 | 0.6% | ||
kqaosphreak | 0 | 970,801,775 | 15% | ||
waivio.curator | 0 | 1,603,096,270 | 3% | ||
taradraz1 | 0 | 2,837,491,318 | 100% | ||
vindiesel1980 | 0 | 583,858,269 | 0.6% | ||
zerozerozero | 0 | 67,202,654,554 | 100% | ||
zerozeroone | 0 | 19,039,541,892 | 100% | ||
benwickenton | 0 | 533,800,829 | 1.2% | ||
archangel21 | 0 | 601,738,914 | 1.2% | ||
embunpagi | 0 | 487,911,787 | 25% | ||
soyjoselopez | 0 | 474,858,129 | 20% | ||
sbtofficial | 0 | 964,805,303 | 0.6% | ||
inibless | 0 | 481,185,731 | 7.5% | ||
clpacksperiment | 0 | 606,295,207 | 0.6% | ||
humbe | 0 | 1,255,700,113 | 1% | ||
opticus | 0 | 1,014,525,109 | 0.6% | ||
rhemagames | 0 | 1,054,028,992 | 0.6% | ||
fredgsanford | 0 | 26,636,329 | 100% | ||
danigada18 | 0 | 6,161,571,236 | 100% |
Tossing virtual coins into the gaming pot is a pirate’s plunder and you’re the swashbuckler after the loot! Sail toward baccarat with a slick 1.06% edge for smooth sailing. Don’t spill your treasure chest, wager 2% per round to keep the ship steady. Bonuses are your wind in the sails, some spots hoist a 180% lift, turning $100 to $280 quicker than a cannon blast. For a trusty captain’s log, https://casinosmitschnellerauszahlung.com charts the course with ace gaming wisdom. Set sail when your compass is true, not after a grog-soaked night. Withdrawals dock in under 45 minutes, fast as a sea breeze. Seek provably fair booty for an honest haul and pile those digital doubloons like a pirate’s secret cove. Steer sharp, grin at the horizon, and claim a treasure trove that’d make Blackbeard jealous! Posted using [STEMGeeks](https://stemgeeks.net/@carteritos/re-skyehi-mau2pngr)
author | carteritos |
---|---|
permlink | re-skyehi-mau2pngr |
category | hive-196387 |
json_metadata | {"tags":["stem"],"format":"markdown","canonical_url":"https://stemgeeks.net/@carteritos/re-skyehi-mau2pngr","app":"stemgeeks/1.2.0"} |
created | 2025-05-18 19:52:57 |
last_update | 2025-05-18 19:52:57 |
depth | 1 |
children | 0 |
last_payout | 2025-05-25 19:52:57 |
cashout_time | 1969-12-31 23:59:59 |
total_payout_value | 0.000 HBD |
curator_payout_value | 0.000 HBD |
pending_payout_value | 0.000 HBD |
promoted | 0.000 HBD |
body_length | 886 |
author_reputation | 155,663,696,394 |
root_title | "How to build a Dice Roller App for Android: Our First Game - Hive Programmers" |
beneficiaries | [] |
max_accepted_payout | 1,000,000.000 HBD |
percent_hbd | 10,000 |
post_id | 142,813,708 |
net_rshares | 0 |
<div class='text-justify'> <div class='pull-left'> <img src='https://stem.openhive.network/images/stemsocialsupport7.png'> </div> Thanks for your contribution to the <a href='/trending/hive-196387'>STEMsocial community</a>. Feel free to join us on <a href='https://discord.gg/9c7pKVD'>discord</a> to get to know the rest of us! Please consider delegating to the @stemsocial account (85% of the curation rewards are returned). Thanks for including @stemsocial as a beneficiary, which gives you stronger support. <br /> <br /> </div>
author | stemsocial |
---|---|
permlink | re-skyehi-how-to-build-a-dice-roller-app-for-android-our-first-game-hive-programmers-20231130t231953268z |
category | hive-196387 |
json_metadata | {"app":"STEMsocial"} |
created | 2023-11-30 23:19:54 |
last_update | 2023-11-30 23:19:54 |
depth | 1 |
children | 0 |
last_payout | 2023-12-07 23:19:54 |
cashout_time | 1969-12-31 23:59:59 |
total_payout_value | 0.000 HBD |
curator_payout_value | 0.000 HBD |
pending_payout_value | 0.000 HBD |
promoted | 0.000 HBD |
body_length | 545 |
author_reputation | 22,915,413,852,146 |
root_title | "How to build a Dice Roller App for Android: Our First Game - Hive Programmers" |
beneficiaries | [] |
max_accepted_payout | 1,000,000.000 HBD |
percent_hbd | 10,000 |
post_id | 129,307,138 |
net_rshares | 0 |